


Object-Oriented Programming in C? Implementing an Interface from Scratch
Exploring the intricacies of computing often involves understanding not just how something works, but also why and how it could be built from scratch. This article delves into the concept of interfaces in object-oriented programming (OOP), using Java as a reference point, and then demonstrates a rudimentary C implementation.
A Simple Vehicle Pricing Example
Our example focuses on calculating vehicle prices: cars priced by speed, motorcycles by engine displacement (cc). We begin with a Java interface defining the core vehicle behavior:
public interface Vehicle { Integer price(); }
This interface is then implemented by Car
and Motorcycle
classes:
public class Car implements Vehicle { private final Integer speed; public Car(Integer speed) { this.speed = speed; } @Override public Integer price() { return speed * 60; } } public class Motorcycle implements Vehicle { private final Integer cc; public Motorcycle(Integer cc) { this.cc = cc; } @Override public Integer price() { return cc * 10; } }
A helper function prints the price:
public static void printVehiclePrice(Vehicle vehicle) { System.out.println("$" + vehicle.price() + ".00"); }
The main method demonstrates usage:
public static void main(String[] args) { Car car = new Car(120); Motorcycle motorcycle = new Motorcycle(1000); printVehiclePrice(car); // Output: 00.00 printVehiclePrice(motorcycle); // Output: 000.00 }
Replicating this in C requires a different approach.
Implementing Interfaces in C: A Manual Approach
In C, we lack the built-in interface mechanism of Java. We'll simulate it using structs for data and functions for methods. The compiler doesn't handle interface resolution; we must do it manually.
Our "interface" skeleton:
#include <stdio.h> #include <stdlib.h> typedef enum { VEHICLE_CAR, VEHICLE_MOTORCYCLE } VehicleType; typedef struct { VehicleType type; } Vehicle; void vehicle_free(Vehicle *vehicle); int vehicle_price(Vehicle *vehicle);
The Car
implementation:
typedef struct { VehicleType type; int speed; } Car; Car *car_init(int speed) { Car *car = malloc(sizeof(Car)); car->type = VEHICLE_CAR; car->speed = speed; return car; } void car_free(Car *car) { free(car); } int car_price(Car *car) { return car->speed * 60; }
The Motorcycle
implementation (similar to Car
):
typedef struct { VehicleType type; int cc; } Motorcycle; Motorcycle *motorcycle_init(int cc) { Motorcycle *motorcycle = malloc(sizeof(Motorcycle)); motorcycle->type = VEHICLE_MOTORCYCLE; motorcycle->cc = cc; return motorcycle; } void motorcycle_free(Motorcycle *motorcycle) { free(motorcycle); } int motorcycle_price(Motorcycle *motorcycle) { return motorcycle->cc * 10; }
The price printing function:
void print_vehicle_price(Vehicle *vehicle) { printf("$%d.00\n", vehicle_price(vehicle)); }
Crucially, we implement vehicle_free
and vehicle_price
using switch
statements to handle different vehicle types:
void vehicle_free(Vehicle *vehicle) { switch (vehicle->type) { case VEHICLE_CAR: car_free((Car *)vehicle); break; case VEHICLE_MOTORCYCLE: motorcycle_free((Motorcycle *)vehicle); break; } } int vehicle_price(Vehicle *vehicle) { switch (vehicle->type) { case VEHICLE_CAR: return car_price((Car *)vehicle); case VEHICLE_MOTORCYCLE: return motorcycle_price((Motorcycle *)vehicle); } }
The main function demonstrates usage:
int main(void) { Car *car = car_init(120); Motorcycle *motorcycle = motorcycle_init(1000); print_vehicle_price((Vehicle *)car); // Output: 00.00 print_vehicle_price((Vehicle *)motorcycle); // Output: 000.00 vehicle_free((Vehicle *)car); vehicle_free((Vehicle *)motorcycle); return 0; }
A Practical Application: Abstract Syntax Trees (ASTs)
This manual interface approach is particularly useful in scenarios like parsing, where an Abstract Syntax Tree (AST) might benefit from a similar structure. Different node types in the AST can be represented as separate structs, all conforming to a common "interface" defined by a set of functions.
Conclusion
While C lacks built-in interfaces, simulating them with careful struct and function design provides a powerful mechanism for achieving similar OOP principles. This manual approach offers flexibility and control, particularly beneficial in complex projects like parsers and interpreters.
The above is the detailed content of Object-Oriented Programming in C? Implementing an Interface from Scratch. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










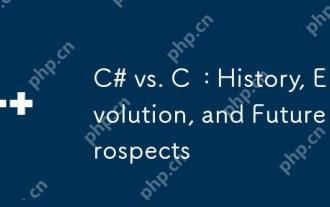
The history and evolution of C# and C are unique, and the future prospects are also different. 1.C was invented by BjarneStroustrup in 1983 to introduce object-oriented programming into the C language. Its evolution process includes multiple standardizations, such as C 11 introducing auto keywords and lambda expressions, C 20 introducing concepts and coroutines, and will focus on performance and system-level programming in the future. 2.C# was released by Microsoft in 2000. Combining the advantages of C and Java, its evolution focuses on simplicity and productivity. For example, C#2.0 introduced generics and C#5.0 introduced asynchronous programming, which will focus on developers' productivity and cloud computing in the future.
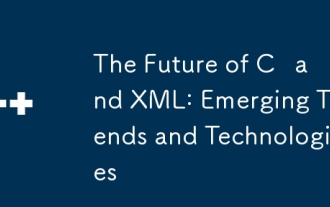
The future development trends of C and XML are: 1) C will introduce new features such as modules, concepts and coroutines through the C 20 and C 23 standards to improve programming efficiency and security; 2) XML will continue to occupy an important position in data exchange and configuration files, but will face the challenges of JSON and YAML, and will develop in a more concise and easy-to-parse direction, such as the improvements of XMLSchema1.1 and XPath3.1.
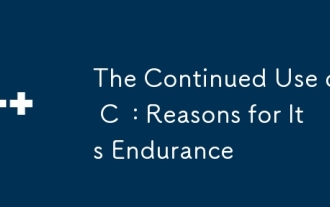
C Reasons for continuous use include its high performance, wide application and evolving characteristics. 1) High-efficiency performance: C performs excellently in system programming and high-performance computing by directly manipulating memory and hardware. 2) Widely used: shine in the fields of game development, embedded systems, etc. 3) Continuous evolution: Since its release in 1983, C has continued to add new features to maintain its competitiveness.
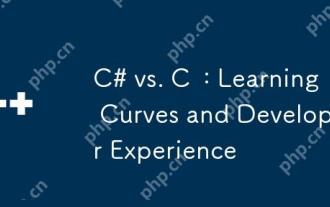
There are significant differences in the learning curves of C# and C and developer experience. 1) The learning curve of C# is relatively flat and is suitable for rapid development and enterprise-level applications. 2) The learning curve of C is steep and is suitable for high-performance and low-level control scenarios.
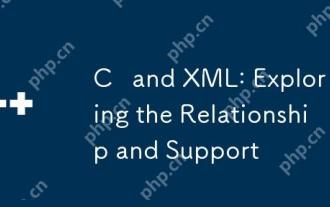
C interacts with XML through third-party libraries (such as TinyXML, Pugixml, Xerces-C). 1) Use the library to parse XML files and convert them into C-processable data structures. 2) When generating XML, convert the C data structure to XML format. 3) In practical applications, XML is often used for configuration files and data exchange to improve development efficiency.
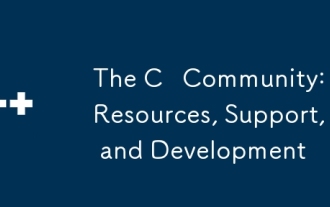
C Learners and developers can get resources and support from StackOverflow, Reddit's r/cpp community, Coursera and edX courses, open source projects on GitHub, professional consulting services, and CppCon. 1. StackOverflow provides answers to technical questions; 2. Reddit's r/cpp community shares the latest news; 3. Coursera and edX provide formal C courses; 4. Open source projects on GitHub such as LLVM and Boost improve skills; 5. Professional consulting services such as JetBrains and Perforce provide technical support; 6. CppCon and other conferences help careers
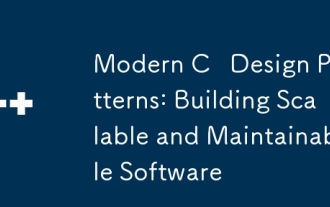
The modern C design model uses new features of C 11 and beyond to help build more flexible and efficient software. 1) Use lambda expressions and std::function to simplify observer pattern. 2) Optimize performance through mobile semantics and perfect forwarding. 3) Intelligent pointers ensure type safety and resource management.
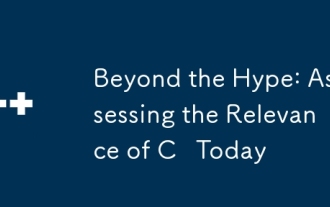
C still has important relevance in modern programming. 1) High performance and direct hardware operation capabilities make it the first choice in the fields of game development, embedded systems and high-performance computing. 2) Rich programming paradigms and modern features such as smart pointers and template programming enhance its flexibility and efficiency. Although the learning curve is steep, its powerful capabilities make it still important in today's programming ecosystem.
