


How Do I Query Complex Data Types (Arrays, Maps, Structs) in Spark SQL DataFrames?
Jan 21, 2025 am 11:22 AMAccessing Complex Data in Spark SQL DataFrames
Spark SQL supports complex data types like arrays and maps. However, querying these requires specific approaches. This guide details how to effectively query these structures:
Arrays:
Several methods exist for accessing array elements:
-
getItem
method: This DataFrame API method directly accesses elements by index.df.select($"an_array".getItem(1)).show
Copy after login -
Hive bracket syntax: This SQL-like syntax offers an alternative.
SELECT an_array[1] FROM df
Copy after login -
User-Defined Functions (UDFs): UDFs provide flexibility for more complex array manipulations.
val get_ith = udf((xs: Seq[Int], i: Int) => Try(xs(i)).toOption) df.select(get_ith($"an_array", lit(1))).show
Copy after login -
Built-in functions: Spark offers built-in functions like
transform
,filter
,aggregate
, and thearray_*
family for array processing.
Maps:
Accessing map values involves similar techniques:
-
getField
method: Retrieves values using the key.df.select($"a_map".getField("foo")).show
Copy after login -
Hive bracket syntax: Provides a SQL-like approach.
SELECT a_map['foo'] FROM df
Copy after login -
Dot syntax: A concise way to access map fields.
df.select($"a_map.foo").show
Copy after login -
UDFs: For customized map operations.
val get_field = udf((kvs: Map[String, String], k: String) => kvs.get(k)) df.select(get_field($"a_map", lit("foo"))).show
Copy after login -
*`map_
functions:** Functions like
map_keysand
map_values` are available for map manipulation.
Structs:
Accessing struct fields is straightforward:
-
Dot syntax: The most direct method.
df.select($"a_struct.x").show
Copy after login -
Raw SQL: An alternative using SQL syntax.
SELECT a_struct.x FROM df
Copy after login
Arrays of Structs:
Querying nested structures requires combining the above techniques:
-
Nested dot syntax: Access fields within structs within arrays.
df.select($"an_array_of_structs.foo").show
Copy after login -
Combined methods: Using
getItem
to access array elements and then dot syntax for struct fields.df.select($"an_array_of_structs.vals".getItem(1).getItem(1)).show
Copy after login
User-Defined Types (UDTs):
UDTs are typically accessed using UDFs.
Important Considerations:
-
Context: Some methods might only work with
HiveContext
, depending on your Spark version. - Nested Field Support: Not all operations support deeply nested fields.
- Efficiency: Schema flattening or collection explosion might improve performance for complex queries.
-
Wildcard: The wildcard character (
*
) can be used with dot syntax to select multiple fields.
This guide provides a comprehensive overview of querying complex data types in Spark SQL DataFrames. Remember to choose the method best suited for your specific needs and data structure.
The above is the detailed content of How Do I Query Complex Data Types (Arrays, Maps, Structs) in Spark SQL DataFrames?. For more information, please follow other related articles on the PHP Chinese website!

Hot Article

Hot tools Tags

Hot Article

Hot Article Tags

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
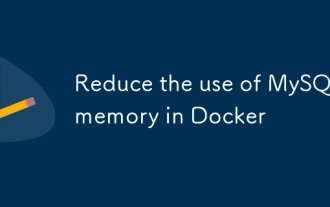
Reduce the use of MySQL memory in Docker
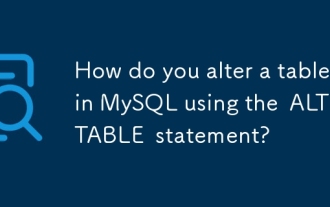
How do you alter a table in MySQL using the ALTER TABLE statement?
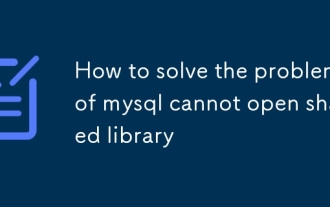
How to solve the problem of mysql cannot open shared library
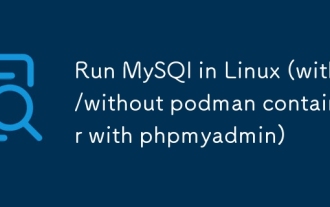
Run MySQl in Linux (with/without podman container with phpmyadmin)
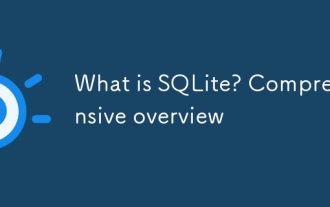
What is SQLite? Comprehensive overview
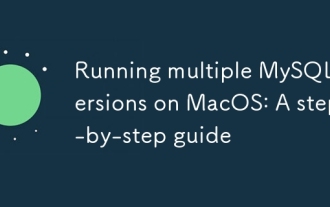
Running multiple MySQL versions on MacOS: A step-by-step guide
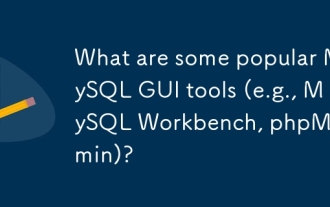
What are some popular MySQL GUI tools (e.g., MySQL Workbench, phpMyAdmin)?
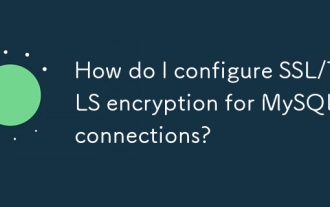
How do I configure SSL/TLS encryption for MySQL connections?
