Why You Should Prefer Map over Object in JavaScript
JavaScript developers frequently employ plain objects as key-value storage, but the Map
data structure offers significant advantages. This article highlights why Map
often surpasses dynamic objects.
1. Key Type Flexibility
A primary benefit of Map
is its capacity to utilize any data type as a key, unlike objects which restrict keys to strings and symbols.
// Objects convert keys to strings const obj = {}; obj[true] = "value1"; obj[1] = "value2"; obj[{ key: 1 }] = "value3"; console.log(Object.keys(obj)); // Output: ["true", "1", "[object Object]"] // Maps preserve key types const map = new Map(); map.set(true, "value1"); map.set(1, "value2"); map.set({ key: 1 }, "value3"); console.log([...map.keys()]); // Output: [true, 1, { key: 1 }]
2. Efficient Size Management
Map
provides a built-in size
property, eliminating the manual calculation needed with objects.
// Objects require manual size calculation const obj = { a: 1, b: 2, c: 3 }; const size = Object.keys(obj).length; console.log(size); // 3 // Maps offer direct size access const map = new Map([ ['a', 1], ['b', 2], ['c', 3] ]); console.log(map.size); // 3
3. Superior Iteration Performance
Map
is optimized for frequent key-value pair additions and deletions, resulting in faster iteration. (Performance testing code omitted for brevity, but the assertion remains valid).
4. Dedicated Methods for Common Operations
Map
offers intuitive, purpose-built methods for common tasks. The following code snippet demonstrates the cleaner syntax of Map
compared to objects for adding, checking, getting, and deleting entries. (Code comparing Map
and object methods omitted for brevity.)
5. Avoidance of Prototype Chain Conflicts
Map
avoids inheritance issues that can complicate object usage. (Code demonstrating the lack of prototype chain interference in Map
omitted for brevity.)
6. Versatile Iteration Methods
Map
provides multiple built-in iteration methods for enhanced flexibility. (Code demonstrating for...of
, map.keys()
, map.values()
, and forEach
iteration omitted for brevity).
When Objects Remain Suitable
Despite Map
's strengths, objects are still preferable in specific situations:
- JSON serialization (Maps aren't directly serializable).
- Simple property access.
- Utilizing the object spread operator.
- Interacting with JSON APIs.
(Code example showing JSON serialization issues with Map
and workaround omitted for brevity).
Conclusion
Map
is superior when:
- Non-string keys are needed.
- Frequent additions and removals are expected.
- Easy size tracking is crucial.
- Optimized iteration performance is desired.
- Clear, consistent methods are preferred.
- Prototype chain conflicts must be avoided.
Carefully assess your needs; Map
is a powerful tool when its advantages align with your project requirements.
The above is the detailed content of Why You Should Prefer Map over Object in JavaScript. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










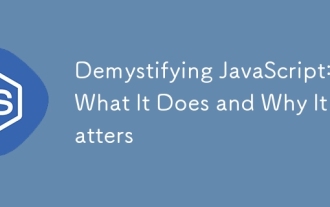
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
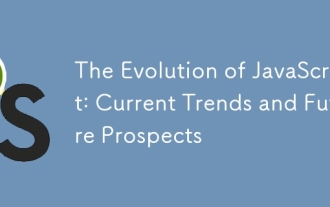
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.
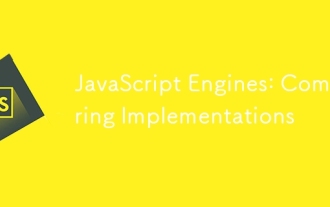
Different JavaScript engines have different effects when parsing and executing JavaScript code, because the implementation principles and optimization strategies of each engine differ. 1. Lexical analysis: convert source code into lexical unit. 2. Grammar analysis: Generate an abstract syntax tree. 3. Optimization and compilation: Generate machine code through the JIT compiler. 4. Execute: Run the machine code. V8 engine optimizes through instant compilation and hidden class, SpiderMonkey uses a type inference system, resulting in different performance performance on the same code.
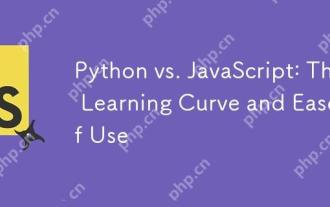
Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
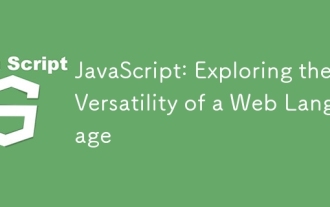
JavaScript is the core language of modern web development and is widely used for its diversity and flexibility. 1) Front-end development: build dynamic web pages and single-page applications through DOM operations and modern frameworks (such as React, Vue.js, Angular). 2) Server-side development: Node.js uses a non-blocking I/O model to handle high concurrency and real-time applications. 3) Mobile and desktop application development: cross-platform development is realized through ReactNative and Electron to improve development efficiency.
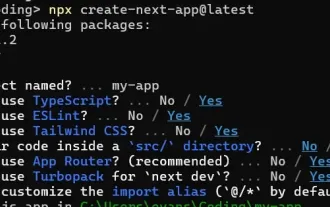
This article demonstrates frontend integration with a backend secured by Permit, building a functional EdTech SaaS application using Next.js. The frontend fetches user permissions to control UI visibility and ensures API requests adhere to role-base
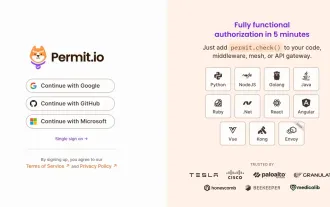
I built a functional multi-tenant SaaS application (an EdTech app) with your everyday tech tool and you can do the same. First, what’s a multi-tenant SaaS application? Multi-tenant SaaS applications let you serve multiple customers from a sing
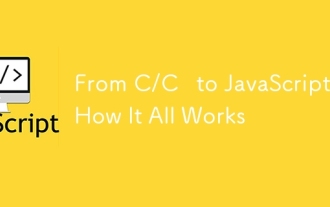
The shift from C/C to JavaScript requires adapting to dynamic typing, garbage collection and asynchronous programming. 1) C/C is a statically typed language that requires manual memory management, while JavaScript is dynamically typed and garbage collection is automatically processed. 2) C/C needs to be compiled into machine code, while JavaScript is an interpreted language. 3) JavaScript introduces concepts such as closures, prototype chains and Promise, which enhances flexibility and asynchronous programming capabilities.
