How to Implement Simple PHP Pagination for Database Results?
Simple PHP database paging script
When dealing with large amounts of data, paging functionality is crucial to effectively organize and present information. Here is an easy-to-understand PHP pagination script to help you accomplish this task.
Core concept:
The script determines the total number of items in the database and then calculates the number of pages required to display all items based on predefined limits. It calculates the offset of the current page and displays information about the current page, such as the starting and ending line numbers.
Example implementation:
The following code example demonstrates PHP pagination scripts in action:
<?php // 数据库连接和查询 $dbh = new PDO(...); $total = $dbh->query('SELECT COUNT(*) FROM table')->fetchColumn(); // 分页设置 $limit = 20; $pages = ceil($total / $limit); $page = min($pages, filter_input(INPUT_GET, 'page', FILTER_VALIDATE_INT, array( 'options' => array( 'default' => 1, 'min_range' => 1, ), ))); $offset = ($page - 1) * $limit; // 分页显示 $start = $offset + 1; $end = min(($offset + $limit), $total); $prevlink = ($page > 1) ? '<a href="?page=1">首页</a> <a href="?page=' . ($page - 1) . '">上一页</a>' : '« ‹'; $nextlink = ($page < $pages) ? '<a href="?page=' . ($page + 1) . '">下一页</a> <a href="?page=' . $pages . '">尾页</a>' : '› »'; echo "<div><p>此脚本根据数据库结果动态计算分页,提供了一种用户友好的方式,可以一次一页地浏览大型数据集。</p></div>"; echo "<div>显示{$start}到{$end}条记录,共{$total}条记录,共{$pages}页</div>"; echo "<div>{$prevlink} {$nextlink}</div>"; // 查询并显示分页数据 $stmt = $dbh->prepare("SELECT * FROM table LIMIT :limit OFFSET :offset"); $stmt->bindParam(':limit', $limit, PDO::PARAM_INT); $stmt->bindParam(':offset', $offset, PDO::PARAM_INT); $stmt->execute(); while ($row = $stmt->fetch(PDO::FETCH_ASSOC)) { // 显示数据 echo "<pre class="brush:php;toolbar:false">"; print_r($row); echo ""; } ?>
The above is the detailed content of How to Implement Simple PHP Pagination for Database Results?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










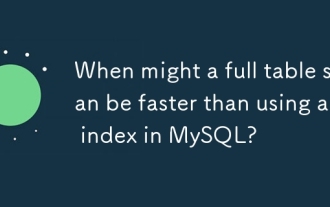
Full table scanning may be faster in MySQL than using indexes. Specific cases include: 1) the data volume is small; 2) when the query returns a large amount of data; 3) when the index column is not highly selective; 4) when the complex query. By analyzing query plans, optimizing indexes, avoiding over-index and regularly maintaining tables, you can make the best choices in practical applications.
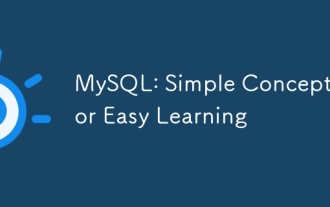
MySQL is an open source relational database management system. 1) Create database and tables: Use the CREATEDATABASE and CREATETABLE commands. 2) Basic operations: INSERT, UPDATE, DELETE and SELECT. 3) Advanced operations: JOIN, subquery and transaction processing. 4) Debugging skills: Check syntax, data type and permissions. 5) Optimization suggestions: Use indexes, avoid SELECT* and use transactions.
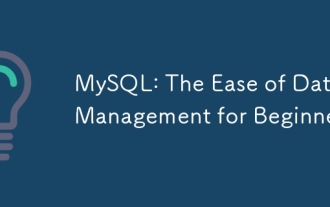
MySQL is suitable for beginners because it is simple to install, powerful and easy to manage data. 1. Simple installation and configuration, suitable for a variety of operating systems. 2. Support basic operations such as creating databases and tables, inserting, querying, updating and deleting data. 3. Provide advanced functions such as JOIN operations and subqueries. 4. Performance can be improved through indexing, query optimization and table partitioning. 5. Support backup, recovery and security measures to ensure data security and consistency.
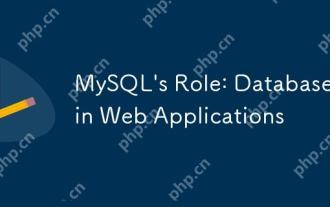
The main role of MySQL in web applications is to store and manage data. 1.MySQL efficiently processes user information, product catalogs, transaction records and other data. 2. Through SQL query, developers can extract information from the database to generate dynamic content. 3.MySQL works based on the client-server model to ensure acceptable query speed.
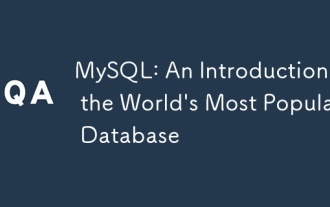
MySQL is an open source relational database management system, mainly used to store and retrieve data quickly and reliably. Its working principle includes client requests, query resolution, execution of queries and return results. Examples of usage include creating tables, inserting and querying data, and advanced features such as JOIN operations. Common errors involve SQL syntax, data types, and permissions, and optimization suggestions include the use of indexes, optimized queries, and partitioning of tables.
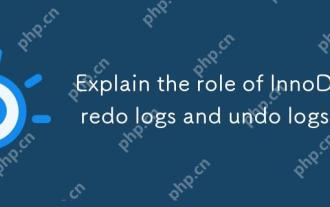
InnoDB uses redologs and undologs to ensure data consistency and reliability. 1.redologs record data page modification to ensure crash recovery and transaction persistence. 2.undologs records the original data value and supports transaction rollback and MVCC.
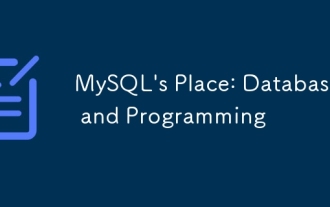
MySQL's position in databases and programming is very important. It is an open source relational database management system that is widely used in various application scenarios. 1) MySQL provides efficient data storage, organization and retrieval functions, supporting Web, mobile and enterprise-level systems. 2) It uses a client-server architecture, supports multiple storage engines and index optimization. 3) Basic usages include creating tables and inserting data, and advanced usages involve multi-table JOINs and complex queries. 4) Frequently asked questions such as SQL syntax errors and performance issues can be debugged through the EXPLAIN command and slow query log. 5) Performance optimization methods include rational use of indexes, optimized query and use of caches. Best practices include using transactions and PreparedStatemen
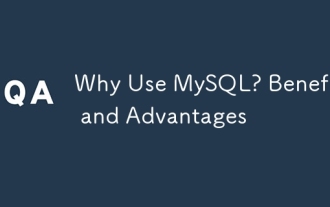
MySQL is chosen for its performance, reliability, ease of use, and community support. 1.MySQL provides efficient data storage and retrieval functions, supporting multiple data types and advanced query operations. 2. Adopt client-server architecture and multiple storage engines to support transaction and query optimization. 3. Easy to use, supports a variety of operating systems and programming languages. 4. Have strong community support and provide rich resources and solutions.
