


How To Structure a Large Flask Application-Best Practices for 5
It is crucial to build a well-structured Flask RESTful API that is readable, maintainable, extensible, and easy for other developers to use. This article will introduce some best practices to help developers improve their API design and provide a complete guide to building a Flask REST API.
Project Structure
A typical and efficient Flask REST API project structure is as follows:
project/ │ ├── app/ │ ├── init.py │ ├── config.py │ ├── models/ │ │ ├── init.py │ │ └── user.py │ ├── routes/ │ │ ├── init.py │ │ └── user_routes.py │ ├── schemas/ │ │ ├── init.py │ │ └── user_schema.py │ ├── services/ │ │ ├── init.py │ │ └── user_service.py │ └── tests/ │ ├── init.py │ └── test_user.py ├── run.py └── requirements.txt
Key components:
- app/init.py: Initialize the Flask application and register the blueprint.
- app/config.py: Contains the application’s configuration settings.
- models/: stores database models.
- routes/: Defines API endpoints.
- schemas/: manages data serialization and validation.
- services/: Contains business logic and interacts with the model.
- tests/: stores the unit tests of the application.
- Using Blueprints: Flask’s blueprint feature allows you to organize your application into different components. Each blueprint can handle its routes, models, and services, making it easier to manage large applications. For example, you can create a user blueprint that specifically handles user-related functionality.
Blueprint initialization example:
# app/routes/user_routes.py from flask import Blueprint user_bp = Blueprint('user', __name__) @user_bp.route('/users', methods=['GET']) def get_users(): # 获取用户逻辑 pass @user_bp.route('/users', methods=['POST']) def create_user(): # 创建新用户逻辑 pass
Implement CRUD operations
Most Flask REST APIs include CRUD operations. Here's how to define these actions in your routes:
CRUD operation example:
# app/routes/user_routes.py @user_bp.route('/users/<user_id>', methods=['GET']) def get_user(user_id): # 根据 ID 获取用户逻辑 pass @user_bp.route('/users/<user_id>', methods=['PUT']) def update_user(user_id): # 更新现有用户逻辑 pass @user_bp.route('/users/<user_id>', methods=['DELETE']) def delete_user(user_id): # 根据 ID 删除用户逻辑 pass
Use Marshmallow for data validation
Data validation and serialization can be greatly simplified using libraries like Marshmallow. Create a schema that represents a data structure:
Pattern definition example:
# app/schemas/user_schema.py from marshmallow import Schema, fields class UserSchema(Schema): id = fields.Int(required=True) username = fields.Str(required=True) email = fields.Email(required=True)
API Test
Testing is critical to ensuring your API works correctly. Unit tests can be written using tools such as pytest.
Test case example:
# app/tests/test_user.py def test_get_users(client): response = client.get('/users') assert response.status_code == 200
Conclusion
You can follow this structured approach to develop a robust and easy-to-maintain Flask REST API. Using blueprints, efficient CRUD operations, data validation through schemas, and documentation with Swagger are best practices that can help you jumpstart your development efforts.
The above is the detailed content of How To Structure a Large Flask Application-Best Practices for 5. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


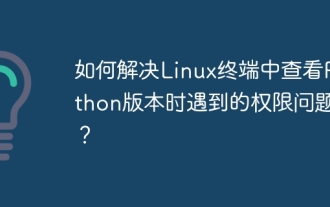
Solution to permission issues when viewing Python version in Linux terminal When you try to view Python version in Linux terminal, enter python...
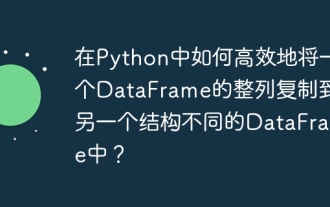
When using Python's pandas library, how to copy whole columns between two DataFrames with different structures is a common problem. Suppose we have two Dats...
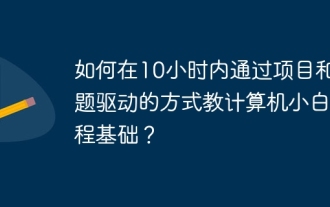
How to teach computer novice programming basics within 10 hours? If you only have 10 hours to teach computer novice some programming knowledge, what would you choose to teach...
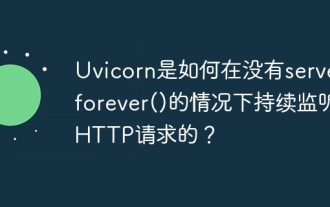
How does Uvicorn continuously listen for HTTP requests? Uvicorn is a lightweight web server based on ASGI. One of its core functions is to listen for HTTP requests and proceed...
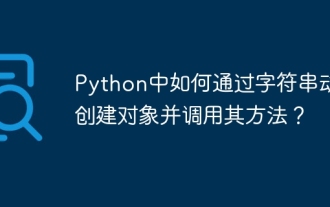
In Python, how to dynamically create an object through a string and call its methods? This is a common programming requirement, especially if it needs to be configured or run...
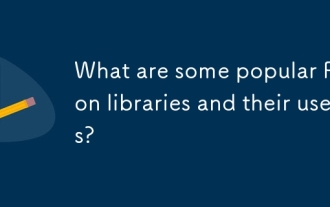
The article discusses popular Python libraries like NumPy, Pandas, Matplotlib, Scikit-learn, TensorFlow, Django, Flask, and Requests, detailing their uses in scientific computing, data analysis, visualization, machine learning, web development, and H
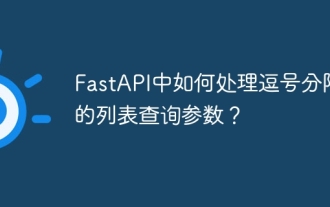
Fastapi ...
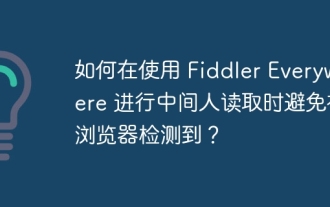
How to avoid being detected when using FiddlerEverywhere for man-in-the-middle readings When you use FiddlerEverywhere...
