JavaScript Basics: Your First Step Into Web Development
?CONTENTS
- Understanding JavaScript
- Setting up Your JavaScript Environment
- Core Syntax and Concepts
- Exploring Control Flow
- JavaScript's Importance in Web Development
- Wrapping Up
?️What is JavaScript?
JavaScript is a versatile programming language crucial for web development. It allows developers to create interactive and dynamic web experiences. Ever wondered how a webpage responds to clicks or other user actions? That's the magic of JavaScript!
From simple form validation to complex web applications, JavaScript is an indispensable tool in modern web development.
?️Setting up Your JavaScript Environment
1. Browser-Based Experiments: You can test JavaScript directly in your browser's developer console. To access it:
- Windows: Press F12 or Ctrl Shift J
- Mac: Press Command Option J
Type console.log("Hello, World!");
and press Enter to see your first JavaScript output.
2. Using a Code Editor: Professionals typically use code editors like Visual Studio Code (VS Code). To begin:
- Install VS Code.
- Create an HTML file and embed your JavaScript using
<script>
tags, or create a separate.js
file.
Example:
console.log("Hello from JavaScript!");
?Core Syntax and Concepts
1. Variables and Constants:
var
, let
, const
: These keywords declare variables to store data.
let
is block-scoped and generally preferred.const
is for values that remain unchanged.
Example:
let username = "MJ"; const age = 26; console.log(`Hello, ${username}! You are ${age} years old.`);
2. Data Types: JavaScript supports various data types:
- Primitive Types: Numbers, Strings, Booleans, Null, Undefined.
- Complex Types: Objects, Arrays.
Example:
let isLearning = true; // Boolean let tools = ["HTML", "CSS", "JavaScript"]; // Array console.log(tools[0]); // Outputs: HTML
?Exploring Control Flow
1. If/Else Statements: Control the execution flow based on conditions.
Example:
let score = 85; if (score >= 90) { console.log("You got an A!"); } else if (score >= 70) { console.log("You passed!"); } else { console.log("Keep trying!"); }
2. Loops: Repeat actions efficiently:
- For Loop:
for (let i = 1; i <= 5; i++) { console.log(i); }
- While Loop:
let count = 0; while (count < 5) { console.log(count); count++; }
**? JavaScript's Importance in Web Development**
JavaScript is the driving force behind interactive web experiences, allowing websites to respond to user input without page reloads.
- Enhances user engagement through interactivity.
- Underpins modern frameworks like React, Angular, and Vue.js.
- Used for both frontend and backend development (e.g., with Node.js).
JavaScript's applications extend beyond websites, encompassing mobile apps and game development.
?Wrapping Up
Mastering JavaScript fundamentals is key to becoming a proficient web developer. Experiment with the concepts presented here and build your own code.
Next Steps: Our next article will delve into JavaScript Functions—essential for creating reusable and efficient code.
Until next time, your friendly neighborhood writer, MJ Bye!!!!
The above is the detailed content of JavaScript Basics: Your First Step Into Web Development. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










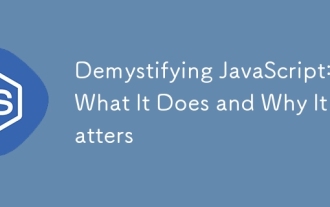
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
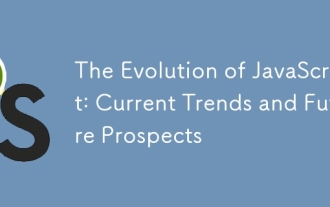
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.
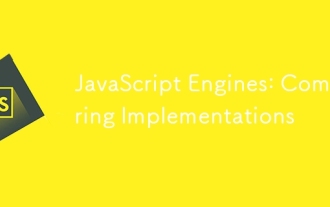
Different JavaScript engines have different effects when parsing and executing JavaScript code, because the implementation principles and optimization strategies of each engine differ. 1. Lexical analysis: convert source code into lexical unit. 2. Grammar analysis: Generate an abstract syntax tree. 3. Optimization and compilation: Generate machine code through the JIT compiler. 4. Execute: Run the machine code. V8 engine optimizes through instant compilation and hidden class, SpiderMonkey uses a type inference system, resulting in different performance performance on the same code.
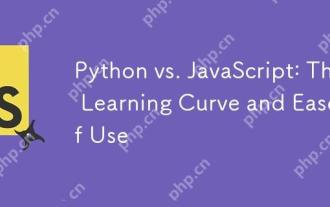
Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
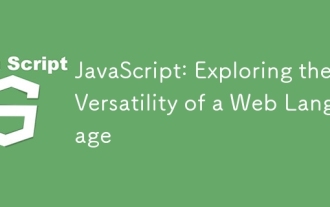
JavaScript is the core language of modern web development and is widely used for its diversity and flexibility. 1) Front-end development: build dynamic web pages and single-page applications through DOM operations and modern frameworks (such as React, Vue.js, Angular). 2) Server-side development: Node.js uses a non-blocking I/O model to handle high concurrency and real-time applications. 3) Mobile and desktop application development: cross-platform development is realized through ReactNative and Electron to improve development efficiency.
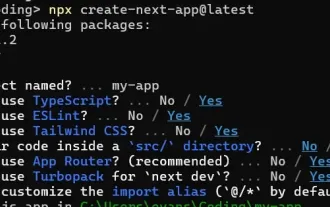
This article demonstrates frontend integration with a backend secured by Permit, building a functional EdTech SaaS application using Next.js. The frontend fetches user permissions to control UI visibility and ensures API requests adhere to role-base
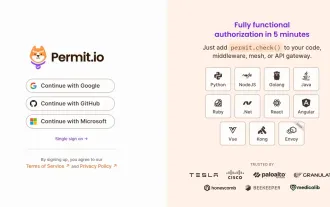
I built a functional multi-tenant SaaS application (an EdTech app) with your everyday tech tool and you can do the same. First, what’s a multi-tenant SaaS application? Multi-tenant SaaS applications let you serve multiple customers from a sing
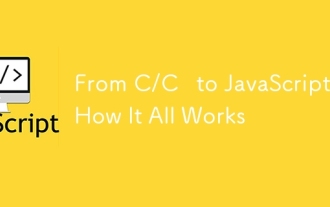
The shift from C/C to JavaScript requires adapting to dynamic typing, garbage collection and asynchronous programming. 1) C/C is a statically typed language that requires manual memory management, while JavaScript is dynamically typed and garbage collection is automatically processed. 2) C/C needs to be compiled into machine code, while JavaScript is an interpreted language. 3) JavaScript introduces concepts such as closures, prototype chains and Promise, which enhances flexibility and asynchronous programming capabilities.
