How to build and deploy a Python library
In software development, many projects involve repetitive tasks using identical code and logic. Programmers constantly rewrite this code, creating inefficiencies. Examples include email validation or random string generation.
A solution is to package this code into reusable libraries. These libraries are installed and used across projects, eliminating redundant coding. Most are open-source and freely available.
This is standard practice in languages like Python and JavaScript. This tutorial details building and deploying a Python library to PyPI, the official Python package repository.
Project Overview
This tutorial guides you through creating and deploying an open-source Python library that interacts with a REST API.
The Python Library
The library will wrap the Abstract API's Exchange Rates REST API. This API offers three endpoints:
-
/live
: Retrieves live exchange rates. -
/convert
: Converts amounts between currencies (e.g., 5 USD to GBP). -
/historical
: Retrieves historical exchange rates for a specified period.
This library simplifies API interaction for developers.
Project Requirements
This tutorial assumes Python coding proficiency, including object-oriented programming (OOP) and function usage. Familiarity with Git, GitHub repositories, and project pushes is also necessary.
Tools and Packages
You'll need:
- Python: Interpreter (>=3.9).
- Requests: For making HTTP requests.
- Abstract API account: To obtain an API key (instructions provided).
- Dotenv: For managing environment variables.
- Poetry: A Python dependency management and packaging tool.
Creating the Project
-
Create a project directory:
mkdir exchangeLibrary
Copy after loginCopy after loginCopy after login -
Install virtualenv (if not already installed):
pip install virtualenv
Copy after loginCopy after loginCopy after login -
Navigate to the project directory:
cd exchangeLibrary
Copy after loginCopy after login -
Create and activate a virtual environment:
virtualenv env
Copy after loginCopy after loginActivate (Windows):
.envScriptsactivate
Activate (Linux/macOS):source env/bin/activate
-
Install required packages:
pip install requests poetry dotenv
Copy after loginCopy after login -
Open the project in a code editor (PyCharm/VS Code).
Setting Up Project Resources
-
Create the
src
directory: Inside, createexchange_python
containing__init__.py
andexchange.py
. Your structure should resemble the image below: -
Create
test.py
for unit testing. -
Obtain your Abstract API key: a. Sign up on Abstract API.
b. Log in. c. Navigate to the Exchange Rates API.
d. Obtain your Primary key.
-
Create a
.env
file: Add your API key:mkdir exchangeLibrary
Copy after loginCopy after loginCopy after loginYour structure should now look like this:
Building the API Wrapper (exchange.py
)
pip install virtualenv
(The live
, historical
, and convert
methods remain as described in the original input.)
Testing the API Wrapper (test.py
)
-
__init__.py
:cd exchangeLibrary
Copy after loginCopy after login -
test.py
:virtualenv env
Copy after loginCopy after login -
Run the tests: You should see a successful test result.
Deploying the Project
-
Create a
.gitignore
file: Addenv
,.env
. -
Create a
README.md
file. -
Create
pyproject.toml
:pip install requests poetry dotenv
Copy after loginCopy after login -
Create a GitHub repository.
-
Initialize Git:
git init
-
Add remote:
git remote add origin <your github repo>
-
Update
pyproject.toml
with your GitHub repo URL. -
Push to GitHub.
Deploying to PyPI
- Create a PyPI account and verify your email.
- Set up 2FA.
- Generate a recovery code.
- Use an authenticator app to scan the QR code.
- Get your API token.
- Build the package:
poetry build
- Set the PyPI token:
poetry config pypi-token.pypi <your-api-token>
- Publish:
poetry publish
Project Maintenance
After updates, push changes to GitHub, build (poetry build
), and republish (poetry publish
), updating the version in pyproject.toml
as needed.
Installation and Usage
mkdir exchangeLibrary
pip install virtualenv
Conclusion
This tutorial covered building and deploying a Python library to PyPI, including updates and republishing. Your contribution to the open-source community helps improve Python development.
The above is the detailed content of How to build and deploy a Python library. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










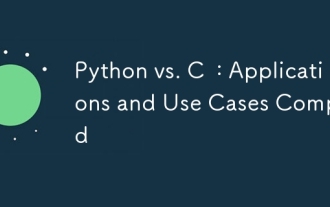
Python is suitable for data science, web development and automation tasks, while C is suitable for system programming, game development and embedded systems. Python is known for its simplicity and powerful ecosystem, while C is known for its high performance and underlying control capabilities.
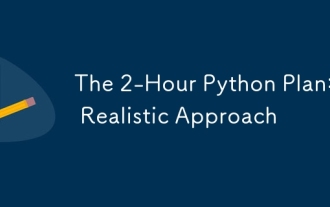
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
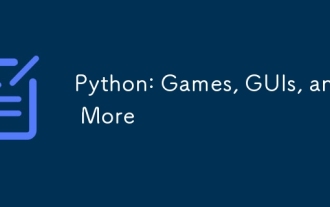
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.
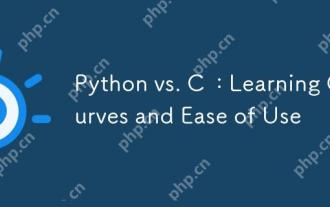
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.
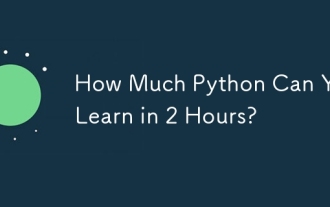
You can learn the basics of Python within two hours. 1. Learn variables and data types, 2. Master control structures such as if statements and loops, 3. Understand the definition and use of functions. These will help you start writing simple Python programs.
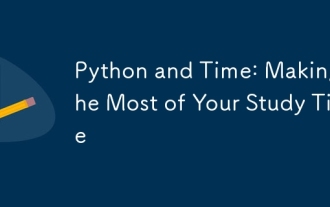
To maximize the efficiency of learning Python in a limited time, you can use Python's datetime, time, and schedule modules. 1. The datetime module is used to record and plan learning time. 2. The time module helps to set study and rest time. 3. The schedule module automatically arranges weekly learning tasks.
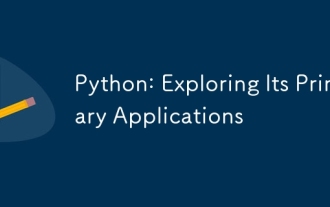
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
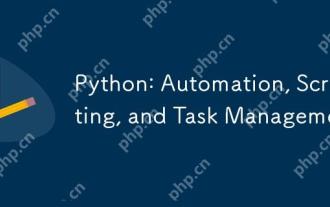
Python excels in automation, scripting, and task management. 1) Automation: File backup is realized through standard libraries such as os and shutil. 2) Script writing: Use the psutil library to monitor system resources. 3) Task management: Use the schedule library to schedule tasks. Python's ease of use and rich library support makes it the preferred tool in these areas.
