


Solving `Uncaught (in promise) ReferenceError: exports is not defined` in Nuxt.js with Vee-Validate
When using vee-validate in a Nuxt.js project, I encountered the following error:
<code>Uncaught (in promise) ReferenceError: exports is not defined</code>
This is not an uncommon problem! This error is usually caused by incorrect transpilation of the vee-validate library, which uses modern ES modules. This article explains how to fix this problem and make sure everything runs smoothly.
Error reason
This error occurs because Nuxt.js (especially when running in SSR mode) sometimes requires explicit instructions to transpile modern libraries like vee-validate. Without these directives, the library might try to use exports (a CommonJS feature) in an ES module context, causing errors.
Solution
1. Ensure version compatibility
Before making configuration changes, please confirm that you are using the correct version of Nuxt and Vue:
- Nuxt 3 Vue 3: Use vee-validate v4.
- Nuxt 2 Vue 2: Use vee-validate v3 unless you are using the Vue Composition API plugin, which works with v4.
2. Translation Vee-Validate
Add vee-validate to the nuxt.config
options in your transpile
file.
For Nuxt 3:
<code>// nuxt.config.ts export default defineNuxtConfig({ build: { transpile: ['vee-validate'] } })</code>
For Nuxt 2:
<code>// nuxt.config.js export default { build: { transpile: ['vee-validate'] } }</code>
This will ensure that Nuxt converts vee-validate correctly for both client and server environments.
3. Example of form settings using Vee-Validate
Here's a quick example of schema validation using vee-validate and yup in Nuxt 3:
<code>import { useForm, useField } from 'vee-validate' import * as yup from 'yup' // 定义验证模式 const formSchema = yup.object({ email: yup.string().required('Email is required').email('Invalid email'), password: yup.string().required('Password is required').min(6, 'Password must be at least 6 characters') }) // 使用模式初始化表单 const { handleSubmit, errors } = useForm({ validationSchema: formSchema }) // 定义表单字段 const { value: email, errorMessage: emailError } = useField('email') const { value: password, errorMessage: passwordError } = useField('password') // 处理表单提交 const onSubmit = handleSubmit((values) => { console.log('Form values:', values) }) <template> <div> <label for="email">Email</label> <hr></hr><h3> 4. **检查您的 Node 版本** </h3> <p>确保您正在运行 <strong>Node.js 14 或更高版本</strong>,因为较旧的版本可能无法很好地处理现代 ES 模块语法。</p> <hr></hr><h2> 最终总结 </h2> <p>通过将 vee-validate 添加到 transpile 配置中,您可以避免令人沮丧的“exports is not defined”错误,并在您的 Nuxt.js 项目中充分利用 vee-validate 的强大功能。如果您仍然遇到问题,请仔细检查您的软件包版本和项目设置。</p> <p>祝您编码愉快!?</p> <hr></hr><h3> 相关链接 </h3> <ul><li>Vee-Validate 文档</li> <li>Nuxt.js 转译指南</li> </ul><p>有问题?请留言或分享您的经验!</p> </div> </template></code>
The above is the detailed content of Solving `Uncaught (in promise) ReferenceError: exports is not defined` in Nuxt.js with Vee-Validate. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










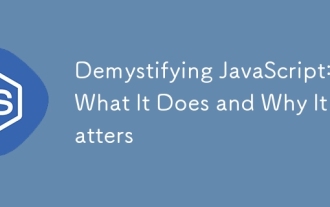
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
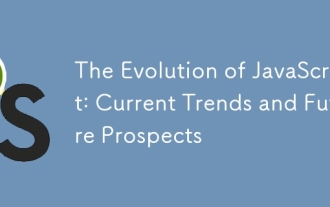
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.
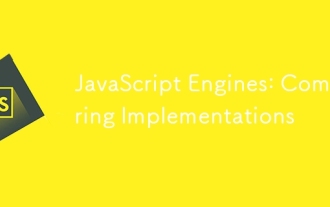
Different JavaScript engines have different effects when parsing and executing JavaScript code, because the implementation principles and optimization strategies of each engine differ. 1. Lexical analysis: convert source code into lexical unit. 2. Grammar analysis: Generate an abstract syntax tree. 3. Optimization and compilation: Generate machine code through the JIT compiler. 4. Execute: Run the machine code. V8 engine optimizes through instant compilation and hidden class, SpiderMonkey uses a type inference system, resulting in different performance performance on the same code.
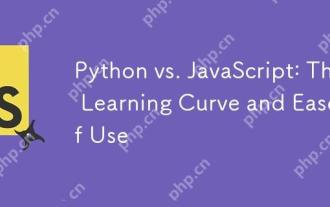
Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
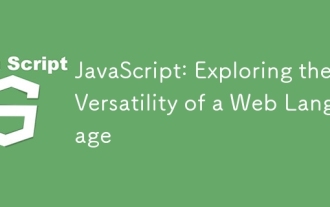
JavaScript is the core language of modern web development and is widely used for its diversity and flexibility. 1) Front-end development: build dynamic web pages and single-page applications through DOM operations and modern frameworks (such as React, Vue.js, Angular). 2) Server-side development: Node.js uses a non-blocking I/O model to handle high concurrency and real-time applications. 3) Mobile and desktop application development: cross-platform development is realized through ReactNative and Electron to improve development efficiency.
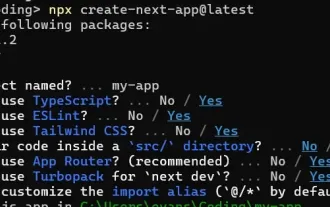
This article demonstrates frontend integration with a backend secured by Permit, building a functional EdTech SaaS application using Next.js. The frontend fetches user permissions to control UI visibility and ensures API requests adhere to role-base
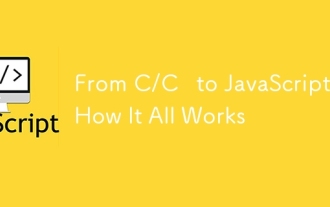
The shift from C/C to JavaScript requires adapting to dynamic typing, garbage collection and asynchronous programming. 1) C/C is a statically typed language that requires manual memory management, while JavaScript is dynamically typed and garbage collection is automatically processed. 2) C/C needs to be compiled into machine code, while JavaScript is an interpreted language. 3) JavaScript introduces concepts such as closures, prototype chains and Promise, which enhances flexibility and asynchronous programming capabilities.
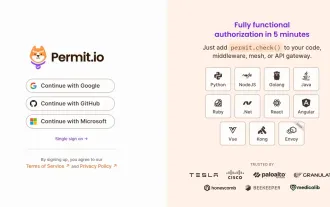
I built a functional multi-tenant SaaS application (an EdTech app) with your everyday tech tool and you can do the same. First, what’s a multi-tenant SaaS application? Multi-tenant SaaS applications let you serve multiple customers from a sing
