


How Can I Use OR Logic for Concatenating WHERE Clauses in Dynamic LINQ to Entities Queries?
Building Dynamic LINQ to Entities Queries with OR Conditions
Creating dynamic LINQ queries often requires combining multiple WHERE clauses. While standard LINQ easily handles AND conditions, constructing dynamic OR conditions presents a challenge. This article demonstrates a solution using LINQKit's PredicateBuilder
to achieve this.
The key is leveraging PredicateBuilder
for flexible predicate creation and ensuring compatibility with Entity Framework. The following code exemplifies this approach:
var query = from u in context.Users select u; var pred = PredicateBuilder.False<User>(); // Start with a predicate that's always false if (type.HasFlag(IdentifierType.Username)) pred = pred.Or(u => u.Username == identifier); if (type.HasFlag(IdentifierType.Windows)) pred = pred.Or(u => u.WindowsUsername == identifier); // Corrected parenthesis return query.Where(pred.Expand()).FirstOrDefault(); // or return query.AsExpandable().Where(pred).FirstOrDefault();
Crucially, notice the use of pred.Expand()
or query.AsExpandable()
. This is essential because PredicateBuilder
creates expressions that Entity Framework doesn't directly support. Expand()
(or AsExpandable()
) utilizes LINQKit's expression visitor to translate these expressions into a form compatible with Entity Framework, preventing exceptions.
As an alternative, consider exploring other PredicateBuilder
implementations, such as the one by Peter Montgomery (https://www.php.cn/link/451e10de8e2fb18a9f795679b52dc9f6), which might offer similar functionality without requiring the explicit Expand()
call. This provides a streamlined approach to constructing dynamic OR conditions within your LINQ to Entities queries.
The above is the detailed content of How Can I Use OR Logic for Concatenating WHERE Clauses in Dynamic LINQ to Entities Queries?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


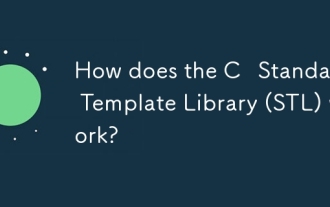
This article explains the C Standard Template Library (STL), focusing on its core components: containers, iterators, algorithms, and functors. It details how these interact to enable generic programming, improving code efficiency and readability t
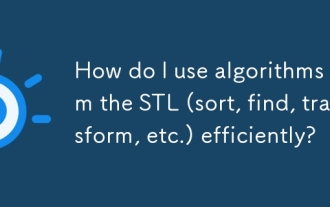
This article details efficient STL algorithm usage in C . It emphasizes data structure choice (vectors vs. lists), algorithm complexity analysis (e.g., std::sort vs. std::partial_sort), iterator usage, and parallel execution. Common pitfalls like
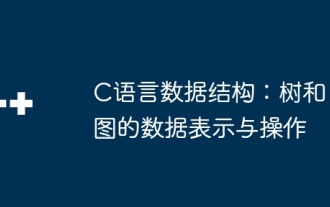
C language data structure: The data representation of the tree and graph is a hierarchical data structure consisting of nodes. Each node contains a data element and a pointer to its child nodes. The binary tree is a special type of tree. Each node has at most two child nodes. The data represents structTreeNode{intdata;structTreeNode*left;structTreeNode*right;}; Operation creates a tree traversal tree (predecision, in-order, and later order) search tree insertion node deletes node graph is a collection of data structures, where elements are vertices, and they can be connected together through edges with right or unrighted data representing neighbors.
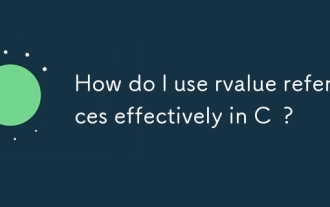
Article discusses effective use of rvalue references in C for move semantics, perfect forwarding, and resource management, highlighting best practices and performance improvements.(159 characters)
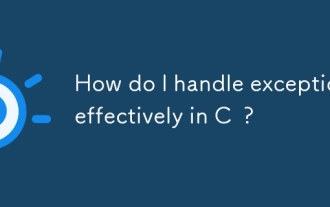
This article details effective exception handling in C , covering try, catch, and throw mechanics. It emphasizes best practices like RAII, avoiding unnecessary catch blocks, and logging exceptions for robust code. The article also addresses perf
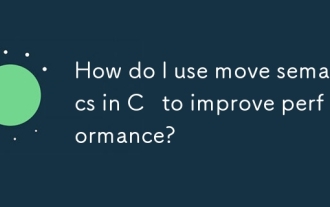
The article discusses using move semantics in C to enhance performance by avoiding unnecessary copying. It covers implementing move constructors and assignment operators, using std::move, and identifies key scenarios and pitfalls for effective appl
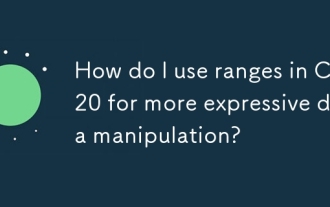
C 20 ranges enhance data manipulation with expressiveness, composability, and efficiency. They simplify complex transformations and integrate into existing codebases for better performance and maintainability.
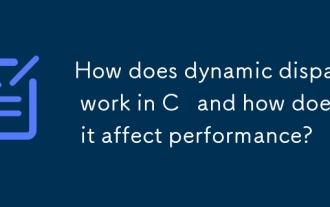
The article discusses dynamic dispatch in C , its performance costs, and optimization strategies. It highlights scenarios where dynamic dispatch impacts performance and compares it with static dispatch, emphasizing trade-offs between performance and
