Web Frameworks: The Future
Recently, a livestream host sparked an interesting thought: "In a decade, React might not be my go-to framework." This got me pondering the evolution of web frameworks. Let's explore some potential directions.
Syntax: A Blend of HTML and JSX
For those familiar with HTML, whether through server-side rendering, CodePen experiments, or even Tumblr customization, a familiar syntax is key. Consider this:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 |
|
This resembles Svelte's approach, enhancing HTML's inherent structure. A more modern iteration might look like:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 |
|
Markup remains in HTML, CSS in <style>
, and JavaScript in <script>
. It feels like component-based, modern HTML. However, building a website requires server-side interaction.
Server-Side Rendering with JSX
Web servers handle database connections, authentication, and data processing before sending assets to the browser. A typical server-side route might look like:
1 2 3 4 5 6 7 8 |
|
Using JSX for server-rendered pages makes intuitive sense:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
|
This resembles a React component but resides entirely on the server. Client-side JavaScript remains crucial for interactivity.
The Best of Both Worlds
Let's combine server-side JSX with client-side interactivity using a system similar to Remix's loaders and actions, or React Server Components (RSC), but without explicit directives.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 |
|
The framework would intelligently identify updateCount
as an RPC call based on its context.
Reactivity: Signals for Speed
A lightweight, fast reactivity system is essential. Svelte's Signals are a strong candidate:
1 2 3 4 5 6 7 8 9 10 11 12 13 |
|
Data Fetching: Implicit Server Actions
Instead of explicit directives like 'use server'
, we could leverage language features. Imagine a keyword like action
to designate functions for server-side processing:
1 2 3 4 5 6 7 |
|
This simplifies the code while maintaining clear separation of client and server logic.
Conclusion: A Vision, Not a Proposal
This exploration isn't a concrete framework proposal, but a thought experiment. The goal is to envision a framework that blends the best aspects of existing technologies, offering a simpler, more intuitive development experience for the next decade. What are your thoughts on the future of web frameworks?
The above is the detailed content of Web Frameworks: The Future. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
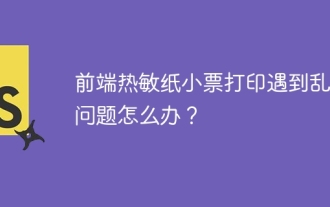
Frequently Asked Questions and Solutions for Front-end Thermal Paper Ticket Printing In Front-end Development, Ticket Printing is a common requirement. However, many developers are implementing...
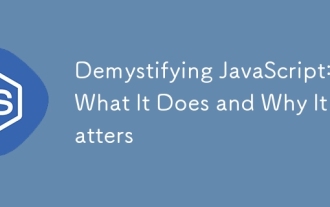
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
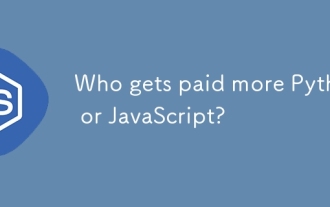
There is no absolute salary for Python and JavaScript developers, depending on skills and industry needs. 1. Python may be paid more in data science and machine learning. 2. JavaScript has great demand in front-end and full-stack development, and its salary is also considerable. 3. Influencing factors include experience, geographical location, company size and specific skills.
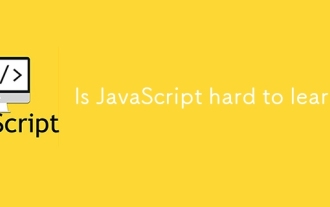
Learning JavaScript is not difficult, but it is challenging. 1) Understand basic concepts such as variables, data types, functions, etc. 2) Master asynchronous programming and implement it through event loops. 3) Use DOM operations and Promise to handle asynchronous requests. 4) Avoid common mistakes and use debugging techniques. 5) Optimize performance and follow best practices.
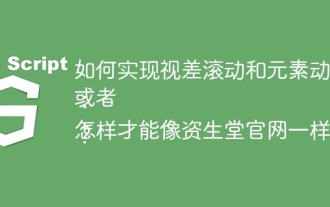
Discussion on the realization of parallax scrolling and element animation effects in this article will explore how to achieve similar to Shiseido official website (https://www.shiseido.co.jp/sb/wonderland/)...
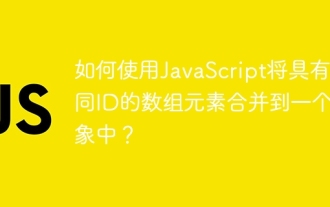
How to merge array elements with the same ID into one object in JavaScript? When processing data, we often encounter the need to have the same ID...
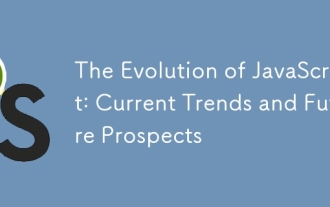
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.
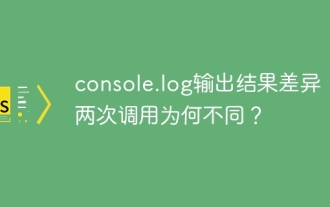
In-depth discussion of the root causes of the difference in console.log output. This article will analyze the differences in the output results of console.log function in a piece of code and explain the reasons behind it. �...
