How to maximize crawler efficiency?
In the data-driven era, web crawlers have become an important tool for obtaining Internet information. However, in the face of massive data and complex network environments, how to improve crawler efficiency has become the focus of every crawler developer. This article will discuss how to maximize crawler efficiency from multiple dimensions, including optimizing crawler strategies, using efficient tools, and rationally utilizing proxy IP, and briefly mentions 98IP proxy as one of the solutions.
1. Optimize crawler strategy
1.1 Reasonable request frequency
Note: Excessive request frequency may cause excessive pressure on the target website server and even trigger the anti-crawler mechanism. However, if the request frequency is too low, it will reduce the data collection efficiency. Therefore, a reasonable request frequency needs to be set based on the load capacity of the target website and the anti-crawler strategy.
Implementation method:
import time import random def sleep_between_requests(min_seconds, max_seconds): time.sleep(random.uniform(min_seconds, max_seconds)) # 示例:每次请求后随机等待1到3秒 sleep_between_requests(1, 3)
1.2 Concurrent Requests
Note: Realizing concurrent requests through multi-threading, asynchronous request and other technologies can significantly improve the speed of data capture. However, it should be noted that the number of concurrent requests must match the carrying capacity of the target website server to avoid triggering the anti-crawler mechanism.
Implementation method (asynchronous request example):
import aiohttp import asyncio async def fetch(session, url): async with session.get(url) as response: return await response.text() async def main(): urls = ['http://example.com/page1', 'http://example.com/page2', ...] async with aiohttp.ClientSession() as session: tasks = [fetch(session, url) for url in urls] htmls = await asyncio.gather(*tasks) # 运行异步请求 asyncio.run(main())
2. Use efficient tools
2.1 Choose a suitable crawler framework
Note: Different crawler frameworks, such as Scrapy, BeautifulSoup, Selenium, etc., have different applicable scenarios and performance. Choosing the right framework can greatly simplify the development process and improve crawler efficiency.
2.2 Data storage optimization
Note: Using efficient database storage solutions, such as MongoDB, Redis, etc., can speed up data writing and reduce I/O waiting time.
Implementation method (MongoDB example):
from pymongo import MongoClient client = MongoClient('mongodb://localhost:27017/') db = client['mydatabase'] collection = db['mycollection'] # 插入数据 data = {'name': 'example', 'value': 123} collection.insert_one(data)
3. Proper use of proxy IP
3.1 The role of proxy IP
Note: Using proxy IP can hide the real IP address of the crawler and avoid being banned by the target website. At the same time, using proxy IPs distributed in different geographical locations can simulate real user access and improve the success rate of data capture.
3.2 Choose a reliable proxy service provider
Note: Choosing a reliable service provider like 98IP Proxy can ensure the stability, anonymity and availability of the proxy IP.
Implementation method (using proxy IP example):
import requests proxies = { 'http': 'http://proxy_ip:port', 'https': 'https://proxy_ip:port', } url = 'http://example.com' response = requests.get(url, proxies=proxies) print(response.text)
Note: When using a proxy IP, you need to change the proxy IP regularly to avoid a single IP being blocked due to frequent requests.
4. Other optimization measures
4.1 Intelligent identification and anti-crawler strategy
Description: By analyzing the target website’s request headers, cookies, verification codes and other anti-crawler mechanisms, intelligently adjust the crawler strategy to improve the success rate of data capture.
4.2 Distributed crawler architecture
Description: Establishing a distributed crawler architecture and allocating tasks to multiple machines for parallel execution can significantly improve the speed and scale of data crawling.
5. Summary
Improving crawler efficiency is a systematic project that requires comprehensive consideration from multiple aspects such as strategy optimization, tool selection, and proxy IP utilization. Through reasonable request frequency, concurrent requests, choosing an appropriate crawler framework, optimizing data storage, utilizing proxy IP, and intelligently identifying anti-crawler strategies, crawler efficiency can be maximized. At the same time, with the continuous development of technology, continuous learning and exploration of new crawler technologies and tools are also the key to improving crawler efficiency.
The above is the detailed content of How to maximize crawler efficiency?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










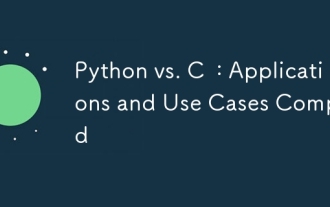
Python is suitable for data science, web development and automation tasks, while C is suitable for system programming, game development and embedded systems. Python is known for its simplicity and powerful ecosystem, while C is known for its high performance and underlying control capabilities.
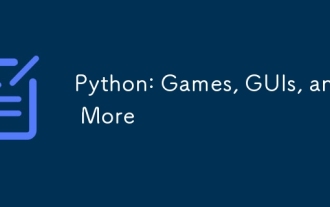
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.
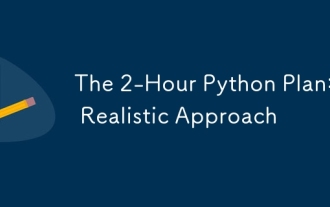
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
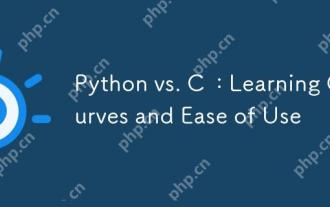
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.
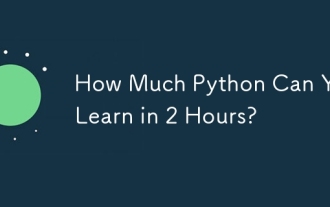
You can learn the basics of Python within two hours. 1. Learn variables and data types, 2. Master control structures such as if statements and loops, 3. Understand the definition and use of functions. These will help you start writing simple Python programs.
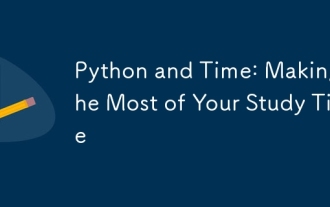
To maximize the efficiency of learning Python in a limited time, you can use Python's datetime, time, and schedule modules. 1. The datetime module is used to record and plan learning time. 2. The time module helps to set study and rest time. 3. The schedule module automatically arranges weekly learning tasks.
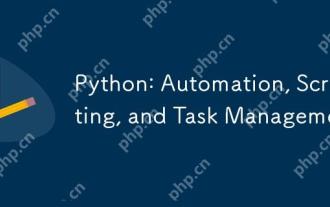
Python excels in automation, scripting, and task management. 1) Automation: File backup is realized through standard libraries such as os and shutil. 2) Script writing: Use the psutil library to monitor system resources. 3) Task management: Use the schedule library to schedule tasks. Python's ease of use and rich library support makes it the preferred tool in these areas.
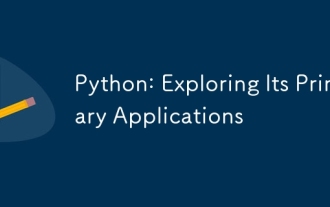
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
