How Do I Determine the Start of the Week (Monday or Sunday) in C#?
Determine the start date of the week (Monday or Sunday) in C#
In C#, determining the start date of the week is useful for various scenarios such as scheduling or data aggregation. To achieve this we need to consider the definition of the start of the week, which may vary depending on the specific engagement or region.
Start the week on Monday
For regions that start the week on Monday, we can use the extension method:
public static class DateTimeExtensions { public static DateTime StartOfWeek(this DateTime dt) { int diff = dt.DayOfWeek - DayOfWeek.Monday; return dt.AddDays(-diff).Date; } }
How to use:
DateTime mondayStart = DateTime.Now.StartOfWeek();
Start the week on Sunday
For regions that start the week on Sunday, we can modify the extension method slightly:
public static class DateTimeExtensions { public static DateTime StartOfWeek(this DateTime dt) { int diff = dt.DayOfWeek - DayOfWeek.Sunday; return dt.AddDays(-diff).Date; } }
How to use:
DateTime sundayStart = DateTime.Now.StartOfWeek();
These extension methods provide a flexible and customizable way to determine the start date of the week based on the desired convention.
The above is the detailed content of How Do I Determine the Start of the Week (Monday or Sunday) in C#?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


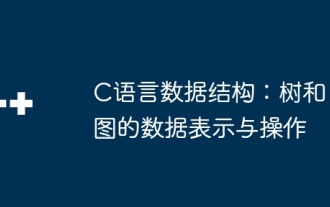
C language data structure: The data representation of the tree and graph is a hierarchical data structure consisting of nodes. Each node contains a data element and a pointer to its child nodes. The binary tree is a special type of tree. Each node has at most two child nodes. The data represents structTreeNode{intdata;structTreeNode*left;structTreeNode*right;}; Operation creates a tree traversal tree (predecision, in-order, and later order) search tree insertion node deletes node graph is a collection of data structures, where elements are vertices, and they can be connected together through edges with right or unrighted data representing neighbors.
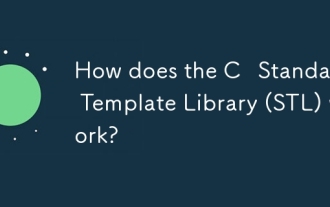
This article explains the C Standard Template Library (STL), focusing on its core components: containers, iterators, algorithms, and functors. It details how these interact to enable generic programming, improving code efficiency and readability t
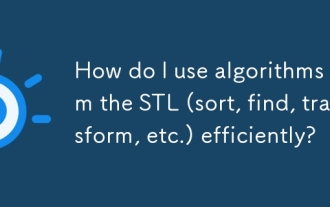
This article details efficient STL algorithm usage in C . It emphasizes data structure choice (vectors vs. lists), algorithm complexity analysis (e.g., std::sort vs. std::partial_sort), iterator usage, and parallel execution. Common pitfalls like
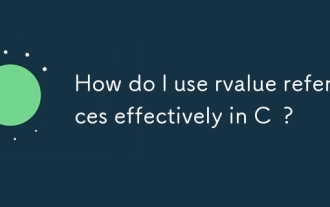
Article discusses effective use of rvalue references in C for move semantics, perfect forwarding, and resource management, highlighting best practices and performance improvements.(159 characters)
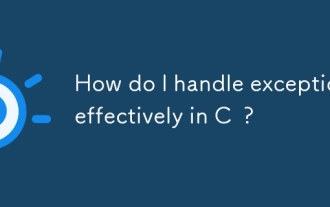
This article details effective exception handling in C , covering try, catch, and throw mechanics. It emphasizes best practices like RAII, avoiding unnecessary catch blocks, and logging exceptions for robust code. The article also addresses perf
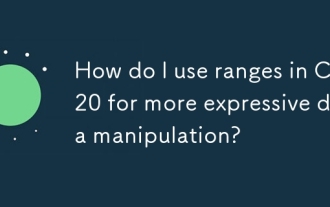
C 20 ranges enhance data manipulation with expressiveness, composability, and efficiency. They simplify complex transformations and integrate into existing codebases for better performance and maintainability.
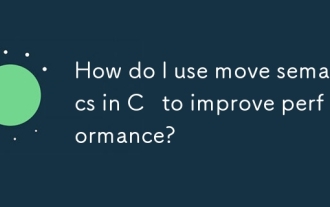
The article discusses using move semantics in C to enhance performance by avoiding unnecessary copying. It covers implementing move constructors and assignment operators, using std::move, and identifies key scenarios and pitfalls for effective appl
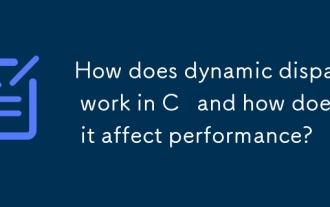
The article discusses dynamic dispatch in C , its performance costs, and optimization strategies. It highlights scenarios where dynamic dispatch impacts performance and compares it with static dispatch, emphasizing trade-offs between performance and
