SUM OF TWO INTEGERS - leetcode - Python
Let's get to the core of adding two integers without using the ' ' operator. This requires binary manipulation.
We'll approach this like regular addition, but using binary.
- Start adding from the right, just as you normally would: 1 1, 0 1, 1 0, 0 0.
- Since we're working in binary, if the sum reaches 2, reset it to 0 (1 1 = 10 binary, becomes 0 with a carry).
- Repeat this for all bits. This gives us a partial sum, ignoring carries for now.
The XOR (^) bitwise operator handles this initial sum perfectly:
- If bits are the same, the result is 0. If they're different, the result is 1.
This aligns with our needs: 1 1 → 0 (with a carry), 0 1 or 1 0 → 1, and 0 0 → 0.
Now, let's address the carries. The AND (&) operator helps us find them:
- If both bits are 1, the result is 1 (a carry).
To shift the carry to the left, we'll use a left bit shift.
Algorithm:
-
Initialization:
-
sum = a ^ b
(XOR for sum without carry) -
carry = (a & b)
(AND for carry)
-
-
Iteration:
- Repeat until
carry == 0
:a = sum
-
b = carry << 1
(left bit shift for carry)
- Repeat until
Example (5 3):
- Initial Values:
- Iteration 1:
sum = 0101 ^ 0011 = 0110
carry = 0101 & 0011 = 0001
- Iteration 2:
sum = 0110 ^ 0010 = 0100
carry = 0110 & 0010 = 0010
- Iteration 3:
sum = 0100 ^ 00100 = 0000
carry = 0100 & 0100 = 0100
- Iteration 4:
sum = 0000 ^ 1000 = 1000
carry = 0000 & 1000 = 0000
The carry is 0, so the final sum is 1000 (8).
Python's unbounded integers cause issues with negative numbers. The left bit shift can lead to infinite growth. To fix this, we need to simulate fixed-size integers (e.g., 32-bit).
We'll use a 32-bit mask (0xFFFFFFFF) to limit the number of bits:
This ensures that only the last 32 bits are considered, preventing infinite growth. We also handle potential negative results by converting them to their 32-bit two's complement representation if necessary.
This approach effectively simulates 32-bit integer arithmetic within Python, resolving the issue with unbounded integers and negative numbers. The if a > MAX_INT
condition ensures that the result remains within the 32-bit signed integer range. The example with -12 and -8 demonstrates how this correction works to produce the expected result of -20.
My Name is Jaimin Bariya, if you find something useful, please like and comment, and follow me on github jaimin-bariya
The above is the detailed content of SUM OF TWO INTEGERS - leetcode - Python. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










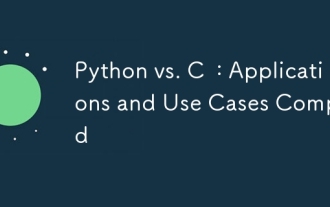
Python is suitable for data science, web development and automation tasks, while C is suitable for system programming, game development and embedded systems. Python is known for its simplicity and powerful ecosystem, while C is known for its high performance and underlying control capabilities.
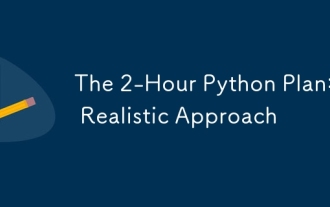
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
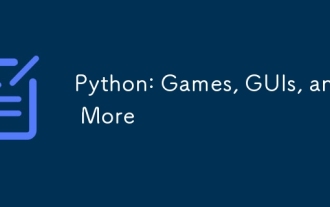
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.
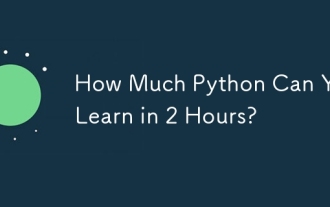
You can learn the basics of Python within two hours. 1. Learn variables and data types, 2. Master control structures such as if statements and loops, 3. Understand the definition and use of functions. These will help you start writing simple Python programs.
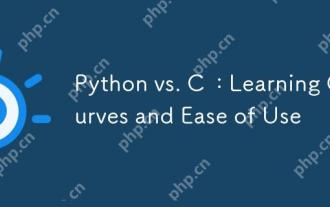
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.
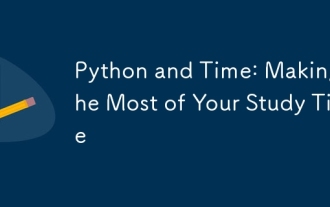
To maximize the efficiency of learning Python in a limited time, you can use Python's datetime, time, and schedule modules. 1. The datetime module is used to record and plan learning time. 2. The time module helps to set study and rest time. 3. The schedule module automatically arranges weekly learning tasks.
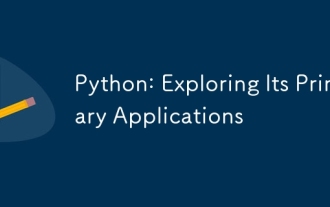
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
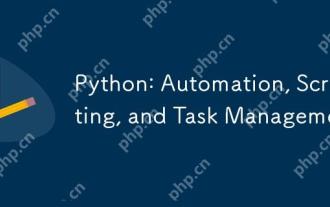
Python excels in automation, scripting, and task management. 1) Automation: File backup is realized through standard libraries such as os and shutil. 2) Script writing: Use the psutil library to monitor system resources. 3) Task management: Use the schedule library to schedule tasks. Python's ease of use and rich library support makes it the preferred tool in these areas.
