


How Can I Efficiently Generate Number Sequences in SQL Using a UDF or Auxiliary Table?
Generating Number Sequences in SQL: UDFs and Auxiliary Tables
Need to efficiently generate number sequences within your SQL queries? Avoid complex loops or recursive CTEs; instead, leverage the power of user-defined functions (UDFs) or auxiliary tables. This approach offers simplicity and performance advantages.
Method 1: User-Defined Function (UDF)
This UDF generates a numerical sequence within a specified range:
CREATE FUNCTION GetNumberSequence ( @Start INT, @End INT ) RETURNS TABLE AS BEGIN RETURN ( SELECT ROW_NUMBER() OVER (ORDER BY (SELECT NULL)) AS N FROM sys.all_columns AS ac1 CROSS JOIN sys.all_columns AS ac2 WHERE ROW_NUMBER() OVER (ORDER BY (SELECT NULL)) BETWEEN @Start AND @End ); END;
This function takes a starting value (@Start
) and an ending value (@End
) as input. The ROW_NUMBER()
function assigns a unique number to each row, and the CROSS JOIN
efficiently generates the sequence.
Usage Example:
To generate numbers from 1 to 100:
SELECT * FROM GetNumberSequence(1, 100);
Method 2: Permanent Auxiliary Table
For frequently used sequences, a permanent auxiliary table offers superior performance. Create a table like this:
CREATE TABLE dbo.Numbers ( N INT PRIMARY KEY ); INSERT INTO dbo.Numbers (N) SELECT ROW_NUMBER() OVER (ORDER BY (SELECT NULL)) FROM sys.all_columns AS ac1 CROSS JOIN sys.all_columns AS ac2;
This creates a table (dbo.Numbers
) containing a large sequence of numbers. You can then easily query this table to retrieve your desired sequence.
Choosing the Right Method
Both methods are effective. The UDF is ideal for one-off sequence generation, while the permanent auxiliary table is best for frequently accessed sequences, offering significant performance gains. The choice depends on your application's specific requirements and frequency of sequence generation.
The above is the detailed content of How Can I Efficiently Generate Number Sequences in SQL Using a UDF or Auxiliary Table?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










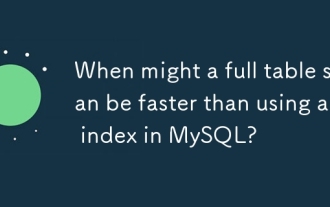
Full table scanning may be faster in MySQL than using indexes. Specific cases include: 1) the data volume is small; 2) when the query returns a large amount of data; 3) when the index column is not highly selective; 4) when the complex query. By analyzing query plans, optimizing indexes, avoiding over-index and regularly maintaining tables, you can make the best choices in practical applications.
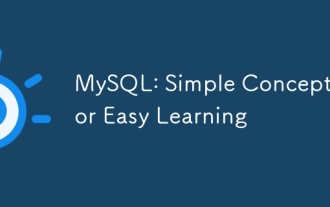
MySQL is an open source relational database management system. 1) Create database and tables: Use the CREATEDATABASE and CREATETABLE commands. 2) Basic operations: INSERT, UPDATE, DELETE and SELECT. 3) Advanced operations: JOIN, subquery and transaction processing. 4) Debugging skills: Check syntax, data type and permissions. 5) Optimization suggestions: Use indexes, avoid SELECT* and use transactions.
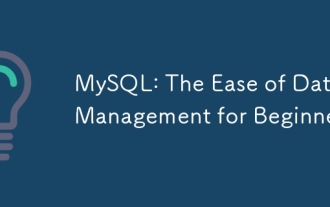
MySQL is suitable for beginners because it is simple to install, powerful and easy to manage data. 1. Simple installation and configuration, suitable for a variety of operating systems. 2. Support basic operations such as creating databases and tables, inserting, querying, updating and deleting data. 3. Provide advanced functions such as JOIN operations and subqueries. 4. Performance can be improved through indexing, query optimization and table partitioning. 5. Support backup, recovery and security measures to ensure data security and consistency.
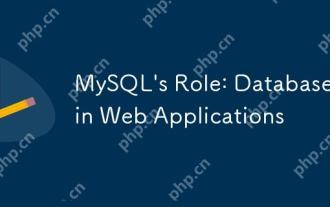
The main role of MySQL in web applications is to store and manage data. 1.MySQL efficiently processes user information, product catalogs, transaction records and other data. 2. Through SQL query, developers can extract information from the database to generate dynamic content. 3.MySQL works based on the client-server model to ensure acceptable query speed.
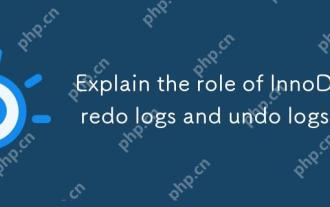
InnoDB uses redologs and undologs to ensure data consistency and reliability. 1.redologs record data page modification to ensure crash recovery and transaction persistence. 2.undologs records the original data value and supports transaction rollback and MVCC.
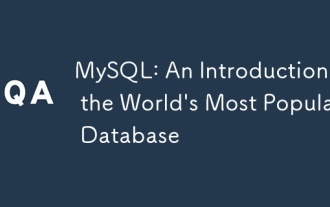
MySQL is an open source relational database management system, mainly used to store and retrieve data quickly and reliably. Its working principle includes client requests, query resolution, execution of queries and return results. Examples of usage include creating tables, inserting and querying data, and advanced features such as JOIN operations. Common errors involve SQL syntax, data types, and permissions, and optimization suggestions include the use of indexes, optimized queries, and partitioning of tables.
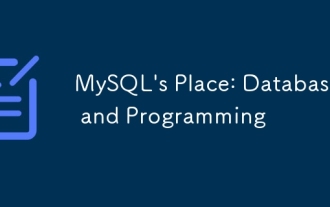
MySQL's position in databases and programming is very important. It is an open source relational database management system that is widely used in various application scenarios. 1) MySQL provides efficient data storage, organization and retrieval functions, supporting Web, mobile and enterprise-level systems. 2) It uses a client-server architecture, supports multiple storage engines and index optimization. 3) Basic usages include creating tables and inserting data, and advanced usages involve multi-table JOINs and complex queries. 4) Frequently asked questions such as SQL syntax errors and performance issues can be debugged through the EXPLAIN command and slow query log. 5) Performance optimization methods include rational use of indexes, optimized query and use of caches. Best practices include using transactions and PreparedStatemen
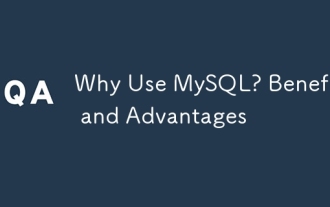
MySQL is chosen for its performance, reliability, ease of use, and community support. 1.MySQL provides efficient data storage and retrieval functions, supporting multiple data types and advanced query operations. 2. Adopt client-server architecture and multiple storage engines to support transaction and query optimization. 3. Easy to use, supports a variety of operating systems and programming languages. 4. Have strong community support and provide rich resources and solutions.
