How to Deserialize Line-Delimited JSON in C# using JSON.NET?
Jan 22, 2025 pm 04:52 PMSerialization and deserialization of newline-delimited JSON data in C#
In C#, you can leverage the JSON.NET library to process newline-delimited JSON data. This format of JSON data is essential for interacting with services like Google BigQuery.
Serialization and deserialization process
You can use JsonTextReader
to parse newline-delimited JSON data. The specific steps are as follows:
- Create a POCO class (such as
Foo
orPerson
) to represent the JSON data structure. - Initialize the
SupportMultipleContent
object using thetrue
flag set toJsonTextReader
. This is essential for handling multiple JSON objects in a single stream. - Deserialize each JSON object using
JsonSerializer
.
If you need to store the deserialized results as a list, add each deserialized item to the list in a loop.
Example 1: Simple JSON data
For the following JSON data:
<code>{"some":"thing1"} {"some":"thing2"} {"some":"thing3"}</code>
The following code demonstrates the deserialization process:
var json = "{\"some\":\"thing1\"}\r\n{\"some\":\"thing2\"}\r\n{\"some\":\"thing3\"}"; var jsonReader = new JsonTextReader(new StringReader(json)) { SupportMultipleContent = true }; var jsonSerializer = new JsonSerializer(); var fooList = new List<Foo>(); while (jsonReader.Read()) { fooList.Add(jsonSerializer.Deserialize<Foo>(jsonReader)); }
Example 2: Complex JSON data
For more complex JSON data:
<code>{"kind": "person", "fullName": "John Doe", "age": 22, "gender": "Male", "citiesLived": [{ "place": "Seattle", "numberOfYears": 5}, {"place": "Stockholm", "numberOfYears": 6}]} {"kind": "person", "fullName": "Jane Austen", "age": 24, "gender": "Female", "citiesLived": [{"place": "Los Angeles", "numberOfYears": 2}, {"place": "Tokyo", "numberOfYears": 2}]}</code>
Create a Person
POCO class and deserialize using the same method as Example 1.
It is important to note that this technology also supports comma-delimited JSON entries using Json.Net 10.0.4 and higher.
The above is the detailed content of How to Deserialize Line-Delimited JSON in C# using JSON.NET?. For more information, please follow other related articles on the PHP Chinese website!

Hot Article

Hot tools Tags

Hot Article

Hot Article Tags

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
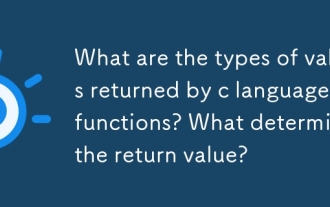
What are the types of values returned by c language functions? What determines the return value?

What are the definitions and calling rules of c language functions and what are the

Where is the return value of the c language function stored in memory?
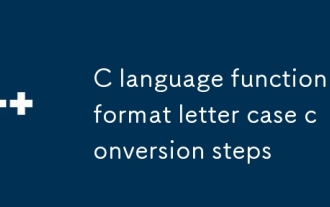
C language function format letter case conversion steps
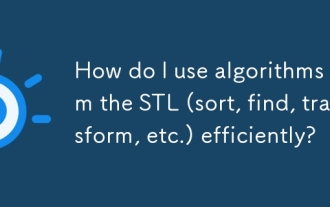
How do I use algorithms from the STL (sort, find, transform, etc.) efficiently?
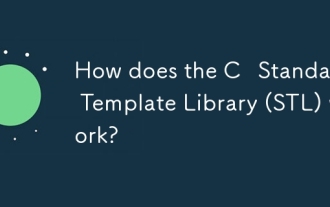
How does the C Standard Template Library (STL) work?
