Flow Control Statements in Go
The flow control statements of Go language are the basis of its programming. Like other languages, they control the program execution flow and implement decision-making, looping and resource management. This article takes an in-depth look at Go’s flow control statements, including for
, if
, switch
, and defer
, and explains how to use them effectively in Go programs.
This article is part of the Go language tutorial series, designed to help developers understand the Go language more deeply. Whether you are a beginner or an experienced developer, this guide will give you the knowledge you need to write more efficient and readable Go code.
After reading this article, you will master:
- Different types of flow control statements in Go language.
- How to use these statements in real situations.
- Best practices and common pitfalls.
Let’s get started!
Core Concept
1. for
Loop
for
Loop is the only loop structure in Go language, but it is very flexible and can be used in a variety of scenarios:
Basicfor
Loop
for i := 0; i < 10; i++ { fmt.Println(i) }
This is a traditional `for` loop, initializing variables, setting conditions and incrementing variables.
for
Continuous execution of loop (similar to while
loop)
Go does not have the `while` keyword, but you can use a `for` loop to achieve the same effect:
sum := 1 for sum < 100 { sum += sum }
This loop continues to execute until the condition `sum < 100` is not true. < 100`不成立。
Infinite loop
If the condition is omitted, the `for` loop will execute infinitely:
for { fmt.Println("无限循环") }
This is useful in tasks that need to run continuously (such as servers).
if
Statement
if
Statement`if` statement is used for conditional execution.
Basicif
Statements
if x > 10 { fmt.Println("x大于10") }
if
statement with short statement
A short statement can be executed before the condition:
if x := 5; x < 10 { fmt.Println("x小于10") }
if
and else
You can also use `else` and `else if`:
if x > 10 { fmt.Println("x大于10") } else if x == 10 { fmt.Println("x等于10") } else { fmt.Println("x小于10") }
switch
Statement
switch
StatementThe `switch` statement is a powerful way to handle multiple conditions.
Basicswitch
Statements
switch os := runtime.GOOS; os { case "darwin": fmt.Println("OS X") case "linux": fmt.Println("Linux") default: fmt.Printf("%s.\n", os) }
switch
Execution order of statements
Go evaluates the cases of the `switch` statement from top to bottom, stopping once a match is successful.
Unconditionalswitch
Statement
The unconditional `switch` statement is equivalent to `switch true`:
t := time.Now() switch { case t.Hour() < 12: fmt.Println("上午") case t.Hour() < 18: fmt.Println("下午") default: fmt.Println("晚上") }
defer
Statement
defer
StatementThe `defer` statement defers the execution of a function until its surrounding function returns.
Basicdefer
Statements
func main() { defer fmt.Println("world") fmt.Println("hello") }
Output:
<code>hello world</code>
Stackeddefer
Statements
Delay functions are executed in last-in-first-out (LIFO) order:
func main() { defer fmt.Println("first") defer fmt.Println("second") defer fmt.Println("third") }
Output:
<code>third second first</code>
Practical example
Let’s look at a practical example demonstrating the use of these flow control statements. We will create a simple program that processes a task list and prints its status.
for i := 0; i < 10; i++ { fmt.Println(i) }
Detailed explanation of steps
- **Task structure**: We define a `Task` structure containing `Name` and `Complete` fields.
- **Task List**: We create a slice of `Task` objects.
- **for loop**: We use a `for` loop to iterate over tasks. For each task, we use an `if` statement to check if it has been completed.
- **switch statement**: We use the `switch` statement to check whether today is a weekend or a working day.
- **defer statement**: We use `defer` to print a message after all tasks are processed.
Best Practices
- **Use for loops wisely**: Since Go only has `for` loops, make sure to use them correctly. Avoid infinite loops unless necessary.
- **Keep if statements simple**: Use short statements in `if` conditions to make the code concise and easy to read.
- **Use switch to handle multiple conditions**: When dealing with multiple conditions, a `switch` statement is more readable than multiple `if-else` statements.
- **Use defer for cleanup**: `defer` is great for resource cleanup, such as closing files or releasing locks.
- **Avoid Deep Nesting**: Deeply nested `if` or `for` statements can make the code difficult to read. Consider refactoring into a function.
Conclusion
Flow control statements are essential tools in the Go language. They allow you to control the execution flow of your program. By mastering `for`, `if`, `switch`, and `defer`, you can write more efficient, readable, and maintainable Go code.
I encourage you to try the examples provided in this article and experiment with the concepts on your own.
Call to Action
This article is part of the Go language tutorial series, designed to help you become a more proficient Go developer. If you found this article helpful, be sure to check out previous and upcoming tutorials in this series. Check it out on my blog or Dev.to.
Happy programming! ?
The above is the detailed content of Flow Control Statements in Go. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










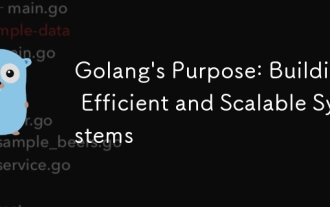
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
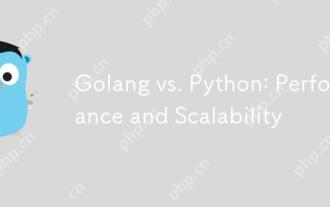
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
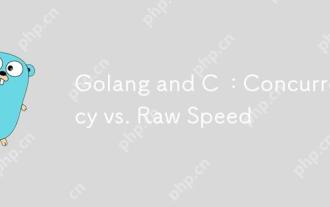
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
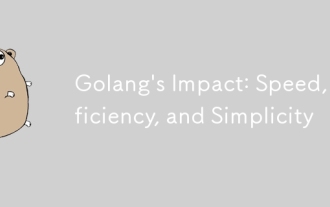
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
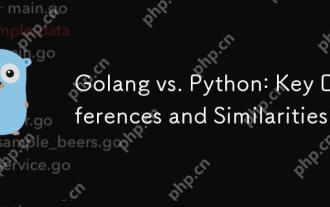
Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
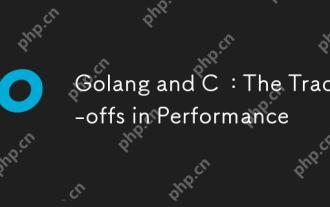
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
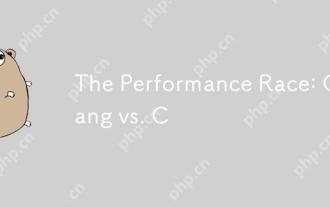
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
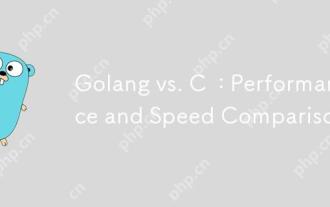
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
