Set vs Array in JavaScript: When to Use Which?
JavaScript provides two powerful data structures for storing collections: Set
and Array
. While both can store multiple values, their unique characteristics make them better suited for different scenarios. Let’s explore when and why to choose one over the other.
-
Unique value by default
Set
The most notable feature is its automatic handling of duplicates.
// 数组允许重复 const arr = [1, 2, 2, 3, 3, 4]; console.log(arr); // [1, 2, 2, 3, 3, 4] // Set 自动删除重复项 const set = new Set([1, 2, 2, 3, 3, 4]); console.log([...set]); // [1, 2, 3, 4] // 使用 Set 从数组中删除重复项 const uniqueArray = [...new Set(arr)]; console.log(uniqueArray); // [1, 2, 3, 4]
-
Element checking performance
Set
provides faster lookup times for checking if an element exists.
const largeArray = Array.from({ length: 1000000 }, (_, i) => i); const largeSet = new Set(largeArray); // 数组查找 console.time('Array includes'); console.log(largeArray.includes(999999)); console.timeEnd('Array includes'); // Set 查找 console.time('Set has'); console.log(largeSet.has(999999)); console.timeEnd('Set has'); // Set 明显更快,因为它内部使用哈希表
-
Available methods and operations
Array
provides more built-in methods for data manipulation, while Set
focuses on uniqueness management.
// 数组方法 const arr = [1, 2, 3, 4, 5]; arr.push(6); // 添加到末尾 arr.pop(); // 从末尾移除 arr.unshift(0); // 添加到开头 arr.shift(); // 从开头移除 arr.splice(2, 1, 'new'); // 替换元素 arr.slice(1, 3); // 提取部分 arr.map(x => x * 2); // 转换元素 arr.filter(x => x > 2); // 过滤元素 arr.reduce((a, b) => a + b); // 归约为单个值 // Set 方法 const set = new Set([1, 2, 3, 4, 5]); set.add(6); // 添加值 set.delete(6); // 删除值 set.has(5); // 检查是否存在 set.clear(); // 删除所有值
-
Sequential and indexed access
Array
maintains insertion order and provides index-based access, while Set
only maintains insertion order.
// 数组索引访问 const arr = ['a', 'b', 'c']; console.log(arr[0]); // 'a' console.log(arr[1]); // 'b' arr[1] = 'x'; // 直接修改 // Set 没有索引访问 const set = new Set(['a', 'b', 'c']); console.log([...set][0]); // 需要先转换为数组 // 不允许直接修改索引
-
Memory usage
Set
typically uses more memory than Array
but provides faster lookups.
// 内存比较(粗略示例) const numbers = Array.from({ length: 1000 }, (_, i) => i); // 数组内存 const arr = [...numbers]; console.log(process.memoryUsage().heapUsed); // Set 内存 const set = new Set(numbers); console.log(process.memoryUsage().heapUsed); // 由于哈希表结构,Set 通常使用更多内存
-
Common use cases
When to use arrays:
// 1. 当顺序和索引访问很重要时 const playlist = ['song1.mp3', 'song2.mp3', 'song3.mp3']; const currentTrack = playlist[currentIndex]; // 2. 当您需要数组方法时 const numbers = [1, 2, 3, 4, 5]; const doubled = numbers.map(x => x * 2); const sum = numbers.reduce((a, b) => a + b, 0); // 3. 当重复值可以接受或需要时 const votes = ['yes', 'no', 'yes', 'yes', 'no']; const yesVotes = votes.filter(vote => vote === 'yes').length;
When to use Set:
// 1. 当跟踪唯一值时 const uniqueVisitors = new Set(); function logVisitor(userId) { uniqueVisitors.add(userId); console.log(`Total unique visitors: ${uniqueVisitors.size}`); } // 2. 用于快速查找操作 const allowedUsers = new Set(['user1', 'user2', 'user3']); function checkAccess(userId) { return allowedUsers.has(userId); } // 3. 用于删除重复项 function getUniqueHashtags(posts) { const uniqueTags = new Set(); posts.forEach(post => { post.hashtags.forEach(tag => uniqueTags.add(tag)); }); return [...uniqueTags]; }
Convert between Set and Array
You can easily switch between Set
and Array
as needed.
// 数组到 Set const arr = [1, 2, 2, 3, 3, 4]; const set = new Set(arr); // Set 到数组 - 三种方法 const back1 = [...set]; const back2 = Array.from(set); const back3 = Array.from(set.values()); // 用于数组去重 const deduped = [...new Set(arr)];
Conclusion
Choose Array
when you need:
- Index-based access
- Extensive array methods (map, reduce, filter, etc.)
- Duplicate value
- Memory efficiency
- Traditional iteration mode
Choose Set
when you need:
- Unique values only
- Quick search operation
- Simple add/delete operation
- Keep a list of unique items
- Quickly remove duplicates
Remember that you can switch between the two types at any time, so choose the one that best suits your current needs.
The above is the detailed content of Set vs Array in JavaScript: When to Use Which?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


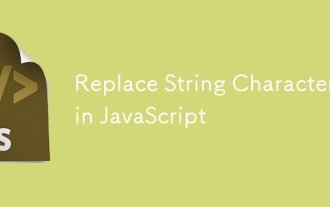
Detailed explanation of JavaScript string replacement method and FAQ This article will explore two ways to replace string characters in JavaScript: internal JavaScript code and internal HTML for web pages. Replace string inside JavaScript code The most direct way is to use the replace() method: str = str.replace("find","replace"); This method replaces only the first match. To replace all matches, use a regular expression and add the global flag g: str = str.replace(/fi
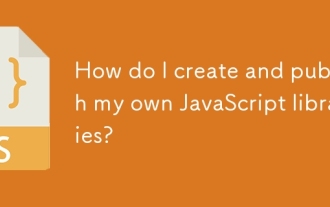
Article discusses creating, publishing, and maintaining JavaScript libraries, focusing on planning, development, testing, documentation, and promotion strategies.
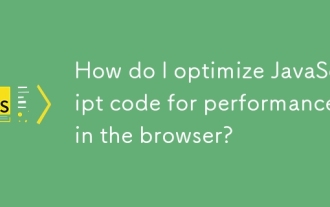
The article discusses strategies for optimizing JavaScript performance in browsers, focusing on reducing execution time and minimizing impact on page load speed.
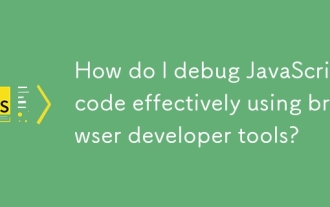
The article discusses effective JavaScript debugging using browser developer tools, focusing on setting breakpoints, using the console, and analyzing performance.
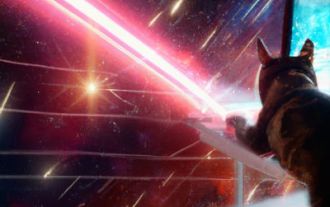
This article outlines ten simple steps to significantly boost your script's performance. These techniques are straightforward and applicable to all skill levels. Stay Updated: Utilize a package manager like NPM with a bundler such as Vite to ensure
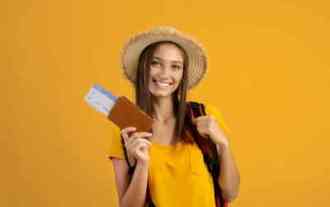
Sequelize is a promise-based Node.js ORM. It can be used with PostgreSQL, MySQL, MariaDB, SQLite, and MSSQL. In this tutorial, we will be implementing authentication for users of a web app. And we will use Passport, the popular authentication middlew
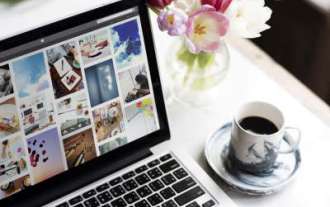
This article will guide you to create a simple picture carousel using the jQuery library. We will use the bxSlider library, which is built on jQuery and provides many configuration options to set up the carousel. Nowadays, picture carousel has become a must-have feature on the website - one picture is better than a thousand words! After deciding to use the picture carousel, the next question is how to create it. First, you need to collect high-quality, high-resolution pictures. Next, you need to create a picture carousel using HTML and some JavaScript code. There are many libraries on the web that can help you create carousels in different ways. We will use the open source bxSlider library. The bxSlider library supports responsive design, so the carousel built with this library can be adapted to any
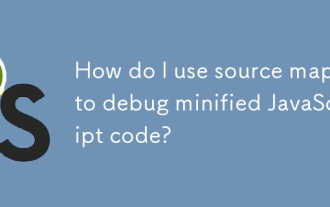
The article explains how to use source maps to debug minified JavaScript by mapping it back to the original code. It discusses enabling source maps, setting breakpoints, and using tools like Chrome DevTools and Webpack.
