AIOMQL
Introduction
Theaiomql
package is an advanced algorithmic trading framework that enhances and extends the functionality of the MetaTrader5 Python integrated library (metatrader5
). Its purpose is to simplify the process of building custom trading bots while providing a rich set of tools and abstractions specifically for algorithmic trading. aiomql
Designed with flexibility and adaptability in mind, the framework can meet the needs of a variety of users, from those looking for a lightweight asynchronous alternative to MetaTrader5 Python integration, to developers building complex multi-strategy trading robots.
Demand
This tutorial introduces the fourth version of the aiomql
framework, which offers significant improvements over previous versions in terms of speed, efficiency, and design. A key highlight of this release is the inclusion of a powerful backtesting feature, allowing users to test trading strategies using historical data before deploying them live.
While familiarity with aiomql
earlier versions of the framework can enhance your understanding, it is not a prerequisite. This tutorial is designed to be easily understood even by beginners who have never used the framework. However, fully understanding and applying the concepts covered requires a working knowledge of Python and a basic understanding of algorithmic trading.
While this article is intended primarily as an introduction to the framework rather than a step-by-step tutorial, it does contain code snippets that require Python 3.11. If you encounter compatibility issues in NumPy, you can resolve them by installing a supported version using the following command:
pip install aiomql pip uninstall numpy pip install 'numpy<v2'
Organization and structure of `aiomql`
aiomql
The framework is divided into three main sub-packages (core, contrib and lib), as well as a top-level module utils.py
that provides utility functions used throughout the framework. For convenience, all classes, functions, constants and other components are directly accessible from the top level.
MetaTrader class
As mentioned earlier, the aiomql
library is the asynchronous counterpart of the metatrader5
package. This functionality is implemented through the MetaTrader class, which is a basic component of the framework. This class replicates the functionality of the metatrader5
library, providing its functions, constants, and specialized types as methods and properties.
Main Features
metatrader5
Functions in the package are accessed as asynchronous instance methods in the MetaTrader class, retaining their original names. This design ensures seamless integration for users familiar with the original library, while taking advantage of the benefits of asynchronous programming.
The MetaTrader class is designed as an asynchronous context manager, simplifying the management of the MetaTrader terminal. After entering the context, it automatically initializes the terminal and performs login operations. On exit, it ensures proper cleanup and disconnection of resources even if an error occurs. This structure provides a concise and efficient method of lifecycle management, making it particularly suitable for asynchronous workflows.
The non-async versions of these functions are still accessible, but are prefixed with an underscore (_) to indicate that they can be treated as private methods.
MetaBackTester
MetaBackTester is a subclass of MetaTrader specifically used for backtesting. It uses a BackTestEngine instance, which can be provided during initialization or retrieved from the configuration. When config.mode
is set to "backtest", this class automatically replaces MetaTrader in applications that rely on a terminal connection, ensuring seamless integration of backtesting functionality.
Base and _Base
TheBase
and _Base
classes are the basic components that form the other classes in the framework, providing basic utilities such as data validation, serialization and seamless access to MetaTrader or MetaBackTester depending on whether the system is in live trading or backtesting mode Example. Although these classes are rarely used directly, they ensure the stability and reliability of the overall system by serving as the backbone for more specialized functionality.
Base
The Base class provides powerful data processing functions, focusing on validation and serialization. During instantiation, only properties that are explicitly annotated in the class body or inherited from a parent class can be assigned values, and these values must strictly conform to their defined types, thus ensuring structured and type-safe data management.
Important properties and methods
-
exclude
(set): A set of property names to exclude when accessing the property or generating a dictionary representation of the object. -
include
(set): A collection of attribute names to be explicitly included. This collection overrides theexclude
collection if both collections are provided. -
dict
: An attribute that returns all attributes as a dictionary, applying the filters specified in theexclude
andinclude
collections. -
set_attributes(**kwargs)
: A method to dynamically set instance properties. It validates the inputs and ensures they have the correct type. -
get_dict(exclude: set = None, include: set = None)
: A method that returns the instance's properties as a dictionary, allowingexclude
andinclude
filters to be specified. If both parameters are supplied, theinclude
parameter takes precedence over theexclude
parameter.
_Base
This class is a subclass of Base
with additional properties and functionality specifically for connecting to terminals.
Important properties and methods
-
config
: Represents a configuration instance. This property contains all necessary configuration settings required for the class to run. -
mt5
(MetaTrader | MetaBackTester): Provides a MetaTrader or MetaBackTester instance, determined by theconfig
property of themode
object. The type of instance depends on whether the system is in live trading mode (MetaTrader) or backtesting mode (MetaBackTester).
Constant
The MetaTrader5 library organizes its large number of constants into specialized enumerations, improving clarity and functionality for developers. These enumerations represent various trading operations, order types, timeframes, and more, simplifying their use by grouping related constants under intuitive categories. Each enumeration provides properties and, where applicable, additional methods or properties that enable functionality such as converting, reversing, or deriving values. This organization reduces errors, improves code readability, and makes constants easier to manage, especially in complex trading applications.
Enumerations such as TradeAction
, OrderFilling
, and TimeFrame
encapsulate constants as integer values while providing a Python-style string representation for improved usability. Some enumerations (like TimeFrame
) contain advanced methods for converting seconds to predefined intervals or accessing all possible values. By systematically organizing these constants, the MetaTrader5 library ensures a more efficient and developer-friendly experience, simplifying interaction with trading operations and platform data.
Model
The models module provides a well-organized set of data processing classes built on the structure of the MetaTrader5 library. All classes inherit from the Base class, which adds functionality such as data validation, serialization, and filtering to simplify their use. These classes represent various trading-related entities such as OrderSendResult
, SymbolInfo
and TradeRequest
, allowing developers to efficiently interact with MetaTrader5’s trading environment.
Each class encapsulates specific properties and characteristics related to its corresponding transaction entity, ensuring robust and structured data management. For example, SymbolInfo
provides detailed information about a financial instrument, while TradeRequest
allows the creation and submission of trading operations. This modular design enhances readability, simplifies complex trading workflows, and ensures data consistency and ease of use for developers leveraging MetaTrader5 in their applications.
TaskQueue
TheTaskQueue class efficiently manages background tasks of a bot or backtester using asyncio.PriorityQueue
instances for concurrent execution. It runs continuously in the background, allowing seamless integration with other components such as the Trader class that uses it to record post-trade execution data. Tasks are added to the queue via the add
method, but they must first be wrapped in a QueueItem
that contains task metadata such as parameters, priority, and completion requirements.
With configurable number of workers, timeout settings and task priorities, TaskQueue ensures robust task management and error handling. It supports limited or infinite execution modes and can gracefully clean up remaining tasks before terminating based on priority. This design enhances workflow by offloading and managing background operations asynchronously, ensuring smooth and reliable functionality of complex trading or testing environments.
Account
TheAccount
class is a singleton used to manage broker account details, ensuring that only one instance exists during the lifetime of the application. It inherits from Base
and _AccountInfo
and provides a structured and user-friendly interface for accessing account-specific information. This architecture ensures consistency and prevents duplicate instances, centralizing account management within the application.
As an AsyncContextManager, the Account
class simplifies resource management by automating the initialization and cleanup process. When entering its context, it initializes the MetaTrader terminal and logs in to the specified account. On exit, it ensures that resources are properly closed and cleaned up. This simplified approach enhances the reliability of resource processing, makes account management more intuitive, and reduces the possibility of errors during trading operations.
Important properties and methods
-
connected
(bool): Indicates whether the login was successful and the connection to the terminal is active. Returns True if the account is connected. -
refresh()
: Updates the account instance by retrieving the latest account details from the terminal, ensuring that the account data remains up to date.
Risk and Asset Management
TheRAM
class is responsible for managing risk assessment and risk management during trading activities. It has attributes such as risk_to_reward
, fixed_amount
, min_amount
, max_amount
and risk
, allowing traders to effectively define and control their risk exposure. These properties provide the flexibility to set risk parameters, minimum and maximum risk amounts, and risk-to-reward ratios for each trade.
This class includes methods such as get_amount
, which calculates the risk amount for each trade based on available margin and risk percentage, and check_losing_positions
and check_open_positions
, which ensure that losses or position amounts remain within a predefined within limits. By integrating these capabilities, the RAM class provides a comprehensive approach to risk management, enhancing decision-making capabilities and promoting safer trading practices.
Important properties and methods
-
risk
(float): The percentage of capital at risk per trade, expressed as an integer (e.g. 1 means 1%). Default is 1. -
risk_to_reward
(float): Risk-reward ratio, indicating the relationship between potential risk and reward. Default is 2. -
fixed_amount
(float): Fixed amount to risk per trade, if set, overrides percentage-based calculations. -
get_amount()
: Calculates and returns the risk amount for each trade based on configured properties such as risk,fixed_amount
,min_amount
andmax_amount
.
RAM
class is best used in the Trader class, which can be used before placing an order.
Candle and Candles
TheCandle
and Candles
classes are custom tools for managing price bars retrieved from the terminal. Candle
class encapsulates the data of a single price bar, ensuring that required properties such as open, high, low and close (OHLC) are present in each instance. It supports Python protocols, including hashing, iteration, and comparison, while providing methods such as keys()
and values()
to manage properties. Additionally, it dynamically adds properties passed during instantiation, allowing flexible customization.
Candles
class acts as a container for multiple Candle objects, arranged in chronological order. It uses pandas DataFrame
to store and manage its data, allowing seamless integration with tools like pandas-ta for advanced technical analysis. This class serves as a powerful basis for developing trading strategies by supporting various Python protocols such as slicing and iteration and extending functionality through specialized properties such as timeframes. Its versatility ensures frequent use in complex analytical workflows.
Properties and methods of Candle class
-
time
(int): candle cycle start time. -
open
(float): The opening price of the candle. -
high
(float): The highest price reached during the candle period. -
low
(float): The lowest price reached during the candle period. -
close
(float): The closing price of the candle. -
tick_volume
(float): Amount of ticks in the candle period. -
real_volume
(float): Actual trading volume during the candle period. -
spread
(float): spread within the candle period. -
Index
(int): A custom property indicating the position of the candle in the sequence. -
set_attributes(**kwargs)
: Dynamically sets the properties of the candle instance from the supplied keyword arguments. -
is_bullish()
: Returns True if the candle is bullish (the closing price is greater than or equal to the opening price), False otherwise. -
is_bearish()
: Returns True if the candle is bearish (the closing price is less than the opening price), False otherwise. -
dict(exclude: set = None, include: set = None)
: Returns a dictionary of candle attributes. You can optionally use theexclude
orinclude
collection to filter attributes.
Properties and methods of Candles class
-
Index
(Series[int]): pandas Series containing the indices of all candles in the object. -
time
(Series[int]): pandas Series containing all candle times in the object. -
open
(Series[float]): pandas Series containing the opening prices of all candles in the object. -
high
(Series[float]): pandas Series containing the highest price of all candles in the object. -
low
(Series[float]): pandas Series containing the lowest price of all candles in the object. -
close
(Series[float]): pandas Series containing the closing prices of all candles in the object. -
tick_volume
(Series[float]): pandas Series containing the tick amounts of all candles in the object. -
real_volume
(Series[float]): pandas Series containing the actual volume of all candles in the object. -
spread
(Series[float]): pandas Series containing all candle spreads in the object. -
Candle
(Type[Candle]): Class used to represent a single candle within the object. -
timeframe
(TimeFrame): The time frame of the candle, calculated from its timestamp. -
data
(DataFrame): pandas DataFrame containing basic data for all candles in the object. -
rename(inplace=True, **kwargs)
: Rename columns of the underlying DataFrame. -
ta
: access the pandas-ta library by performing technical analysis directly on candles via thedata
attribute. -
ta_lib
: Provides access to the ta library for additional technical analysis, use it with functions that require you to pass Series or DataFrame objects as arguments. -
columns
: Returns the column names of the underlying DataFrame.
Ticks and Ticks
TheTicks
and Tick
classes are designed to manage Tick data from the trading terminal, similar to the Candle
class. The Tick
class represents a single Tick, and its properties include bid, ask, last price and volume, ensuring that each instance encapsulates the necessary data for a single Tick event. It supports dynamic attribute management and provides dictionary-like access, making it adaptable and easy to operate in different contexts.
Ticks
class acts as a container for multiple Tick objects, storing data in pandas DataFrame
for efficient manipulation and analysis. It supports iteration, slicing, and various data operations, allowing seamless integration with tools like pandas-ta for technical analysis. This class is essential for processing large amounts of tick data in trading systems, providing a powerful structure for real-time or historical data analysis.
Symbols
The_Symbol_
class provides a powerful structure to encapsulate the data of a financial instrument, supporting calculations and operations required before initiating a transaction. As a subclass of _Base
and SymbolInfo
, it integrates a wide range of properties and methods to efficiently handle financial instruments. This class can be customized for different instruments from different brokers, ensuring adaptability across trading platforms.
The Symbol
class simplifies complex trading tasks with utility methods for accessing current Tick data, managing market depth, and performing currency conversions. It provides the functionality to initialize symbols, verify volumes, and retrieve historical rates or ticks, making it an important part of developing a trading strategy. Its design ensures that developers can extend and customize its behavior to meet specific trading requirements.
Important properties and methods
TheSymbol
class is a feature-rich class. For the purposes of this article, I've only touched on some of the important ones that you're most likely to use. tick
and account
are the only properties of the Symbol
class, unlike those defined in the SymbolInfo
parent class.
-
tick
(Tick): Represents the current price Tick of a financial instrument, providing real-time market data. -
account
(Account): Instance of the current trading account associated with the symbol. -
__init__(*, name: str, **kwargs)
: Initialize symbol instance.name
attribute is required and must match the symbol name specified by the broker in the terminal. -
initialize()
: Populates the symbol instance by retrieving the latest price tick and details from the terminal. If successful, theselect
property is set to True and all properties will be populated with server data. -
convert_currency(*, amount: float, from_currency: str, to_currency: str)
: Converts the specified amount from one currency to another. -
amount_in_quote_currency(*, amount: float)
: Converts the amount from the account currency to the quote currency if the account currency is different from the quote currency. This method is particularly useful for performing risk management calculations. -
info_tick
: Retrieve the current price tick of a financial instrument. -
symbol_select(enable: bool = True)
: Adds symbols to or removes them from the MarketWatch window. -
check_volume(volume: float)
: Verify that the specified transaction volume is within the allowed limits and adjust if necessary. -
round_off_volume(volume: float, round_down: bool = False)
: Rounds the volume to the nearest valid step. -
compute_volume
: Calculates the appropriate volume for a trade. -
copy_rates_from_pos(timeframe, start_position=0, count=500)
: Get bars starting from a specific index. -
copy_rates_from(timeframe, date_from, count=500)
: Retrieve historical exchange rates (bars) starting from the specified date. -
copy_ticks_from(date_from, count=100, flags=CopyTicks.ALL)
: Get Tick data starting from the specified date.
Symbol
class is a versatile and feature-rich tool designed to manage financial instruments, providing the necessary methods to retrieve and transform data. While this introduction focuses on key functions such as initialization, currency conversion, and retrieving the latest Tick data, its many other capabilities will be explored in future discussions. This includes integrating it into algorithmic trading systems and demonstrating its suitability for various use cases.
A notable contribution is the ForexSymbol
class, which is a specialized extension of the Symbol
class and is designed specifically for Forex trading. This subclass is designed to handle the unique requirements of currency pair trading, further demonstrating the flexibility and customization potential of the Symbol
class in different trading scenarios.
Order
TheOrder
class simplifies the management of trading orders by combining related properties and methods, making it easier to check, validate and send orders. As a subclass of _Base
and TradeRequest
, it inherits powerful functionality while providing additional convenience. By setting sensible default values for properties such as action
, type_time
and type_filling
, it minimizes code duplication during instantiation, ensuring efficiency and clarity in transaction operations.
This class simplifies core tasks such as retrieving pending orders, checking fund adequacy, and calculating margin, profit and loss. The integration of methods like send()
and check()
ensures a seamless workflow for initiating and validating trading operations, while utilities like calc_margin()
and calc_profit()
facilitate pre-trade analysis. Its design makes it an essential tool for efficient implementation of algorithmic trading strategies.
Important properties and methods of the Order class
-
__init__
: Initialize the order instance using keyword arguments that must be validTradeRequest
attributes. The default value foraction
isDEAL
, fortype_time
isDAY
, and fortype_filling
isFOK
, although they can be customized during instantiation. -
check(**kwargs)
: Verify your order before placing it. Any keyword arguments provided will update the request object. -
send
: Send the order to the terminal for execution. -
calc_margin
: Calculates the margin required to place a trade. -
calc_profit
: Calculate the potential profit of the trade. -
calc_loss
: Calculate the potential loss of a trade. -
request
: This property returns the transaction request object as a dictionary.
Trader
TheTrader
class acts as a utility-rich abstract base class for managing trade orders. It provides basic methods for creating and managing various order types, while handling key processes such as verification, routing and recording of transactions. This class needs to implement place_trade
methods in its subclasses to ensure flexibility in adapting to specific trading strategies.
Key features include ways to set stop loss and take profit levels based on pips or pips, create orders with or without stop loss levels, and calculate the appropriate amount for a trade. This class integrates with properly initialized Symbol instances and optional RAM
instances for risk assessment, allowing for seamless transaction management. It also supports transaction result recording to facilitate tracking and analysis of executed transactions.
Important properties and methods of Trader class
-
modify_order(**kwargs)
: Modify order attributes using the provided keyword parameters. -
modify_ram(**kwargs)
: Updates the RAM (Risk Assessment and Management) instance properties using the supplied keyword arguments. -
check_order
: Verify your order before placing it to make sure it meets the necessary conditions. -
send_order
: Send the order to the broker for execution. -
record_trade(*, result: OrderSendResult, parameters: dict = None, name: str = "")
: Log transaction details to a CSV or JSON file and delegate the task to theconfig.task_queue
instance. Includes trading results and strategy parameters (if provided).
contrib
package comes with two simple traders, ScalpTrader
and SimpleTrader
, where ScalpTrader
is shown below.
Session and Sessions
TheSession
and Sessions
classes provide a powerful framework to manage and execute trading times. Session
represents a time period defined in UTC, with a start time and an end time, and can be specified as an integer or a datetime.time
object. These sessions can trigger predefined actions, such as closing all trades or only profitable/losing positions, or user-specified custom actions. This allows the strategy to have precise control over trading activity during a specific period.
Sessions
class groups multiple Session
instances into a sorted collection, making it easy to manage overlapping or consecutive trading periods. It acts as an asynchronous context manager, continuously monitoring the current time to determine active sessions. If there are no active sessions, the Sessions
instance pauses trading operations until the next scheduled session starts, ensuring compliance with the specified trading window.
By integrating these classes, trading strategies can easily incorporate time-based rules, thereby increasing their accuracy and reliability. This feature is particularly useful in automated systems that require strict adherence to trading hours (such as Forex or stock market trading) or in automated systems that require operations to be aligned with predefined time intervals.
Important properties and methods of Session class
-
start
(datetime.time): The start time of the session in UTC. -
end
(datetime.time): The end time of the session in UTC. -
on_start
(str): Specifies the action to perform when the session starts, such as"close_all"
,"close_win"
,"close_loss"
or a custom action. -
on_end
(str): Specifies the action to be performed when the session ends, similar toon_start
. -
custom_start
(Callable): Optional custom function to be executed when the session starts. -
custom_end
(Callable): Optional custom function to be executed when the session ends. -
name
(str): The name of the session, if not provided, defaults to a combination of start time and end time. -
in_session
: Check if the current time is within the session. -
begin
: Perform the action specified byon_start
orcustom_start
at the beginning of the session. -
close
: Perform the action specified byon_end
orcustom_end
at the end of the session. -
duration
: Returns the duration of the session as a Duration object with hours, minutes, and seconds. -
close_all
: Close all open positions. -
close_win
: Close all profitable positions. -
close_loss
: Close all losing positions. -
action(action: str)
: Perform the specified action, such as closing a position or triggering a custom function. -
until
: Calculates the time in seconds from the current time to the start of the session.
Important properties and methods of the Sessions class
-
sessions
(list[Session]): A list ofSession
objects sorted by their start time. -
current_session
(Session | None): The currently active session (if any). -
find(moment: datetime.time = None)
: Find and return conversations containing the specified time. If no time is provided, the current time is used. -
find_next(moment: datetime.time = None)
: Find and return the next session after the specified time. If no time is provided, it defaults to the current time. -
check
: Monitor the current session. If there is no active session, it will wait until the next session starts. -
config
: Configuration instance associated with the session, determining the mode (live or backtest).
Strategy
TheStrategy
class is the basic framework for developing trading strategies and can be seamlessly integrated with Bot
and Backtester
. As an abstract base class, it is equipped with many features but needs to implement trade
methods in derived classes. This method serves as the core trading logic of the strategy. The Strategy
class acts as an asynchronous context manager and executes the policy using the run_strategy
method, calling the live_strategy
or backtest_strategy
method depending on the operation mode.
With attributes such as symbol
, sessions
and parameters
, this class ensures that the strategy can be customized and adapted to specific financial instruments and trading sessions. The integration of collaborative multi-tasking methods such as sleep
and delay
ensures that strategies remain efficient and in sync with market updates in a live or backtest environment. This makes the Strategy
class a powerful and flexible tool for building complex trading systems.
Properties and methods
-
name
(str): The name of the strategy. If not provided explicitly, defaults to the class name. -
symbol
(Symbol): The financial instrument associated with the strategy. -
parameters
(dict): Dictionary of policy-specific parameters. -
sessions
(Sessions): The trading session in which the strategy is active. -
mt5
(MetaTrader | MetaBackTester): Instance ofMetaTrader
orMetaBackTester
based on mode (live or backtest). -
config
: Configuration settings of the policy. -
running
(bool): Indicates whether the strategy is currently running. -
backtest_controller
(BackTestController): Controller used to manage backtest operations. -
current_session
(Session): The currently active trading session. -
__init__(symbol: Symbol, params: dict = None, sessions: Sessions = None, name: str = "")
: Use the given symbols, parameters and session initialization strategy.
The above is the detailed content of AIOMQL. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










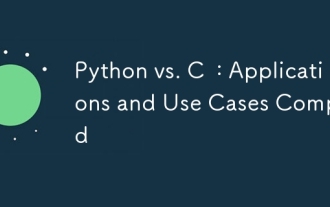
Python is suitable for data science, web development and automation tasks, while C is suitable for system programming, game development and embedded systems. Python is known for its simplicity and powerful ecosystem, while C is known for its high performance and underlying control capabilities.
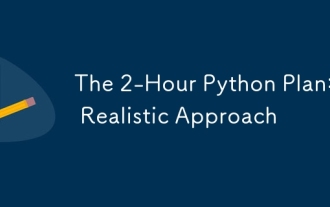
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
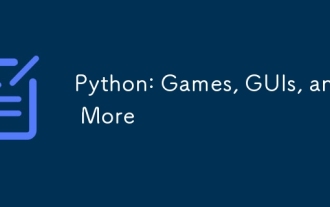
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.
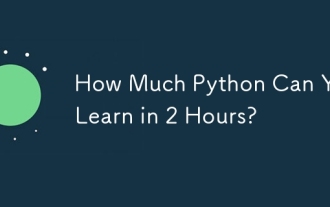
You can learn the basics of Python within two hours. 1. Learn variables and data types, 2. Master control structures such as if statements and loops, 3. Understand the definition and use of functions. These will help you start writing simple Python programs.
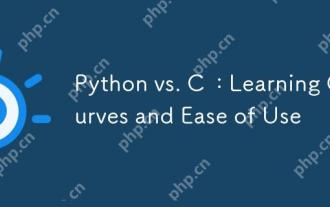
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.
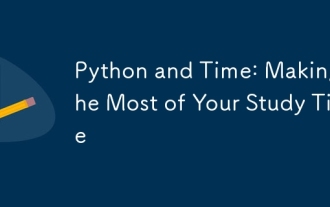
To maximize the efficiency of learning Python in a limited time, you can use Python's datetime, time, and schedule modules. 1. The datetime module is used to record and plan learning time. 2. The time module helps to set study and rest time. 3. The schedule module automatically arranges weekly learning tasks.
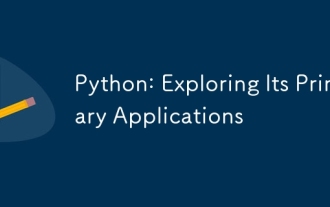
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
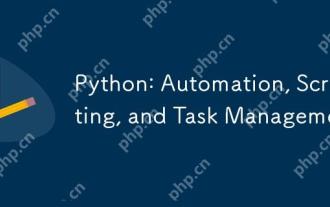
Python excels in automation, scripting, and task management. 1) Automation: File backup is realized through standard libraries such as os and shutil. 2) Script writing: Use the psutil library to monitor system resources. 3) Task management: Use the schedule library to schedule tasks. Python's ease of use and rich library support makes it the preferred tool in these areas.
