How to Build a Simple Web Component from Scratch
This guide demonstrates building a reusable web component: a simple counter. We'll leverage Custom Elements, Shadow DOM, and HTML Templates. The finished counter will feature buttons to increment and decrement a displayed numerical value.
A complete, runnable version of this code is available here.
Prerequisites:
Familiarity with basic JavaScript and a conceptual understanding of the DOM (Document Object Model) are helpful, though not strictly required.
Project Setup:
Create two files: counter.html
(containing the page structure) and counter.js
(housing the custom element definition).
counter.html
(Initial Structure):
<!DOCTYPE html> <html> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width"> <title>Counter Component</title> </head> <body> <script src="counter.js"></script> </body> </html>
Creating the Template (counter.html
- Adding the Template):
We'll define the counter's visual structure using an HTML template:
<template id="x-counter"> <button id="min-btn">-</button> <span id="counter-display">0</span> <button id="plus-btn">+</button> </template>
This template won't render directly; it serves as a blueprint for our custom element.
Defining the Custom Element (counter.js
):
This JavaScript code defines the counter's functionality:
class XCounter extends HTMLElement { constructor() { super(); this.counter = 0; this.elements = {}; } connectedCallback() { const template = document.getElementById("x-counter"); this.attachShadow({ mode: "open" }); this.shadowRoot.appendChild(template.content.cloneNode(true)); this.elements = { plusBtn: this.shadowRoot.querySelector("#plus-btn"), minBtn: this.shadowRoot.querySelector("#min-btn"), counterDisplay: this.shadowRoot.querySelector("#counter-display") }; this.displayCount(); this.elements.plusBtn.onclick = () => this.increment(); this.elements.minBtn.onclick = () => this.decrement(); } increment() { this.counter++; this.displayCount(); } decrement() { this.counter--; this.displayCount(); } displayCount() { this.elements.counterDisplay.textContent = this.counter; } } customElements.define("x-counter", XCounter);
This class extends HTMLElement
. connectedCallback
handles setup when the element is added to the page, including attaching the shadow DOM and event listeners. increment
, decrement
, and displayCount
manage the counter's value and display.
Using the Counter Component (counter.html
- Adding the Custom Element):
To use the counter, simply add <x-counter></x-counter>
to your HTML.
Styling the Component (counter.js
- Adding Styles):
Encapsulate styling within the component using adoptedStyleSheets
:
connectedCallback() { // ... (previous code) ... const sheet = new CSSStyleSheet(); sheet.replaceSync(this.styles()); this.shadowRoot.adoptedStyleSheets = [sheet]; // ... (rest of connectedCallback) ... } styles() { return ` :host { display: block; border: dotted 3px #333; width: fit-content; height: fit-content; padding: 15px; } button { border: solid 1px #333; padding: 10px; min-width: 35px; background: #333; color: #fff; cursor: pointer; } button:hover { background: #222; } span { display: inline-block; padding: 10px; width: 50px; text-align: center; } `; }
This adds basic styling, contained within the shadow DOM.
Conclusion:
This tutorial demonstrates creating a simple, reusable web component. The use of templates, shadow DOM, and custom elements promotes modularity and maintainability in web development. Remember to replace [here](https://www.php.cn/link/2eac42424d12436bdd6a5b8a88480cc3)
with the actual link to your final code.
The above is the detailed content of How to Build a Simple Web Component from Scratch. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


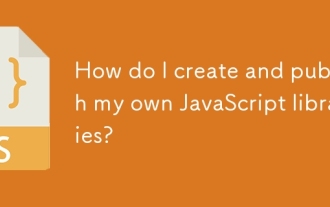
Article discusses creating, publishing, and maintaining JavaScript libraries, focusing on planning, development, testing, documentation, and promotion strategies.
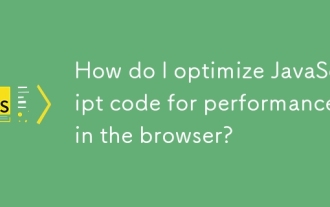
The article discusses strategies for optimizing JavaScript performance in browsers, focusing on reducing execution time and minimizing impact on page load speed.
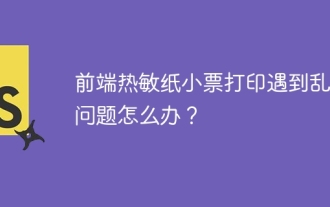
Frequently Asked Questions and Solutions for Front-end Thermal Paper Ticket Printing In Front-end Development, Ticket Printing is a common requirement. However, many developers are implementing...
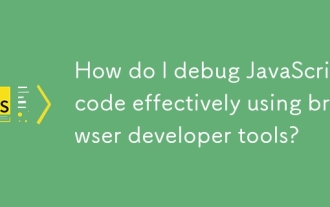
The article discusses effective JavaScript debugging using browser developer tools, focusing on setting breakpoints, using the console, and analyzing performance.
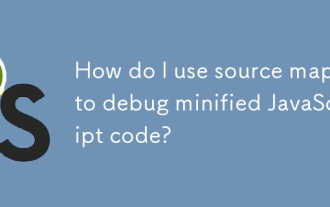
The article explains how to use source maps to debug minified JavaScript by mapping it back to the original code. It discusses enabling source maps, setting breakpoints, and using tools like Chrome DevTools and Webpack.
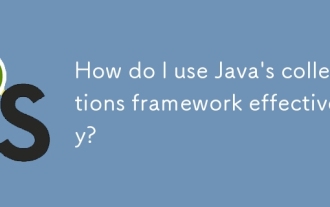
This article explores effective use of Java's Collections Framework. It emphasizes choosing appropriate collections (List, Set, Map, Queue) based on data structure, performance needs, and thread safety. Optimizing collection usage through efficient
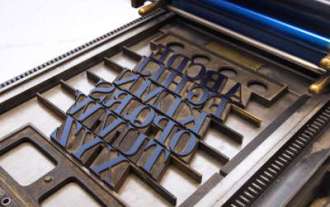
Once you have mastered the entry-level TypeScript tutorial, you should be able to write your own code in an IDE that supports TypeScript and compile it into JavaScript. This tutorial will dive into various data types in TypeScript. JavaScript has seven data types: Null, Undefined, Boolean, Number, String, Symbol (introduced by ES6) and Object. TypeScript defines more types on this basis, and this tutorial will cover all of them in detail. Null data type Like JavaScript, null in TypeScript

This tutorial will explain how to create pie, ring, and bubble charts using Chart.js. Previously, we have learned four chart types of Chart.js: line chart and bar chart (tutorial 2), as well as radar chart and polar region chart (tutorial 3). Create pie and ring charts Pie charts and ring charts are ideal for showing the proportions of a whole that is divided into different parts. For example, a pie chart can be used to show the percentage of male lions, female lions and young lions in a safari, or the percentage of votes that different candidates receive in the election. Pie charts are only suitable for comparing single parameters or datasets. It should be noted that the pie chart cannot draw entities with zero value because the angle of the fan in the pie chart depends on the numerical size of the data point. This means any entity with zero proportion
