


How to Efficiently Remove Duplicate Rows from a Table Without Unique Identifiers?
Efficiently remove duplicate rows without unique identifiers
Removing duplicates can be a challenge when a data table lacks unique row identifiers. This article provides an efficient solution for removing duplicate rows while retaining the first occurrence of the row.
Let’s look at a table with duplicate rows:
col1 | col2 | col3 | col4 | col5 | col6 | col7 |
---|---|---|---|---|---|---|
john | 1 | 1 | 1 | 1 | 1 | 1 |
john | 1 | 1 | 1 | 1 | 1 | 1 |
sally | 2 | 2 | 2 | 2 | 2 | 2 |
sally | 2 | 2 | 2 | 2 | 2 | 2 |
The desired result after removing duplicate rows is:
col1 | col2 | col3 | col4 | col5 | col6 | col7 |
---|---|---|---|---|---|---|
john | 1 | 1 | 1 | 1 | 1 | 1 |
sally | 2 | 2 | 2 | 2 | 2 | 2 |
Solution using CTE and ROW_NUMBER
This method utilizes the common table expression (CTE) and the ROW_NUMBER() function. CTE assigns each row a sequence number (RN) based on a specific order, allowing us to identify and eliminate duplicates.
Here is the SQL query with step-by-step instructions:
WITH CTE AS ( SELECT [col1], [col2], [col3], [col4], [col5], [col6], [col7], RN = ROW_NUMBER() OVER (PARTITION BY col1 ORDER BY col1) -- 为 col1 定义的每个组内分配序列号 FROM dbo.Table1 ) DELETE FROM CTE WHERE RN > 1; -- 删除 RN 大于 1 的行(表示重复项)
Instructions:
- CTE Creation: The WITH statement creates a CTE named CTE that contains the columns of the table and assigns RN values to each row using the ROW_NUMBER() function. The PARTITION BY clause groups the rows based on the col1 column and sorts them within each group to determine the order.
- ROW_NUMBER() function: The ROW_NUMBER() function generates a sequence of integers starting from 1 for each row within each partition defined by the PARTITION BY clause.
- Delete operation: The DELETE statement deletes rows with RN greater than 1 in the CTE, thereby eliminating duplicate rows.
Output:
After executing the query, the updated table will contain:
col1 | col2 | col3 | col4 | col5 | col6 | col7 |
---|---|---|---|---|---|---|
john | 1 | 1 | 1 | 1 | 1 | 1 |
sally | 2 | 2 | 2 | 2 | 2 | 2 |
The above is the detailed content of How to Efficiently Remove Duplicate Rows from a Table Without Unique Identifiers?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


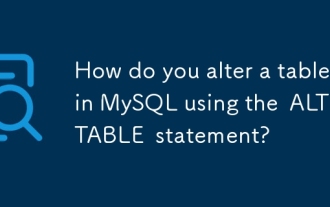
The article discusses using MySQL's ALTER TABLE statement to modify tables, including adding/dropping columns, renaming tables/columns, and changing column data types.
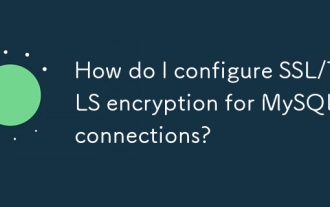
Article discusses configuring SSL/TLS encryption for MySQL, including certificate generation and verification. Main issue is using self-signed certificates' security implications.[Character count: 159]
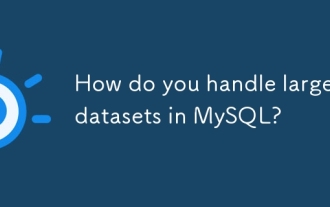
Article discusses strategies for handling large datasets in MySQL, including partitioning, sharding, indexing, and query optimization.
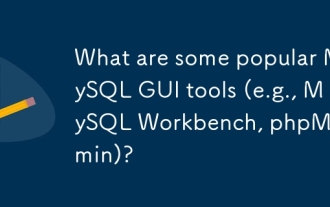
Article discusses popular MySQL GUI tools like MySQL Workbench and phpMyAdmin, comparing their features and suitability for beginners and advanced users.[159 characters]
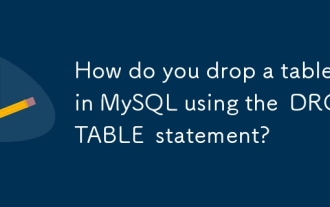
The article discusses dropping tables in MySQL using the DROP TABLE statement, emphasizing precautions and risks. It highlights that the action is irreversible without backups, detailing recovery methods and potential production environment hazards.
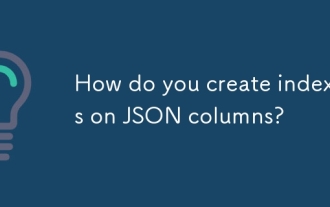
The article discusses creating indexes on JSON columns in various databases like PostgreSQL, MySQL, and MongoDB to enhance query performance. It explains the syntax and benefits of indexing specific JSON paths, and lists supported database systems.
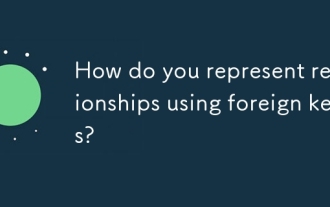
Article discusses using foreign keys to represent relationships in databases, focusing on best practices, data integrity, and common pitfalls to avoid.
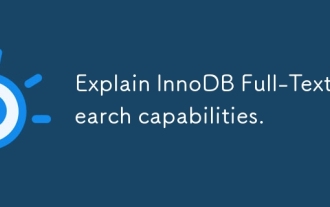
InnoDB's full-text search capabilities are very powerful, which can significantly improve database query efficiency and ability to process large amounts of text data. 1) InnoDB implements full-text search through inverted indexing, supporting basic and advanced search queries. 2) Use MATCH and AGAINST keywords to search, support Boolean mode and phrase search. 3) Optimization methods include using word segmentation technology, periodic rebuilding of indexes and adjusting cache size to improve performance and accuracy.
