How Do Parameterized Queries Enhance Database Security and Efficiency?
Jan 23, 2025 am 04:46 AMParameterized Queries: A Deep Dive
Parameterized queries are essential in database programming, significantly improving both security and performance. Their core function is to isolate query logic from user-supplied data, thus mitigating the threat of SQL injection vulnerabilities.
Understanding Parameterized Queries
A parameterized query is a pre-compiled SQL statement containing placeholders (parameters) that are populated with data only during execution. This separation prevents potentially harmful input from being interpreted as SQL code.
Parameterized Queries with PHP and MySQL (mysqli)
The mysqli extension in PHP offers a robust method for creating parameterized queries:
// Prepare the statement $stmt = $mysqli->prepare("SELECT * FROM users WHERE username = ?"); // Bind the parameter $stmt->bind_param('s', $username); // Set the parameter value $username = 'admin'; // Execute the query $stmt->execute(); // Fetch results $result = $stmt->fetch();
The "?" acts as a placeholder for the username
parameter. bind_param
specifies the parameter's data type ('s' for string). execute
runs the query using the supplied value.
Parameterized Queries with PDO
PDO (PHP Data Objects) provides a more modern, database-agnostic approach:
// Prepare the statement $stmt = $pdo->prepare("SELECT * FROM users WHERE username = :username"); // Bind the parameter $stmt->bindParam(':username', $username); // Set the parameter value $username = 'admin'; // Execute the query $stmt->execute(); // Fetch results $result = $stmt->fetch();
Here, :username
serves as the placeholder. bindParam
links the $username
variable to the placeholder. Note that the specific syntax might differ slightly based on the database driver.
Using parameterized queries is a crucial step towards building secure and efficient database applications, safeguarding against attacks and optimizing query execution.
The above is the detailed content of How Do Parameterized Queries Enhance Database Security and Efficiency?. For more information, please follow other related articles on the PHP Chinese website!

Hot Article

Hot tools Tags

Hot Article

Hot Article Tags

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
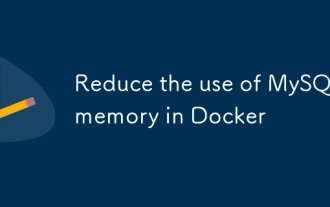
Reduce the use of MySQL memory in Docker
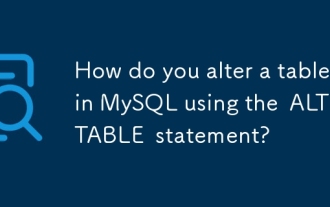
How do you alter a table in MySQL using the ALTER TABLE statement?
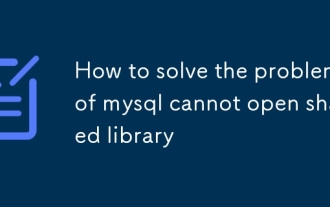
How to solve the problem of mysql cannot open shared library
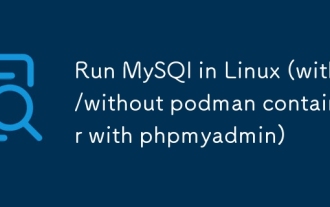
Run MySQl in Linux (with/without podman container with phpmyadmin)
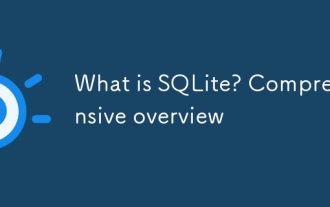
What is SQLite? Comprehensive overview
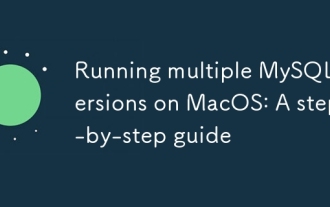
Running multiple MySQL versions on MacOS: A step-by-step guide
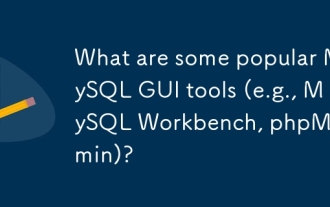
What are some popular MySQL GUI tools (e.g., MySQL Workbench, phpMyAdmin)?
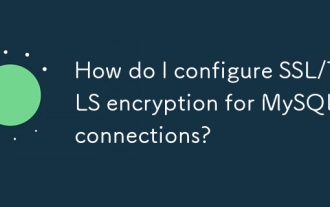
How do I configure SSL/TLS encryption for MySQL connections?
