How to Serialize and Deserialize Objects to/from Files in C#?
Jan 23, 2025 am 11:51 AMC# Object File Saving and Restoration
Saving the object to the computer requires that the object be serializable. This means that the object must be able to be converted into a format that can be written to a file, and then later read back into memory and converted back into an object. The following functions perform serialization and deserialization:
Binary serialization
public static void WriteToBinaryFile<T>(string filePath, T objectToWrite, bool append = false) public static T ReadFromBinaryFile<T>(string filePath)
XML serialization
public static void WriteToXmlFile<T>(string filePath, T objectToWrite, bool append = false) where T : new() public static T ReadFromXmlFile<T>(string filePath) where T : new()
JSON serialization
public static void WriteToJsonFile<T>(string filePath, T objectToWrite, bool append = false) where T : new() public static T ReadFromJsonFile<T>(string filePath) where T : new()
Example
Save the contents of the object1
variable to a file using binary serialization:
WriteToBinaryFile<SomeClass>("C:\someClass.txt", object1);
Read the file contents back into a variable:
SomeClass object1 = ReadFromBinaryFile<SomeClass>("C:\someClass.txt");
The above is the detailed content of How to Serialize and Deserialize Objects to/from Files in C#?. For more information, please follow other related articles on the PHP Chinese website!

Hot Article

Hot tools Tags

Hot Article

Hot Article Tags

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
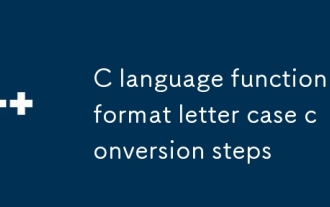
C language function format letter case conversion steps
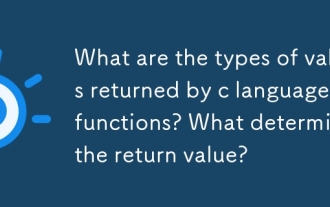
What are the types of values returned by c language functions? What determines the return value?

What are the definitions and calling rules of c language functions and what are the
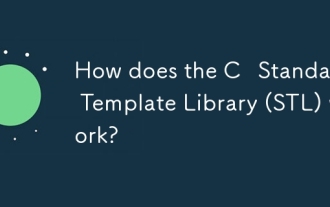
How does the C Standard Template Library (STL) work?

Where is the return value of the c language function stored in memory?
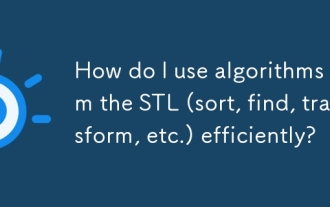
How do I use algorithms from the STL (sort, find, transform, etc.) efficiently?
