


How to Efficiently Select the First Row of Each Group in a Spark DataFrame?
How to efficiently select the first row of each grouping in Spark DataFrame?
Given a DataFrame containing Hour, Category and TotalValue columns, the task is to select the first row of each grouping, where each grouping is defined by a unique combination of Hour and Category.
Use window functions
<code>import org.apache.spark.sql.functions._ import org.apache.spark.sql.expressions.Window val w = Window.partitionBy($"Hour").orderBy($"TotalValue".desc) val dfTop = df.withColumn("rn", row_number.over(w)).where($"rn" === 1).drop("rn")</code>
Use simple SQL to join after aggregation
<code>val dfMax = df.groupBy($"Hour").agg(max($"TotalValue").as("max_value")) val dfTopByJoin = df.join(dfMax, ($"Hour" === dfMax("Hour")) && ($"TotalValue" === dfMax("max_value"))).drop(dfMax("Hour")).drop(dfMax("max_value"))</code>
Use structural sorting
<code>val dfTop = df.select($"Hour", struct($"TotalValue", $"Category").alias("vs")) .groupBy($"Hour") .agg(max("vs").alias("vs")) .select($"Hour", $"vs.Category", $"vs.TotalValue")</code>
Use DataSet API (Spark 1.6, 2.0)
<code>import org.apache.spark.sql.Encoder import org.apache.spark.sql.expressions.Aggregator case class Record(Hour: Integer, Category: String, TotalValue: Double) def firstOfHour[T: Encoder : Aggregator]: TypedColumn[Record, Record] = { aggregator[Record, (Option[Record], Long)](Record(Hour = 0, Category = null, TotalValue = 0.0)) { (buffer, record) => if (record.Hour > buffer._2) buffer else (Some(record), record.Hour) } { (buffer1, buffer2) => if (buffer1._2 > buffer2._2) buffer1 else buffer2 } { x => x._1 match { case Some(r) => r case _ => Record(Hour = 0, Category = "0", TotalValue = 0.0) } } } df.as[Record].groupByKey(_.Hour).agg(firstOfHour[Record]).show</code>
The above is the detailed content of How to Efficiently Select the First Row of Each Group in a Spark DataFrame?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


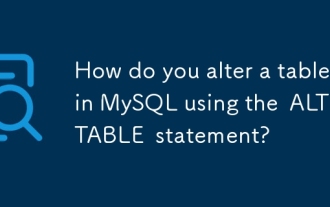
The article discusses using MySQL's ALTER TABLE statement to modify tables, including adding/dropping columns, renaming tables/columns, and changing column data types.
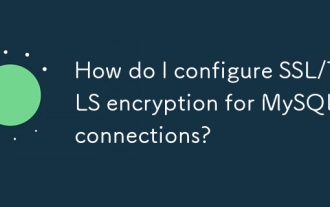
Article discusses configuring SSL/TLS encryption for MySQL, including certificate generation and verification. Main issue is using self-signed certificates' security implications.[Character count: 159]
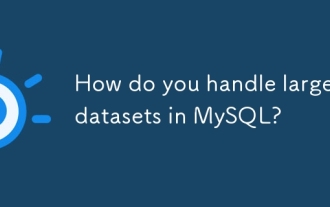
Article discusses strategies for handling large datasets in MySQL, including partitioning, sharding, indexing, and query optimization.
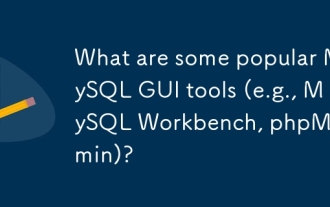
Article discusses popular MySQL GUI tools like MySQL Workbench and phpMyAdmin, comparing their features and suitability for beginners and advanced users.[159 characters]
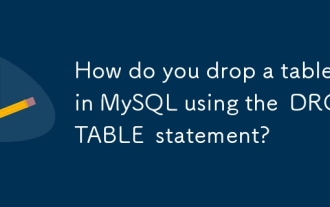
The article discusses dropping tables in MySQL using the DROP TABLE statement, emphasizing precautions and risks. It highlights that the action is irreversible without backups, detailing recovery methods and potential production environment hazards.
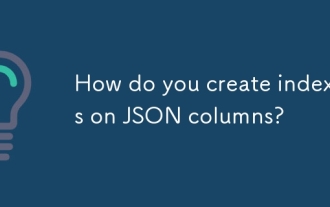
The article discusses creating indexes on JSON columns in various databases like PostgreSQL, MySQL, and MongoDB to enhance query performance. It explains the syntax and benefits of indexing specific JSON paths, and lists supported database systems.
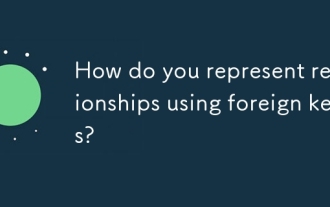
Article discusses using foreign keys to represent relationships in databases, focusing on best practices, data integrity, and common pitfalls to avoid.
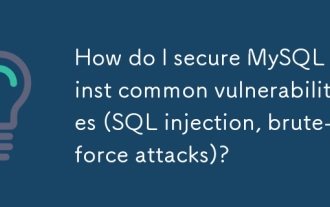
Article discusses securing MySQL against SQL injection and brute-force attacks using prepared statements, input validation, and strong password policies.(159 characters)
