How to Determine Object Type from its String Representation in C#?
Determine object type based on string representation in C#
In C#, a string containing a type name can be converted into the corresponding Type object. However, the method used for this conversion depends on whether the type is defined in the same assembly as the calling code, or in a different assembly.
Use Type.GetType(string)
If the type is defined in the same assembly, you can use the Type.GetType(string)
method and provide the fully qualified type name as a string. For example, to get the int
of the Type
data type, you would use:
Type.GetType("System.Int32");
Use Assembly.GetType(string)
If the type is defined in a different assembly, you also need to provide the assembly name. This can be achieved using the Assembly.GetType(string)
method. First, get a reference to the assembly, then use that reference to get the Type
:
Assembly asm = typeof(SomeKnownType).Assembly; Type type = asm.GetType("Namespace.MyClass");
Namespace and assembly qualification
Make sure to include the type's namespace in the string provided to Type.GetType()
or Assembly.GetType()
. Additionally, for strong-named assemblies, it may be necessary to include the full assembly identity, including version, culture, and public key tags.
Example scenario
Consider the following scenario, where there is a string containing the fully qualified name of a type:
string typeName = "Namespace.MyClass, MyAssembly";
Depending on whether MyAssembly
is referenced by the calling assembly, either Type.GetType()
or Assembly.GetType()
can be used:
// 如果 MyAssembly 被引用 Type type1 = Type.GetType(typeName); // 如果 MyAssembly 未被引用 Assembly asm = typeof(SomeKnownType).Assembly; Type type2 = asm.GetType(typeName);
The above is the detailed content of How to Determine Object Type from its String Representation in C#?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


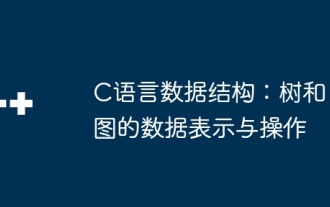
C language data structure: The data representation of the tree and graph is a hierarchical data structure consisting of nodes. Each node contains a data element and a pointer to its child nodes. The binary tree is a special type of tree. Each node has at most two child nodes. The data represents structTreeNode{intdata;structTreeNode*left;structTreeNode*right;}; Operation creates a tree traversal tree (predecision, in-order, and later order) search tree insertion node deletes node graph is a collection of data structures, where elements are vertices, and they can be connected together through edges with right or unrighted data representing neighbors.
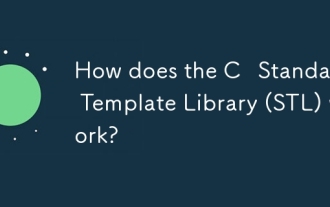
This article explains the C Standard Template Library (STL), focusing on its core components: containers, iterators, algorithms, and functors. It details how these interact to enable generic programming, improving code efficiency and readability t
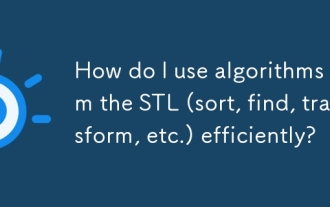
This article details efficient STL algorithm usage in C . It emphasizes data structure choice (vectors vs. lists), algorithm complexity analysis (e.g., std::sort vs. std::partial_sort), iterator usage, and parallel execution. Common pitfalls like
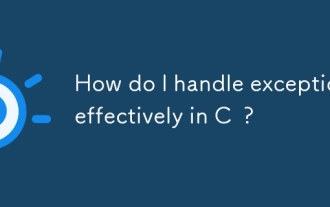
This article details effective exception handling in C , covering try, catch, and throw mechanics. It emphasizes best practices like RAII, avoiding unnecessary catch blocks, and logging exceptions for robust code. The article also addresses perf
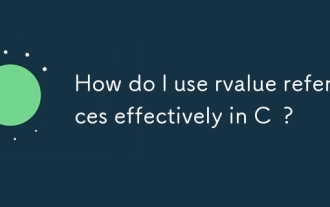
Article discusses effective use of rvalue references in C for move semantics, perfect forwarding, and resource management, highlighting best practices and performance improvements.(159 characters)
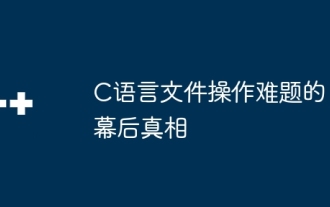
The truth about file operation problems: file opening failed: insufficient permissions, wrong paths, and file occupied. Data writing failed: the buffer is full, the file is not writable, and the disk space is insufficient. Other FAQs: slow file traversal, incorrect text file encoding, and binary file reading errors.
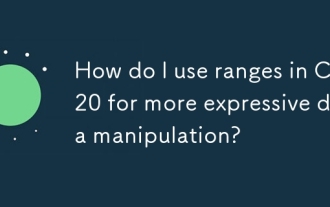
C 20 ranges enhance data manipulation with expressiveness, composability, and efficiency. They simplify complex transformations and integrate into existing codebases for better performance and maintainability.
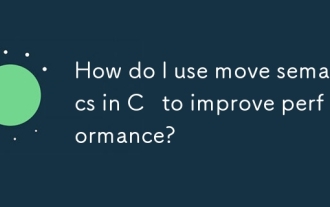
The article discusses using move semantics in C to enhance performance by avoiding unnecessary copying. It covers implementing move constructors and assignment operators, using std::move, and identifies key scenarios and pitfalls for effective appl
