How Can I Efficiently Fill Missing Dates in a MySQL Date Range?
MySQL: Efficiently Handling Missing Dates in a Date Range
This guide demonstrates a highly efficient method for filling gaps in date sequences within your MySQL database. The core technique utilizes a NUMBERS table—a simple table containing a series of integers—to generate the missing dates. By joining this NUMBERS table with your data table, we can create a complete date range, even where data points are absent. Existing scores are preserved; missing scores are represented by a default value (e.g., 0).
Creating and Populating the NUMBERS Table:
First, create a NUMBERS table (if one doesn't already exist):
CREATE TABLE IF NOT EXISTS numbers (id INT UNSIGNED AUTO_INCREMENT PRIMARY KEY);
Next, populate it with a sufficient number of integers. The number of rows should be at least as large as the maximum number of days in your date range. A simple (though potentially slow for very large ranges) method is:
INSERT INTO numbers (id) VALUES (NULL); -- Repeat as needed to fill the desired range
(Note: For very large ranges, more efficient methods for populating the NUMBERS table exist, such as using stored procedures or external scripts.)
Generating the Complete Date Range:
The following query uses the NUMBERS table to generate a sequence of dates and retrieves corresponding scores, filling in missing dates with a default score of 0:
SELECT DATE_ADD('2010-06-06', INTERVAL n.id - 1 DAY) AS timestamp, COALESCE(t.score, 0) AS score -- Replace 't.score' with your actual score column FROM numbers n LEFT JOIN your_table t ON DATE(t.date_column) = DATE_ADD('2010-06-06', INTERVAL n.id - 1 DAY) -- Replace 'your_table' and 'date_column' WHERE DATE_ADD('2010-06-06', INTERVAL n.id - 1 DAY) <= '2010-06-15'; -- Adjust start and end dates as needed
Remember to replace your_table
, date_column
, and score
with your actual table and column names. Adjust the start and end dates in the DATE_ADD
function to cover your desired range. The COALESCE
function handles missing scores gracefully. You can adjust the date formatting using the DATE_FORMAT
function if necessary.
This approach offers a scalable and efficient solution for managing missing dates in your MySQL data. Consider optimizing the NUMBERS table population for very large date ranges.
The above is the detailed content of How Can I Efficiently Fill Missing Dates in a MySQL Date Range?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










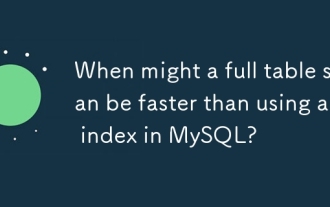
Full table scanning may be faster in MySQL than using indexes. Specific cases include: 1) the data volume is small; 2) when the query returns a large amount of data; 3) when the index column is not highly selective; 4) when the complex query. By analyzing query plans, optimizing indexes, avoiding over-index and regularly maintaining tables, you can make the best choices in practical applications.
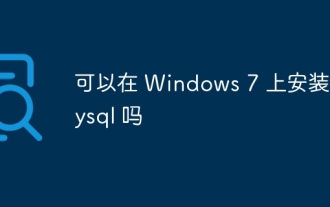
Yes, MySQL can be installed on Windows 7, and although Microsoft has stopped supporting Windows 7, MySQL is still compatible with it. However, the following points should be noted during the installation process: Download the MySQL installer for Windows. Select the appropriate version of MySQL (community or enterprise). Select the appropriate installation directory and character set during the installation process. Set the root user password and keep it properly. Connect to the database for testing. Note the compatibility and security issues on Windows 7, and it is recommended to upgrade to a supported operating system.
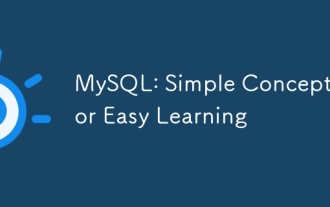
MySQL is an open source relational database management system. 1) Create database and tables: Use the CREATEDATABASE and CREATETABLE commands. 2) Basic operations: INSERT, UPDATE, DELETE and SELECT. 3) Advanced operations: JOIN, subquery and transaction processing. 4) Debugging skills: Check syntax, data type and permissions. 5) Optimization suggestions: Use indexes, avoid SELECT* and use transactions.
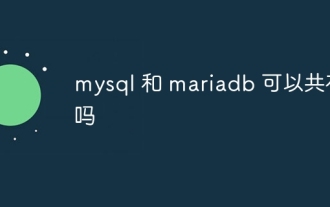
MySQL and MariaDB can coexist, but need to be configured with caution. The key is to allocate different port numbers and data directories to each database, and adjust parameters such as memory allocation and cache size. Connection pooling, application configuration, and version differences also need to be considered and need to be carefully tested and planned to avoid pitfalls. Running two databases simultaneously can cause performance problems in situations where resources are limited.
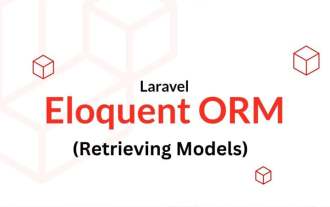
LaravelEloquent Model Retrieval: Easily obtaining database data EloquentORM provides a concise and easy-to-understand way to operate the database. This article will introduce various Eloquent model search techniques in detail to help you obtain data from the database efficiently. 1. Get all records. Use the all() method to get all records in the database table: useApp\Models\Post;$posts=Post::all(); This will return a collection. You can access data using foreach loop or other collection methods: foreach($postsas$post){echo$post->
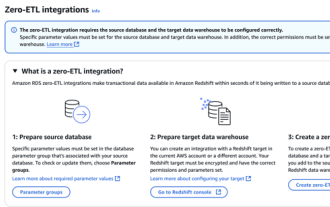
Data Integration Simplification: AmazonRDSMySQL and Redshift's zero ETL integration Efficient data integration is at the heart of a data-driven organization. Traditional ETL (extract, convert, load) processes are complex and time-consuming, especially when integrating databases (such as AmazonRDSMySQL) with data warehouses (such as Redshift). However, AWS provides zero ETL integration solutions that have completely changed this situation, providing a simplified, near-real-time solution for data migration from RDSMySQL to Redshift. This article will dive into RDSMySQL zero ETL integration with Redshift, explaining how it works and the advantages it brings to data engineers and developers.
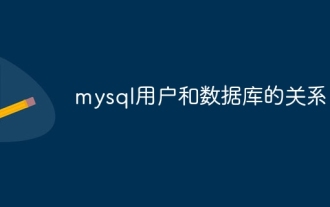
In MySQL database, the relationship between the user and the database is defined by permissions and tables. The user has a username and password to access the database. Permissions are granted through the GRANT command, while the table is created by the CREATE TABLE command. To establish a relationship between a user and a database, you need to create a database, create a user, and then grant permissions.
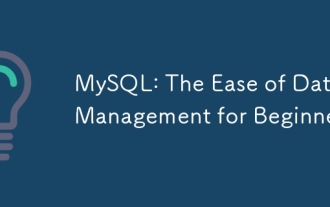
MySQL is suitable for beginners because it is simple to install, powerful and easy to manage data. 1. Simple installation and configuration, suitable for a variety of operating systems. 2. Support basic operations such as creating databases and tables, inserting, querying, updating and deleting data. 3. Provide advanced functions such as JOIN operations and subqueries. 4. Performance can be improved through indexing, query optimization and table partitioning. 5. Support backup, recovery and security measures to ensure data security and consistency.
