How Can Parameterized SQL Prevent SQL Injection Attacks?
Defend SQL injection attacks: use parameterized SQL to process user input
Preventing SQL injection is critical when using user input to dynamically create SQL statements. The best approach is to use parameterized SQL instead of concatenating user-supplied data directly into the query string.
How parameterized SQL works
Parameterized SQL uses special placeholders in SQL statements, such as represented by the @ symbol (e.g., @variableName). These placeholders serve as markers for user-supplied values, which are subsequently added to the command object via the Parameters collection.
Advantages of parameterized SQL
Choosing parameterized SQL has the following advantages:
- Enhanced Security: By separating user input from the query string, it effectively prevents SQL injection attacks because the SQL interpreter recognizes placeholders as different data, not the query part of itself.
- Simplified code: Compared to manual string concatenation, parameterized SQL eliminates the need to escape single quotes or format date literals, simplifying the development process.
- Improved stability: Parameterized SQL ensures that applications are not affected by unexpected characters in user input, such as single quotes or special symbols.
Implementation of parameterized SQL
In C#, use the AddWithValue method to assign a value to the placeholder. For example, consider the following scenario:
var sql = "INSERT INTO myTable (myField1, myField2) " + "VALUES (@someValue, @someOtherValue);"; using (var cmd = new SqlCommand(sql, myDbConnection)) { cmd.Parameters.AddWithValue("@someValue", someVariable); cmd.Parameters.AddWithValue("@someOtherValue", someTextBox.Text); cmd.ExecuteNonQuery(); }
In VB.NET, the process is similar:
Dim sql = "INSERT INTO myTable (myField1, myField2) " & "VALUES (@someValue, @someOtherValue);"; Dim cmd As New SqlCommand(sql, myDbConnection) cmd.Parameters.AddWithValue("@someValue", someVariable) cmd.Parameters.AddWithValue("@someOtherValue", someTextBox.Text) cmd.ExecuteNonQuery()
Conclusion
Parameterized SQL is a powerful tool to resist SQL injection, simplify development and enhance stability. By employing this technology, you can confidently leverage user input into SQL statements without compromising data security.
The above is the detailed content of How Can Parameterized SQL Prevent SQL Injection Attacks?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


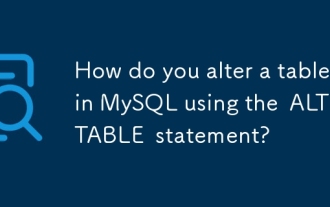
The article discusses using MySQL's ALTER TABLE statement to modify tables, including adding/dropping columns, renaming tables/columns, and changing column data types.
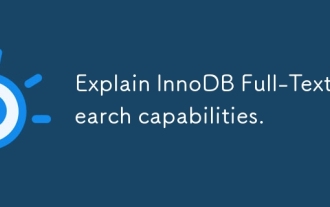
InnoDB's full-text search capabilities are very powerful, which can significantly improve database query efficiency and ability to process large amounts of text data. 1) InnoDB implements full-text search through inverted indexing, supporting basic and advanced search queries. 2) Use MATCH and AGAINST keywords to search, support Boolean mode and phrase search. 3) Optimization methods include using word segmentation technology, periodic rebuilding of indexes and adjusting cache size to improve performance and accuracy.
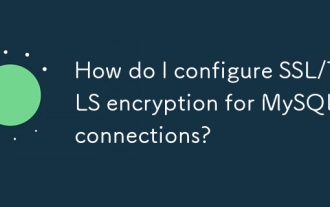
Article discusses configuring SSL/TLS encryption for MySQL, including certificate generation and verification. Main issue is using self-signed certificates' security implications.[Character count: 159]
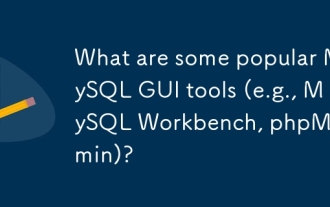
Article discusses popular MySQL GUI tools like MySQL Workbench and phpMyAdmin, comparing their features and suitability for beginners and advanced users.[159 characters]
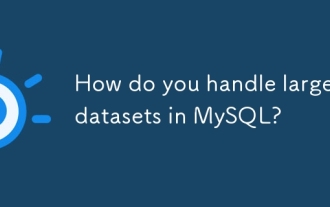
Article discusses strategies for handling large datasets in MySQL, including partitioning, sharding, indexing, and query optimization.
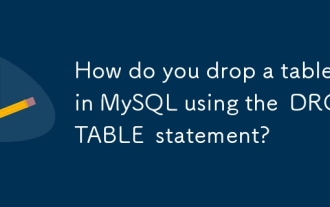
The article discusses dropping tables in MySQL using the DROP TABLE statement, emphasizing precautions and risks. It highlights that the action is irreversible without backups, detailing recovery methods and potential production environment hazards.
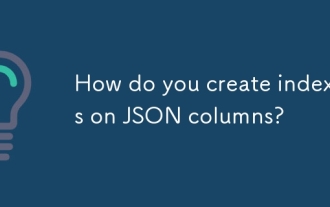
The article discusses creating indexes on JSON columns in various databases like PostgreSQL, MySQL, and MongoDB to enhance query performance. It explains the syntax and benefits of indexing specific JSON paths, and lists supported database systems.
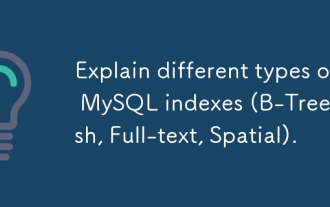
MySQL supports four index types: B-Tree, Hash, Full-text, and Spatial. 1.B-Tree index is suitable for equal value search, range query and sorting. 2. Hash index is suitable for equal value searches, but does not support range query and sorting. 3. Full-text index is used for full-text search and is suitable for processing large amounts of text data. 4. Spatial index is used for geospatial data query and is suitable for GIS applications.
