How to Properly Rethrow Exceptions in C# and Preserve the Stack Trace?
The correct exception rethrowing method in C#
When handling exceptions in C#, correct rethrow syntax is crucial to ensure the accuracy of stack traces. While some rethrowing techniques look similar, subtle differences can affect the debugging process.
Method 1:
try { // ...代码块... } catch (Exception ex) { // ...处理代码... throw; }
This method simply rethrows the caught exception without any modification. Typically, it is preferred over method two because it preserves the original stack trace, allowing developers to accurately trace the exception to its source.
Method 2:
try { // ...代码块... } catch (Exception ex) { // ...处理代码... throw ex; }
While this method also rethrows exceptions, it may alter the stack trace. By explicitly throwing a caught exception, it creates a new stack frame, obscuring the original source of the exception, making it more difficult to pinpoint the root cause.
Use ExceptionDispatchInfo to rethrow exceptions from other sources:
If you need to rethrow an exception from a different context (such as an aggregate exception or a separate thread), you should use the ExceptionDispatchInfo
class. This class captures the necessary information of the original exception and allows it to be rethrown in a new context while retaining its original stack trace.
Example:
try { methodInfo.Invoke(...); } catch (System.Reflection.TargetInvocationException e) { System.Runtime.ExceptionServices.ExceptionDispatchInfo.Capture(e.InnerException).Throw(); throw; // 告知编译器代码流不会离开此代码块 }
By using the ExceptionDispatchInfo
class, the context and stack trace of the original exception are preserved, allowing developers to accurately trace errors to their source.
The above is the detailed content of How to Properly Rethrow Exceptions in C# and Preserve the Stack Trace?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


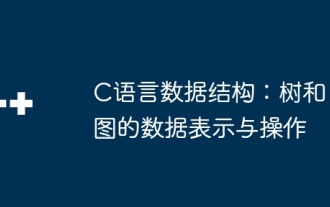
C language data structure: The data representation of the tree and graph is a hierarchical data structure consisting of nodes. Each node contains a data element and a pointer to its child nodes. The binary tree is a special type of tree. Each node has at most two child nodes. The data represents structTreeNode{intdata;structTreeNode*left;structTreeNode*right;}; Operation creates a tree traversal tree (predecision, in-order, and later order) search tree insertion node deletes node graph is a collection of data structures, where elements are vertices, and they can be connected together through edges with right or unrighted data representing neighbors.
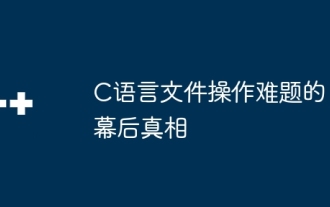
The truth about file operation problems: file opening failed: insufficient permissions, wrong paths, and file occupied. Data writing failed: the buffer is full, the file is not writable, and the disk space is insufficient. Other FAQs: slow file traversal, incorrect text file encoding, and binary file reading errors.
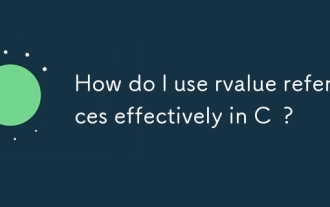
Article discusses effective use of rvalue references in C for move semantics, perfect forwarding, and resource management, highlighting best practices and performance improvements.(159 characters)
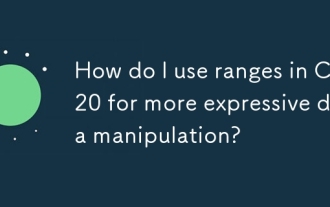
C 20 ranges enhance data manipulation with expressiveness, composability, and efficiency. They simplify complex transformations and integrate into existing codebases for better performance and maintainability.
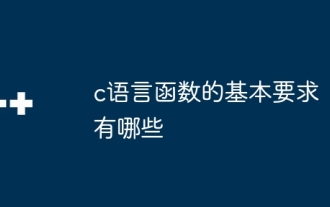
C language functions are the basis for code modularization and program building. They consist of declarations (function headers) and definitions (function bodies). C language uses values to pass parameters by default, but external variables can also be modified using address pass. Functions can have or have no return value, and the return value type must be consistent with the declaration. Function naming should be clear and easy to understand, using camel or underscore nomenclature. Follow the single responsibility principle and keep the function simplicity to improve maintainability and readability.
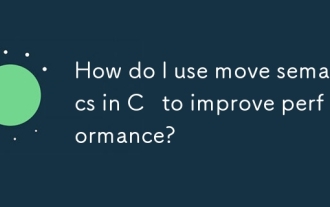
The article discusses using move semantics in C to enhance performance by avoiding unnecessary copying. It covers implementing move constructors and assignment operators, using std::move, and identifies key scenarios and pitfalls for effective appl
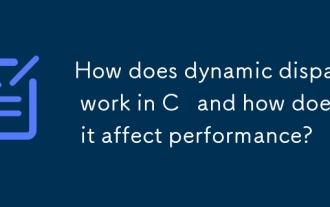
The article discusses dynamic dispatch in C , its performance costs, and optimization strategies. It highlights scenarios where dynamic dispatch impacts performance and compares it with static dispatch, emphasizing trade-offs between performance and
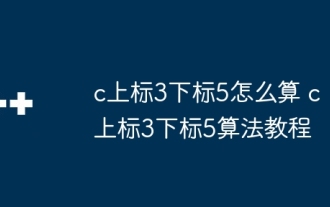
The calculation of C35 is essentially combinatorial mathematics, representing the number of combinations selected from 3 of 5 elements. The calculation formula is C53 = 5! / (3! * 2!), which can be directly calculated by loops to improve efficiency and avoid overflow. In addition, understanding the nature of combinations and mastering efficient calculation methods is crucial to solving many problems in the fields of probability statistics, cryptography, algorithm design, etc.
