How Can I Pass Command Line Arguments to PowerShell Scripts from C#?
Execute PowerShell scripts from C# using command line arguments
Integrating the ability to execute PowerShell scripts in C# applications can bring many advantages. However, to take full advantage of this feature, it's important to understand how to pass command line parameters to PowerShell scripts in C# code. This guide will explore this common scenario and provide a comprehensive solution to help you achieve seamless execution of PowerShell scripts and parameters.
Problem Definition
To execute a PowerShell script from C#, you might use the following code template:
RunspaceConfiguration runspaceConfiguration = RunspaceConfiguration.Create(); Runspace runspace = RunspaceFactory.CreateRunspace(runspaceConfiguration); runspace.Open(); RunspaceInvoke scriptInvoker = new RunspaceInvoke(runspace); Pipeline pipeline = runspace.CreatePipeline(); pipeline.Commands.Add(scriptFile); results = pipeline.Invoke();
In this code, scriptFile
represents the path to the PowerShell script (for example, "C:Program FilesMyProgramWhatever.ps1"). If your script uses command line arguments in the "-key Value" format (where Value can contain spaces), you may have difficulty passing these arguments effectively.
Solution
To solve this problem, you need to build a separate command object for the script file and specify the parameters using the CommandParameter
object:
Command myCommand = new Command(scriptfile); CommandParameter testParam = new CommandParameter("key", "value"); myCommand.Parameters.Add(testParam); pipeline.Commands.Add(myCommand);
This method ensures correct handling of command line arguments, including arguments containing spaces.
Full code example
To summarize, here is the complete modified code containing the solution:
RunspaceConfiguration runspaceConfiguration = RunspaceConfiguration.Create(); Runspace runspace = RunspaceFactory.CreateRunspace(runspaceConfiguration); runspace.Open(); Pipeline pipeline = runspace.CreatePipeline(); // 使用参数构建单独的命令 Command myCommand = new Command(scriptfile); CommandParameter testParam = new CommandParameter("key", "value"); myCommand.Parameters.Add(testParam); pipeline.Commands.Add(myCommand); results = pipeline.Invoke();
By integrating this solution into your C# code, you will be able to confidently execute PowerShell scripts and pass command line arguments, even if the arguments contain spaces. This enhanced functionality will enable you to seamlessly integrate PowerShell scripts into your C# applications.
The above is the detailed content of How Can I Pass Command Line Arguments to PowerShell Scripts from C#?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


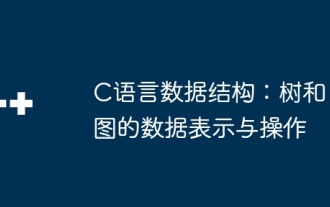
C language data structure: The data representation of the tree and graph is a hierarchical data structure consisting of nodes. Each node contains a data element and a pointer to its child nodes. The binary tree is a special type of tree. Each node has at most two child nodes. The data represents structTreeNode{intdata;structTreeNode*left;structTreeNode*right;}; Operation creates a tree traversal tree (predecision, in-order, and later order) search tree insertion node deletes node graph is a collection of data structures, where elements are vertices, and they can be connected together through edges with right or unrighted data representing neighbors.
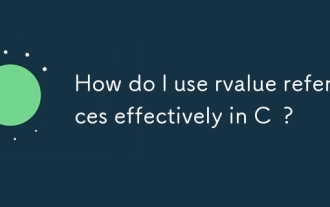
Article discusses effective use of rvalue references in C for move semantics, perfect forwarding, and resource management, highlighting best practices and performance improvements.(159 characters)
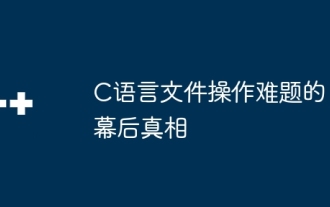
The truth about file operation problems: file opening failed: insufficient permissions, wrong paths, and file occupied. Data writing failed: the buffer is full, the file is not writable, and the disk space is insufficient. Other FAQs: slow file traversal, incorrect text file encoding, and binary file reading errors.
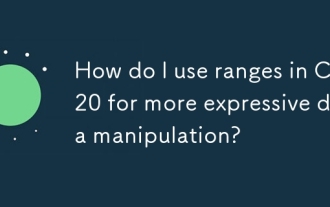
C 20 ranges enhance data manipulation with expressiveness, composability, and efficiency. They simplify complex transformations and integrate into existing codebases for better performance and maintainability.
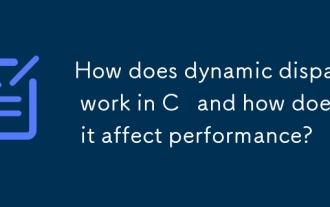
The article discusses dynamic dispatch in C , its performance costs, and optimization strategies. It highlights scenarios where dynamic dispatch impacts performance and compares it with static dispatch, emphasizing trade-offs between performance and
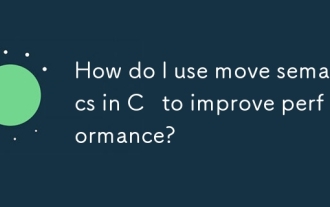
The article discusses using move semantics in C to enhance performance by avoiding unnecessary copying. It covers implementing move constructors and assignment operators, using std::move, and identifies key scenarios and pitfalls for effective appl
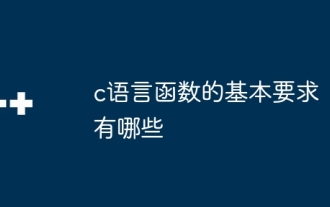
C language functions are the basis for code modularization and program building. They consist of declarations (function headers) and definitions (function bodies). C language uses values to pass parameters by default, but external variables can also be modified using address pass. Functions can have or have no return value, and the return value type must be consistent with the declaration. Function naming should be clear and easy to understand, using camel or underscore nomenclature. Follow the single responsibility principle and keep the function simplicity to improve maintainability and readability.

The article discusses auto type deduction in programming, detailing its benefits like reduced code verbosity and improved maintainability, and its limitations such as potential confusion and debugging challenges.
