EasyJSON: Supercharge JSON Performance in Go
Jan 24, 2025 am 02:18 AMEasyJSON: A High-Performance JSON Solution for Go
Go applications, especially those handling substantial JSON data (like web servers or microservices), often rely on efficient JSON serialization and deserialization. While Go's built-in encoding/json
package is reliable, its performance can lag when processing large datasets. EasyJSON offers a compelling alternative, prioritizing speed and minimal memory usage. This blog post explores EasyJSON's capabilities, benchmarks, and use cases.
Why Choose EasyJSON?
EasyJSON's key advantages stem from its compile-time code generation approach:
- Exceptional Performance: Significantly faster than the standard library and many third-party options.
- No Runtime Reflection: Eliminates the performance overhead associated with reflection.
- Lightweight Footprint: Substantially reduces memory allocations.
- Seamless Integration: Works directly with existing Go structs.
Getting Started
Installation:
go get github.com/mailru/easyjson && go install github.com/mailru/easyjson/...@latest export GOPATH=/Users/<username>/go # Adjust to your GOPATH export PATH=$GOPATH/bin:$PATH
Code Generation:
- Define your struct:
package main type User struct { ID int `json:"id"` Name string `json:"name"` Age int `json:"age"` }
- Generate EasyJSON code:
easyjson -all user.go
This command creates user_easyjson.go
, containing optimized marshaling/unmarshaling functions.
Using EasyJSON
package main import ( "fmt" "github.com/mailru/easyjson" ) //easyjson:json type User struct { ID int `json:"id"` Name string `json:"name"` Age int `json:"age"` } func main() { user := User{ID: 1, Name: "John Doe", Age: 30} // Marshal data, err := easyjson.Marshal(user) if err != nil { panic(err) } fmt.Println("JSON:", string(data)) // Unmarshal var deserialized User if err := easyjson.Unmarshal(data, &deserialized); err != nil { panic(err) } fmt.Println("Struct:", deserialized) }
Performance Benchmarks
Comparative benchmarks (against encoding/json
and json-iterator
) using complex nested structs reveal EasyJSON's significant speed advantage and drastically reduced memory allocation. (Refer to the provided Github repository for detailed benchmark code and results). Generally, EasyJSON demonstrates up to a 3x speed improvement over encoding/json
.
EasyJSON vs. Alternatives
Feature |
|
EasyJSON | |||||||||||||||||||||||||
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
Reflection-Free | No | No | Yes | ||||||||||||||||||||||||
Performance | Medium | High | Very High | ||||||||||||||||||||||||
Memory Efficiency | Medium | High | Very High | ||||||||||||||||||||||||
Code Generation | No | No | Yes | ||||||||||||||||||||||||
API Simplicity | Simple | Simple | Simple |
When to Use EasyJSON
EasyJSON is best suited for:
- High-throughput APIs demanding low-latency JSON handling.
- Memory-constrained environments.
- Applications where compile-time code generation is acceptable.
Caveats
- Code Generation Step: Requires an additional build step.
- Maintenance: Struct modifications necessitate regenerating EasyJSON code.
Conclusion
EasyJSON provides a substantial performance boost for JSON processing in Go. While the code generation adds a small development overhead, the significant gains in speed and efficiency make it a strong choice for performance-critical applications. Consider EasyJSON if your Go project prioritizes speed and reduced memory consumption.
The above is the detailed content of EasyJSON: Supercharge JSON Performance in Go. For more information, please follow other related articles on the PHP Chinese website!

Hot Article

Hot tools Tags

Hot Article

Hot Article Tags

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
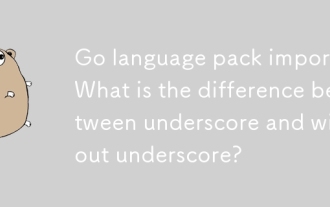
Go language pack import: What is the difference between underscore and without underscore?

How to implement short-term information transfer between pages in the Beego framework?
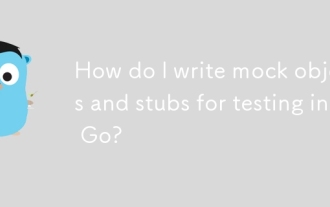
How do I write mock objects and stubs for testing in Go?
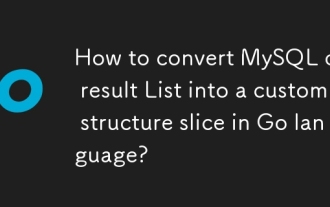
How to convert MySQL query result List into a custom structure slice in Go language?
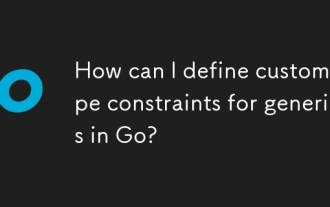
How can I define custom type constraints for generics in Go?
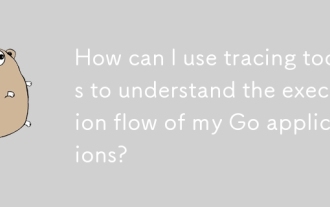
How can I use tracing tools to understand the execution flow of my Go applications?
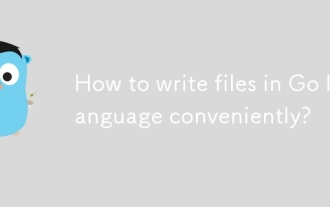
How to write files in Go language conveniently?
