


How to Deserialize a JSON Array of Mixed-Type Values into a Strongly-Typed C# Class?
Deserializing JSON Arrays with Mixed Data Types into C# Classes
This guide addresses the complexities of deserializing JSON data where the structure presents challenges for direct mapping to strongly-typed C# classes. Specifically, we'll tackle scenarios involving arrays of mixed data types within a seemingly dictionary-like structure.
The Problem:
Common JSON structures may present data where:
- Un-named Arrays: Arrays of values lack explicit property names, making direct deserialization difficult.
- Mixed Data Types: Arrays contain elements of varying types (e.g., integers, strings).
- Dictionary-like Structure: The overall structure resembles a dictionary, but the values are these problematic arrays.
Solutions:
Several techniques can overcome these obstacles:
1. Leveraging ObjectToArrayConverter
:
Json.NET's ObjectToArrayConverter
provides a powerful mechanism to map an array of values to a C# object's properties. This requires careful consideration of property order.
1 2 3 4 5 6 7 8 9 |
|
The JsonProperty
attribute's Order
property is crucial; it ensures the correct mapping of array elements to properties based on their sequence.
2. Restructuring the Root Object:
A more straightforward approach might involve restructuring the root object to directly represent the dictionary-like nature of the data.
1 2 3 4 5 |
|
This simplifies deserialization significantly, as the dictionary's keys and values directly correspond to the JSON structure.
3. Custom Converter (Advanced):
For complex scenarios, a custom JsonConverter offers the most control. This allows for intricate parsing logic tailored to the specific JSON structure.
Example (Using ObjectToArrayConverter
):
1 2 3 4 5 6 7 8 9 10 11 |
|
Remember to install the Newtonsoft.Json NuGet package for Json.NET functionality. Choose the solution that best suits your JSON structure and complexity. Restructuring the root object often provides the simplest and most maintainable solution. ObjectToArrayConverter
is a powerful tool for more intricate scenarios requiring precise property order control. A custom converter should be considered only when the other methods are insufficient.
The above is the detailed content of How to Deserialize a JSON Array of Mixed-Type Values into a Strongly-Typed C# Class?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










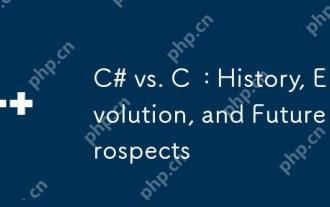
The history and evolution of C# and C are unique, and the future prospects are also different. 1.C was invented by BjarneStroustrup in 1983 to introduce object-oriented programming into the C language. Its evolution process includes multiple standardizations, such as C 11 introducing auto keywords and lambda expressions, C 20 introducing concepts and coroutines, and will focus on performance and system-level programming in the future. 2.C# was released by Microsoft in 2000. Combining the advantages of C and Java, its evolution focuses on simplicity and productivity. For example, C#2.0 introduced generics and C#5.0 introduced asynchronous programming, which will focus on developers' productivity and cloud computing in the future.
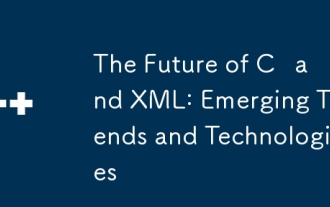
The future development trends of C and XML are: 1) C will introduce new features such as modules, concepts and coroutines through the C 20 and C 23 standards to improve programming efficiency and security; 2) XML will continue to occupy an important position in data exchange and configuration files, but will face the challenges of JSON and YAML, and will develop in a more concise and easy-to-parse direction, such as the improvements of XMLSchema1.1 and XPath3.1.
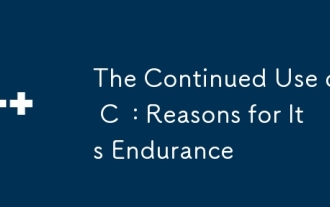
C Reasons for continuous use include its high performance, wide application and evolving characteristics. 1) High-efficiency performance: C performs excellently in system programming and high-performance computing by directly manipulating memory and hardware. 2) Widely used: shine in the fields of game development, embedded systems, etc. 3) Continuous evolution: Since its release in 1983, C has continued to add new features to maintain its competitiveness.
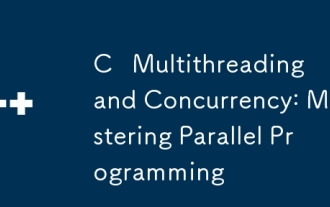
C The core concepts of multithreading and concurrent programming include thread creation and management, synchronization and mutual exclusion, conditional variables, thread pooling, asynchronous programming, common errors and debugging techniques, and performance optimization and best practices. 1) Create threads using the std::thread class. The example shows how to create and wait for the thread to complete. 2) Synchronize and mutual exclusion to use std::mutex and std::lock_guard to protect shared resources and avoid data competition. 3) Condition variables realize communication and synchronization between threads through std::condition_variable. 4) The thread pool example shows how to use the ThreadPool class to process tasks in parallel to improve efficiency. 5) Asynchronous programming uses std::as
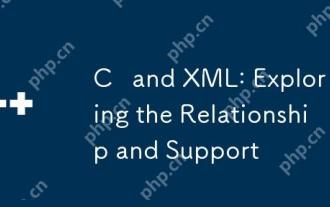
C interacts with XML through third-party libraries (such as TinyXML, Pugixml, Xerces-C). 1) Use the library to parse XML files and convert them into C-processable data structures. 2) When generating XML, convert the C data structure to XML format. 3) In practical applications, XML is often used for configuration files and data exchange to improve development efficiency.
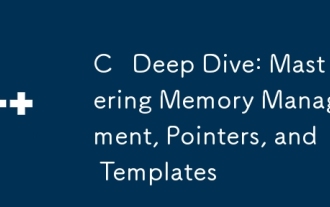
C's memory management, pointers and templates are core features. 1. Memory management manually allocates and releases memory through new and deletes, and pay attention to the difference between heap and stack. 2. Pointers allow direct operation of memory addresses, and use them with caution. Smart pointers can simplify management. 3. Template implements generic programming, improves code reusability and flexibility, and needs to understand type derivation and specialization.
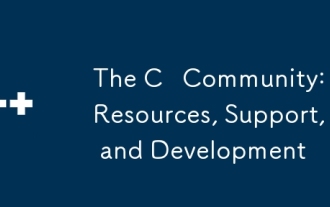
C Learners and developers can get resources and support from StackOverflow, Reddit's r/cpp community, Coursera and edX courses, open source projects on GitHub, professional consulting services, and CppCon. 1. StackOverflow provides answers to technical questions; 2. Reddit's r/cpp community shares the latest news; 3. Coursera and edX provide formal C courses; 4. Open source projects on GitHub such as LLVM and Boost improve skills; 5. Professional consulting services such as JetBrains and Perforce provide technical support; 6. CppCon and other conferences help careers
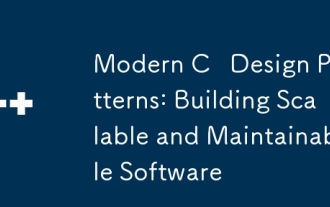
The modern C design model uses new features of C 11 and beyond to help build more flexible and efficient software. 1) Use lambda expressions and std::function to simplify observer pattern. 2) Optimize performance through mobile semantics and perfect forwarding. 3) Intelligent pointers ensure type safety and resource management.
