


Why Does SQL Server Prevent UPDATE with OUTPUT Clause When Triggers Exist?
SQL Server UPDATE Statement: Resolving Conflicts Between OUTPUT Clause and Triggers
Executing an UPDATE
statement with an OUTPUT
clause in SQL Server can result in an error ("Cannot use UPDATE with OUTPUT clause when a trigger is on the table") if triggers are enabled on the affected table. This limitation stems from the potential for triggers to modify data after the OUTPUT
clause captures its values, leading to inconsistencies.
The Problem Explained
The error arises because SQL Server cannot reliably determine the final output values when triggers are involved. Triggers might alter the data before the OUTPUT
clause completes, making the returned values inaccurate. This is particularly true when using the OUTPUT
clause without the INTO
clause.
Solutions
Two primary solutions circumvent this limitation:
Method 1: Employing the INTO
Clause
Redirect the output values to a table variable or temporary table using the INTO
clause. This isolates the output from potential trigger modifications:
UPDATE BatchReports SET IsProcessed = 1 OUTPUT inserted.* INTO @t -- @t is a table variable or temporary table WHERE BatchReports.BatchReportGUID = @someGuid
This approach guarantees that the captured data reflects the state after the UPDATE
and any associated trigger actions.
Method 2: Separate SELECT
and UPDATE
Statements
Retrieve the necessary data using a SELECT
statement before executing the UPDATE
:
SELECT BatchFileXml, ResponseFileXml, ProcessedDate INTO #tempTable -- Create a temporary table FROM BatchReports WHERE BatchReports.BatchReportGUID = @someGuid; UPDATE BatchReports SET IsProcessed = 1 WHERE BatchReports.BatchReportGUID = @someGuid; SELECT * FROM #tempTable; -- Access the desired values from the temporary table
This method ensures that the SELECT
captures the original data, unaffected by subsequent trigger actions during the UPDATE
.
Important Note: Avoiding OUTPUT
with Triggers
Using the OUTPUT
clause directly with triggers is generally discouraged. The potential for discrepancies between the OUTPUT
values and the final data state after trigger execution makes this approach unreliable. The solutions outlined above provide safer and more predictable results.
The above is the detailed content of Why Does SQL Server Prevent UPDATE with OUTPUT Clause When Triggers Exist?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


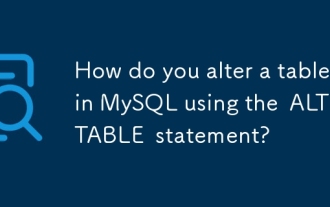
The article discusses using MySQL's ALTER TABLE statement to modify tables, including adding/dropping columns, renaming tables/columns, and changing column data types.
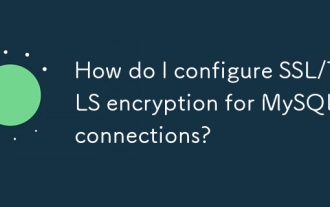
Article discusses configuring SSL/TLS encryption for MySQL, including certificate generation and verification. Main issue is using self-signed certificates' security implications.[Character count: 159]
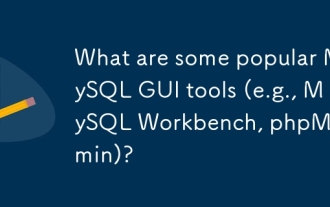
Article discusses popular MySQL GUI tools like MySQL Workbench and phpMyAdmin, comparing their features and suitability for beginners and advanced users.[159 characters]
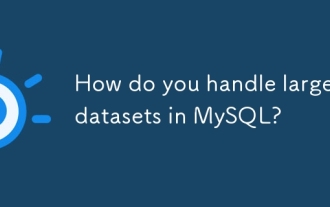
Article discusses strategies for handling large datasets in MySQL, including partitioning, sharding, indexing, and query optimization.
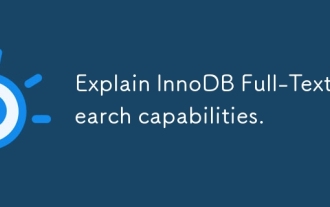
InnoDB's full-text search capabilities are very powerful, which can significantly improve database query efficiency and ability to process large amounts of text data. 1) InnoDB implements full-text search through inverted indexing, supporting basic and advanced search queries. 2) Use MATCH and AGAINST keywords to search, support Boolean mode and phrase search. 3) Optimization methods include using word segmentation technology, periodic rebuilding of indexes and adjusting cache size to improve performance and accuracy.
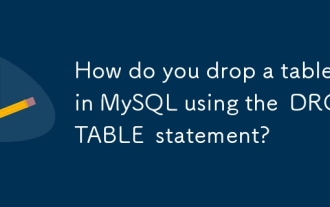
The article discusses dropping tables in MySQL using the DROP TABLE statement, emphasizing precautions and risks. It highlights that the action is irreversible without backups, detailing recovery methods and potential production environment hazards.
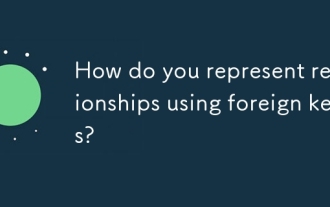
Article discusses using foreign keys to represent relationships in databases, focusing on best practices, data integrity, and common pitfalls to avoid.
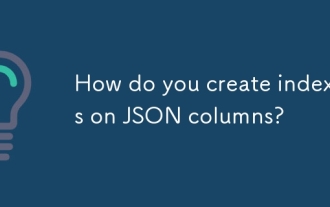
The article discusses creating indexes on JSON columns in various databases like PostgreSQL, MySQL, and MongoDB to enhance query performance. It explains the syntax and benefits of indexing specific JSON paths, and lists supported database systems.
