How to Correctly Group, Sum, and Count Products in LINQ with GroupBy?
Jan 24, 2025 pm 05:01 PMGroup, sum and count products using LINQ: GroupBy method
Problem Description
We have a collection of products that need to be grouped by product code. The goal is to create an object that contains the product name, product quantity, and total price for each product code.
Code snippet (error example)
The initial code uses GroupBy
to group by product code and then calculate the sum and count for each group.
List<resultline> result = Lines .GroupBy(l => l.ProductCode) .SelectMany(cl => cl.Select( csLine => new ResultLine { ProductName = csLine.Name, Quantity = cl.Count().ToString(), Price = cl.Sum(c => c.Price).ToString(), })).ToList<resultline>();
The problem
While this code calculates the sum correctly, the count for all products always shows 1.
Example data
List<cartline> Lines = new List<cartline>(); Lines.Add(new CartLine() { ProductCode = "p1", Price = 6.5M, Name = "Product1" }); Lines.Add(new CartLine() { ProductCode = "p1", Price = 6.5M, Name = "Product1" }); Lines.Add(new CartLine() { ProductCode = "p2", Price = 12M, Name = "Product2" });
Expected results
<code>Product1: count 2 - Price:13 (2x6.5) Product2: count 1 - Price:12 (1x12)</code>
Solution
The problem is using SelectMany
to check every item in every group. The correct approach is:
List<resultline> result = Lines .GroupBy(l => l.ProductCode) .Select(cl => new ResultLine { ProductName = cl.First().Name, Quantity = cl.Count().ToString(), Price = cl.Sum(c => c.Price).ToString(), }).ToList();
Assuming products with the same code have the same name, we can use First()
to get the product name. SelectMany
flattens the result set, causing counting errors, while Select
directly operates on the grouped results.
This revised answer maintains the image and provides a more accurate and concise explanation of the LINQ problem and solution. The wording is also adjusted for improved clarity and flow.
The above is the detailed content of How to Correctly Group, Sum, and Count Products in LINQ with GroupBy?. For more information, please follow other related articles on the PHP Chinese website!

Hot Article

Hot tools Tags

Hot Article

Hot Article Tags

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
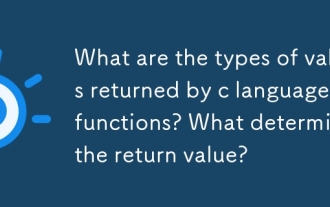
What are the types of values returned by c language functions? What determines the return value?

What are the definitions and calling rules of c language functions and what are the
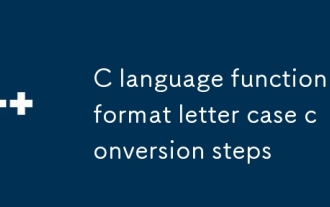
C language function format letter case conversion steps

Where is the return value of the c language function stored in memory?
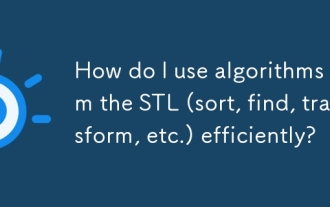
How do I use algorithms from the STL (sort, find, transform, etc.) efficiently?
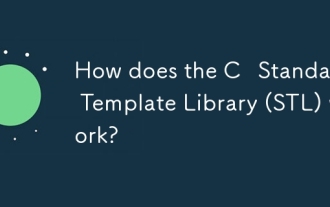
How does the C Standard Template Library (STL) work?
