What Unexpected Behaviors and Corner Cases Exist in C# and .NET?
C# and .NET: Unveiling Hidden Surprises
Software development often reveals unexpected behaviors. C# and .NET, while powerful, are no exception. This article explores some intriguing corner cases that can challenge even experienced developers.
String Creation: A Counterintuitive Result
Consider this seemingly simple code snippet:
string x = new string(new char[0]); string y = new string(new char[0]); Console.WriteLine(object.ReferenceEquals(x, y));
The output is True
, contradicting the expectation that new
creates distinct objects for reference types. The Common Language Runtime (CLR) optimizes this specific scenario, reusing the same empty string instance.
Generic Types and Nullable<T>: A NullReferenceException Mystery
The following code demonstrates another unexpected behavior:
static void Foo<T>() where T : new() { T t = new T(); Console.WriteLine(t.ToString()); // Works fine Console.WriteLine(t.GetHashCode()); // Works fine Console.WriteLine(t.Equals(t)); // Works fine // This throws a NullReferenceException... Console.WriteLine(t.GetType()); }
When T
is Nullable<T>
(e.g., int?
), a NullReferenceException
occurs when calling GetType()
. This is because Nullable<T>
overrides most methods, but not GetType()
. The boxing process during the call to the non-overridden GetType()
results in a null value.
Proxy Attributes and the new()
Constraint: Defying Expectations
Ayende Rahien highlighted a similar, yet more sophisticated, scenario:
private static void Main() { CanThisHappen<MyFunnyType>(); } public static void CanThisHappen<T>() where T : class, new() { var instance = new T(); // new() on a ref-type; should be non-null, then Debug.Assert(instance != null, "How did we break the CLR?"); }
This code, surprisingly, can fail the assertion. By using a proxy attribute (like MyFunnyProxyAttribute
) that intercepts the new()
call and returns null
, the assertion can be violated. This demonstrates the potential for unexpected interactions between runtime behavior and custom attributes. These examples highlight the importance of thorough testing and a deep understanding of the CLR's inner workings to avoid unexpected pitfalls in C# and .NET development.
The above is the detailed content of What Unexpected Behaviors and Corner Cases Exist in C# and .NET?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


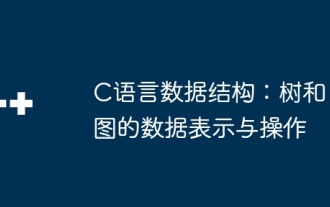
C language data structure: The data representation of the tree and graph is a hierarchical data structure consisting of nodes. Each node contains a data element and a pointer to its child nodes. The binary tree is a special type of tree. Each node has at most two child nodes. The data represents structTreeNode{intdata;structTreeNode*left;structTreeNode*right;}; Operation creates a tree traversal tree (predecision, in-order, and later order) search tree insertion node deletes node graph is a collection of data structures, where elements are vertices, and they can be connected together through edges with right or unrighted data representing neighbors.
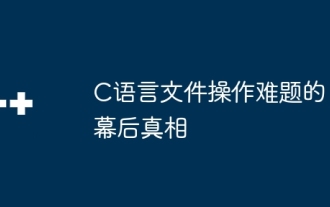
The truth about file operation problems: file opening failed: insufficient permissions, wrong paths, and file occupied. Data writing failed: the buffer is full, the file is not writable, and the disk space is insufficient. Other FAQs: slow file traversal, incorrect text file encoding, and binary file reading errors.
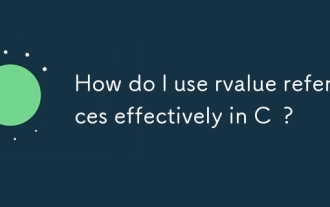
Article discusses effective use of rvalue references in C for move semantics, perfect forwarding, and resource management, highlighting best practices and performance improvements.(159 characters)
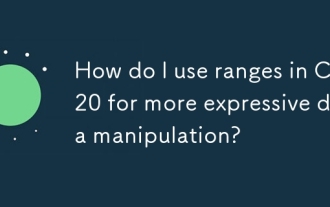
C 20 ranges enhance data manipulation with expressiveness, composability, and efficiency. They simplify complex transformations and integrate into existing codebases for better performance and maintainability.
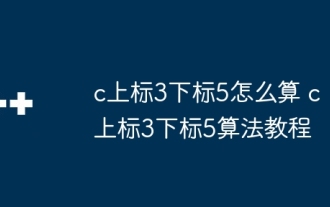
The calculation of C35 is essentially combinatorial mathematics, representing the number of combinations selected from 3 of 5 elements. The calculation formula is C53 = 5! / (3! * 2!), which can be directly calculated by loops to improve efficiency and avoid overflow. In addition, understanding the nature of combinations and mastering efficient calculation methods is crucial to solving many problems in the fields of probability statistics, cryptography, algorithm design, etc.
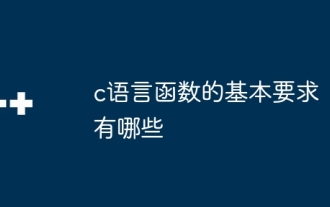
C language functions are the basis for code modularization and program building. They consist of declarations (function headers) and definitions (function bodies). C language uses values to pass parameters by default, but external variables can also be modified using address pass. Functions can have or have no return value, and the return value type must be consistent with the declaration. Function naming should be clear and easy to understand, using camel or underscore nomenclature. Follow the single responsibility principle and keep the function simplicity to improve maintainability and readability.
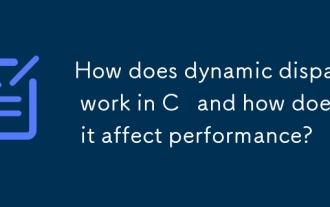
The article discusses dynamic dispatch in C , its performance costs, and optimization strategies. It highlights scenarios where dynamic dispatch impacts performance and compares it with static dispatch, emphasizing trade-offs between performance and
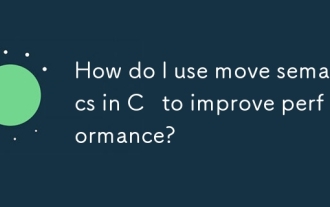
The article discusses using move semantics in C to enhance performance by avoiding unnecessary copying. It covers implementing move constructors and assignment operators, using std::move, and identifies key scenarios and pitfalls for effective appl
