What are access modifiers in Java?
Java access modifiers control the visibility and accessibility of classes, methods, constructors, and data members. There are four access modifiers in Java:
public: Classes, methods and data members can be accessed from anywhere in the program.
public class MyClass { public void display() { System.out.println("Public method"); } }
private: Data members can only be accessed within the same class in which they are declared, and cannot be accessed by other classes even within the same package.
public class MyClass { private int data = 10; private void display() { System.out.println("Private method"); } }
protected: Data members and methods are accessible in the same package and subclasses.
public class MyClass { protected int data = 10; protected void display() { System.out.println("Protected method"); } }
default (package access): If no keyword is specified, the default access modifier is applied, which makes the class, method or data member only accessible within the same package.
class MyClass { // default access void display() { // default access System.out.println("Default method"); } }
Thanks for reading! Welcome to raise your questions and suggestions in the comment area and learn and make progress together!
The above is the detailed content of What are access modifiers in Java?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










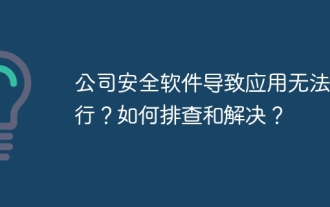
Troubleshooting and solutions to the company's security software that causes some applications to not function properly. Many companies will deploy security software in order to ensure internal network security. ...
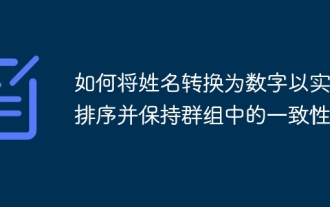
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
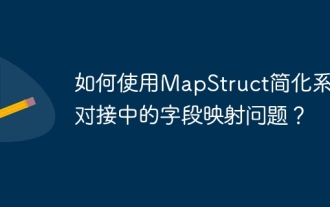
Field mapping processing in system docking often encounters a difficult problem when performing system docking: how to effectively map the interface fields of system A...
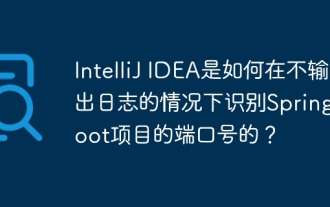
Start Spring using IntelliJIDEAUltimate version...
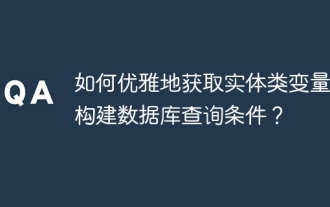
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
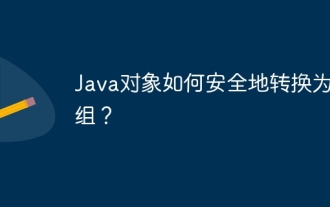
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
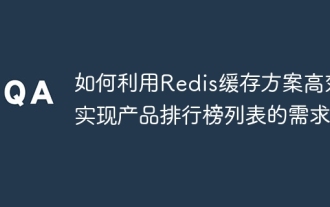
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
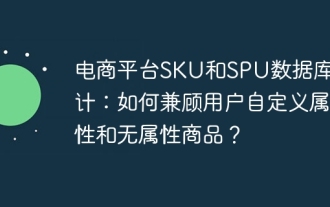
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
