Law of Demeter (LoD) Explained in Seconds
The Law of Demeter (LoD): Keep Your Code Loosely Coupled
The Law of Demeter (LoD) is a design guideline aimed at reducing coupling in your code. Its core principle is simple: "Only talk to your immediate friends, not to strangers."
In essence, a class or module should only interact directly with objects it depends on, avoiding interactions with objects those objects depend on. This promotes simpler, more testable, and less interconnected code.
Anti-Pattern (Avoid):
<code>// Tight coupling through nested calls customerCity := order.GetCustomer().GetAddress().GetCity() fmt.Printf("Customer lives in: %s\n", customerCity)</code>
This example demonstrates tight coupling. Changes to the Order
, Customer
, or Address
classes could break this code.
Improved Approach:
<code>// Decoupled using a single method call customerCity := order.GetCustomerCity() fmt.Printf("Customer lives in: %s\n", customerCity)</code>
The GetCustomerCity()
method encapsulates the complexity, hiding the internal structure and reducing dependencies.
Benefits of LoD:
- Reduced Coupling: Easier to maintain and modify code with fewer interdependencies.
- Improved Readability: Code becomes more straightforward and easier to understand.
- Information Hiding: Internal implementation details are shielded from external components.
- Easier Testing: Simplifies testing by reducing the need for complex mocks.
Applying LoD in Practice:
- Employ Data Transfer Objects (DTOs) to manage data flow.
- Utilize Facade patterns to simplify interactions with complex subsystems.
- Refactor chained method calls into single, higher-level methods.
Further Exploration:
Interested in learning more about software design principles? Explore these related concepts:
- Dependency Inversion Principle (DIP)
- Golang Dependency Injection
- Interface Segregation Principle (ISP)
- You Aren't Gonna Need It (YAGNI) Principle
- Liskov Substitution Principle (LSP)
- Keep It Simple, Stupid (KISS) Principle
- Don't Repeat Yourself (DRY) Principle
- Tell, Don't Ask Principle
Connect with me on LinkedIn, GitHub, and Twitter/X for updates on future posts.
The above is the detailed content of Law of Demeter (LoD) Explained in Seconds. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










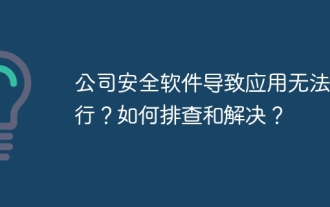
Troubleshooting and solutions to the company's security software that causes some applications to not function properly. Many companies will deploy security software in order to ensure internal network security. ...
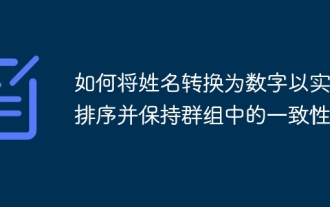
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
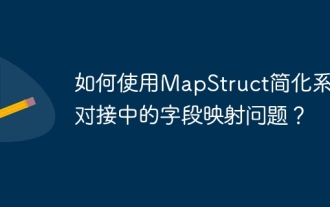
Field mapping processing in system docking often encounters a difficult problem when performing system docking: how to effectively map the interface fields of system A...
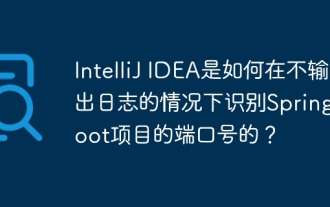
Start Spring using IntelliJIDEAUltimate version...
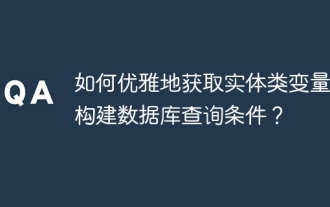
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
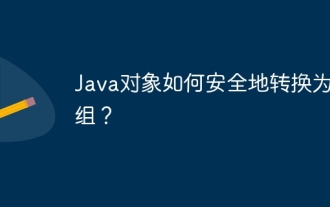
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
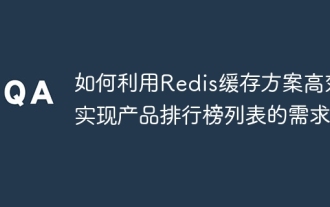
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
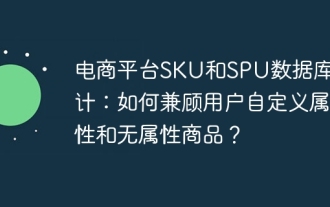
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
