How to Work with HashMap in Java
This comprehensive guide explores Java's HashMap
, a robust data structure for efficient key-value pair storage and management. We'll cover fundamental methods and provide practical examples to solidify your understanding.
Understanding HashMap
HashMap
stores data as key-value pairs, offering (on average) constant-time complexity for core operations like put
, get
, and remove
. Key advantages include:
- Unique Keys: Each key must be unique; values can be duplicated.
- Versatile Data Types: Keys and values can be any object type.
-
Package Location: Resides within the
java.util
package. -
Null Handling: Accepts
null
as both a key and a value.
Illustrative Example:
import java.util.HashMap; public class HashMapDemo { public static void main(String[] args) { HashMap<Integer, String> myMap = new HashMap<>(); // Adding entries myMap.put(1, "Apple"); myMap.put(2, "Banana"); myMap.put(3, "Cherry"); // Retrieving a value System.out.println(myMap.get(1)); // Output: Apple } }
HashMap Creation
The HashMap
constructor is straightforward:
HashMap<KeyType, ValueType> mapName = new HashMap<>();
Example:
HashMap<String, Integer> wordCounts = new HashMap<>();
Here, String
represents the key type and Integer
the value type.
Essential HashMap Methods
Let's delve into frequently used HashMap
methods:
1. put(K key, V value)
- Functionality: Adds a key-value pair. If the key exists, the value is updated.
- Example:
import java.util.HashMap; public class HashMapDemo { public static void main(String[] args) { HashMap<Integer, String> myMap = new HashMap<>(); // Adding entries myMap.put(1, "Apple"); myMap.put(2, "Banana"); myMap.put(3, "Cherry"); // Retrieving a value System.out.println(myMap.get(1)); // Output: Apple } }
2. get(Object key)
- Functionality: Retrieves the value associated with the given key. Returns
null
if the key is absent. - Example:
HashMap<KeyType, ValueType> mapName = new HashMap<>();
3. getOrDefault(Object key, V defaultValue)
- Functionality: Retrieves the value; if the key is missing, returns the
defaultValue
. - Example:
HashMap<String, Integer> wordCounts = new HashMap<>();
4. containsKey(Object key)
- Functionality: Checks if the map contains the specified key.
- Example:
HashMap<Integer, String> myMap = new HashMap<>(); myMap.put(1, "Apple"); myMap.put(2, "Banana"); myMap.put(1, "Orange"); // Updates value for key 1 System.out.println(myMap); // Output: {1=Orange, 2=Banana}
5. containsValue(Object value)
- Functionality: Checks if the map contains the specified value.
- Example:
System.out.println(myMap.get(1)); // Output: Orange System.out.println(myMap.get(4)); // Output: null
6. remove(Object key)
- Functionality: Removes the entry for the given key and returns its value (or
null
if not found). - Example:
System.out.println(myMap.getOrDefault(4, "Default")); // Output: Default
7. putIfAbsent(K key, V value)
- Functionality: Adds the key-value pair only if the key doesn't already exist.
- Example:
System.out.println(myMap.containsKey(1)); // Output: true System.out.println(myMap.containsKey(4)); // Output: false
8. replace(K key, V value)
- Functionality: Replaces the value for the key only if the key exists.
- Example:
System.out.println(myMap.containsValue("Orange")); // Output: true System.out.println(myMap.containsValue("Grape")); // Output: false
9. keySet()
- Functionality: Returns a
Set
of all keys in the map. - Example:
System.out.println(myMap.remove(1)); // Output: Orange System.out.println(myMap); // Output: {2=Banana}
10. values()
- Functionality: Returns a
Collection
of all values in the map. - Example:
myMap.putIfAbsent(3, "Cherry"); // No change if key 3 exists System.out.println(myMap);
11. entrySet()
- Functionality: Returns a
Set
of all key-value pairs (Map.Entry
). - Example:
myMap.replace(2, "Mango"); System.out.println(myMap);
12. compute(K key, BiFunction remappingFunction)
- Functionality: Updates the value using a provided function.
- Example: (Requires a
BiFunction
implementation)
import java.util.HashMap; public class HashMapDemo { public static void main(String[] args) { HashMap<Integer, String> myMap = new HashMap<>(); // Adding entries myMap.put(1, "Apple"); myMap.put(2, "Banana"); myMap.put(3, "Cherry"); // Retrieving a value System.out.println(myMap.get(1)); // Output: Apple } }
13. merge(K key, V value, BiFunction remappingFunction)
- Functionality: Combines a new value with the existing value using a function.
- Example: (Requires a
BiFunction
implementation)
HashMap<KeyType, ValueType> mapName = new HashMap<>();
Comprehensive Example: Word Frequency Analysis
This example showcases HashMap
for counting word frequencies:
HashMap<String, Integer> wordCounts = new HashMap<>();
Conclusion
HashMap
is a fundamental Java data structure, offering efficient key-value pair management. Mastering its methods empowers you to tackle diverse programming challenges, from simple data lookups to sophisticated data manipulation tasks. Incorporate HashMap
into your projects to harness its power and efficiency.
The above is the detailed content of How to Work with HashMap in Java. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


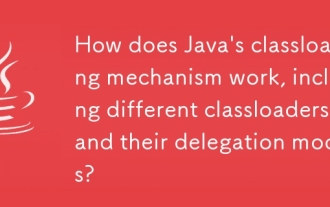
Java's classloading involves loading, linking, and initializing classes using a hierarchical system with Bootstrap, Extension, and Application classloaders. The parent delegation model ensures core classes are loaded first, affecting custom class loa
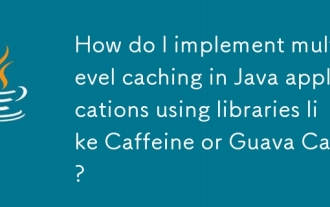
The article discusses implementing multi-level caching in Java using Caffeine and Guava Cache to enhance application performance. It covers setup, integration, and performance benefits, along with configuration and eviction policy management best pra
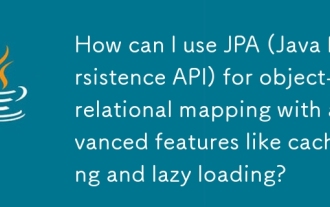
The article discusses using JPA for object-relational mapping with advanced features like caching and lazy loading. It covers setup, entity mapping, and best practices for optimizing performance while highlighting potential pitfalls.[159 characters]
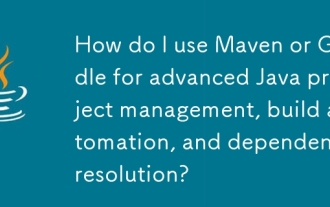
The article discusses using Maven and Gradle for Java project management, build automation, and dependency resolution, comparing their approaches and optimization strategies.
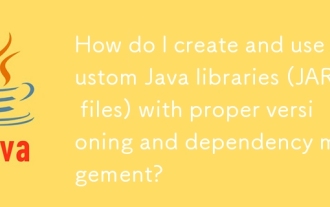
The article discusses creating and using custom Java libraries (JAR files) with proper versioning and dependency management, using tools like Maven and Gradle.
