


How Can a Flat Table Representing a Tree Hierarchy Be Efficiently Parsed into a Nested Tree Structure?
Transforming Flat Data into a Hierarchical Tree
Efficiently converting a flat table representing a tree hierarchy into a nested tree structure is a common programming challenge. A recursive algorithm offers an elegant and effective solution.
Here's a Python example demonstrating this approach:
# Initialize the tree as a dictionary tree = {} # Process each row from the flat table for row in table: # Add the node to the tree tree[row['Id']] = { 'name': row['Name'], 'parent_id': row['ParentId'] if row['ParentId'] else None, 'children': [] # Initialize an empty list for children } # Populate the children for each node for node_id, node in tree.items(): if node['parent_id']: tree[node['parent_id']]['children'].append(node_id)
This code creates a nested dictionary. Each dictionary entry represents a node with 'name', 'parent_id', and a list of 'children' IDs. This structure facilitates easy tree traversal.
Optimizing Tree Storage in Relational Databases
While nested sets and path enumeration are viable options, the Closure Table method presents several benefits for storing hierarchical data in an RDBMS:
- Ease of Implementation: It involves a single additional table, simplifying implementation and maintenance.
- Query Flexibility: Recursive queries are readily implemented in most modern SQL databases, enabling straightforward traversal and manipulation of the hierarchy.
- Performance Advantages: Database engines can effectively optimize queries using indexes on the Closure Table's primary key, leading to improved performance.
In summary, the Closure Table approach provides a robust and efficient method for managing and querying tree structures within relational databases.
The above is the detailed content of How Can a Flat Table Representing a Tree Hierarchy Be Efficiently Parsed into a Nested Tree Structure?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


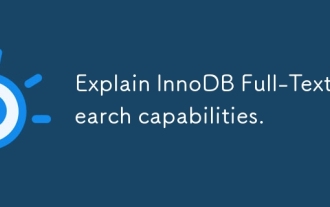
InnoDB's full-text search capabilities are very powerful, which can significantly improve database query efficiency and ability to process large amounts of text data. 1) InnoDB implements full-text search through inverted indexing, supporting basic and advanced search queries. 2) Use MATCH and AGAINST keywords to search, support Boolean mode and phrase search. 3) Optimization methods include using word segmentation technology, periodic rebuilding of indexes and adjusting cache size to improve performance and accuracy.
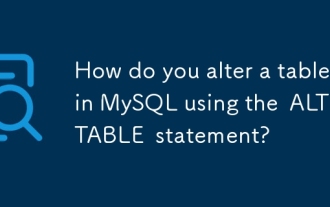
The article discusses using MySQL's ALTER TABLE statement to modify tables, including adding/dropping columns, renaming tables/columns, and changing column data types.
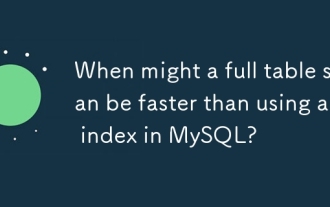
Full table scanning may be faster in MySQL than using indexes. Specific cases include: 1) the data volume is small; 2) when the query returns a large amount of data; 3) when the index column is not highly selective; 4) when the complex query. By analyzing query plans, optimizing indexes, avoiding over-index and regularly maintaining tables, you can make the best choices in practical applications.
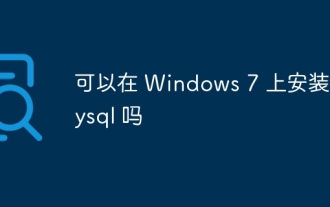
Yes, MySQL can be installed on Windows 7, and although Microsoft has stopped supporting Windows 7, MySQL is still compatible with it. However, the following points should be noted during the installation process: Download the MySQL installer for Windows. Select the appropriate version of MySQL (community or enterprise). Select the appropriate installation directory and character set during the installation process. Set the root user password and keep it properly. Connect to the database for testing. Note the compatibility and security issues on Windows 7, and it is recommended to upgrade to a supported operating system.
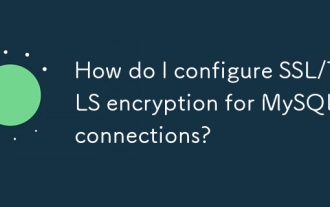
Article discusses configuring SSL/TLS encryption for MySQL, including certificate generation and verification. Main issue is using self-signed certificates' security implications.[Character count: 159]
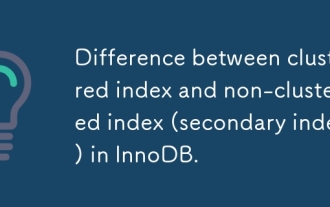
The difference between clustered index and non-clustered index is: 1. Clustered index stores data rows in the index structure, which is suitable for querying by primary key and range. 2. The non-clustered index stores index key values and pointers to data rows, and is suitable for non-primary key column queries.
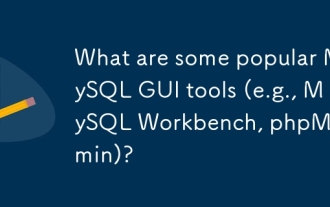
Article discusses popular MySQL GUI tools like MySQL Workbench and phpMyAdmin, comparing their features and suitability for beginners and advanced users.[159 characters]
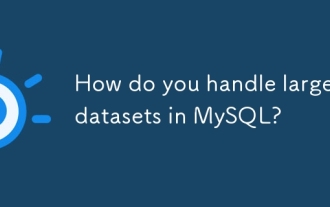
Article discusses strategies for handling large datasets in MySQL, including partitioning, sharding, indexing, and query optimization.
