How to Remove HTML Tags from a String Using C# Regular Expressions?
Efficiently Removing HTML Tags from C# Strings
Cleaning text data by removing HTML tags is a frequent requirement in many C# applications. While regular expressions offer a concise solution, they might not always be the most robust method, especially when dealing with complex HTML structures.
A simple regular expression to remove HTML tags is:
<[^>]*>
This expression identifies and matches any characters enclosed within angle brackets, effectively targeting HTML tags. The Regex.Replace
method then facilitates the removal:
string cleanText = Regex.Replace(htmlString, @"<[^>]*>", string.Empty);
This code snippet replaces all matched tags with an empty string, leaving only the plain text.
Important Considerations:
This regex approach has limitations. It might fail to correctly handle scenarios involving nested tags or CDATA sections containing angle brackets. For more complex HTML, a dedicated HTML parser offers superior accuracy and reliability. Using an XML parser is a better alternative for robust HTML tag removal in such situations.
The above is the detailed content of How to Remove HTML Tags from a String Using C# Regular Expressions?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


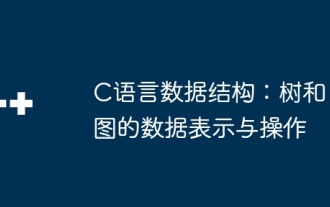
C language data structure: The data representation of the tree and graph is a hierarchical data structure consisting of nodes. Each node contains a data element and a pointer to its child nodes. The binary tree is a special type of tree. Each node has at most two child nodes. The data represents structTreeNode{intdata;structTreeNode*left;structTreeNode*right;}; Operation creates a tree traversal tree (predecision, in-order, and later order) search tree insertion node deletes node graph is a collection of data structures, where elements are vertices, and they can be connected together through edges with right or unrighted data representing neighbors.
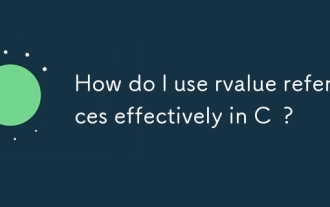
Article discusses effective use of rvalue references in C for move semantics, perfect forwarding, and resource management, highlighting best practices and performance improvements.(159 characters)
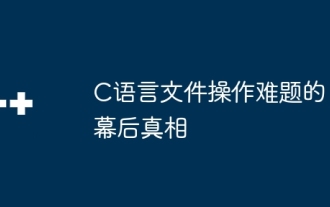
The truth about file operation problems: file opening failed: insufficient permissions, wrong paths, and file occupied. Data writing failed: the buffer is full, the file is not writable, and the disk space is insufficient. Other FAQs: slow file traversal, incorrect text file encoding, and binary file reading errors.
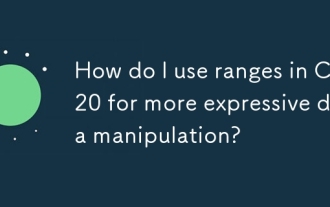
C 20 ranges enhance data manipulation with expressiveness, composability, and efficiency. They simplify complex transformations and integrate into existing codebases for better performance and maintainability.
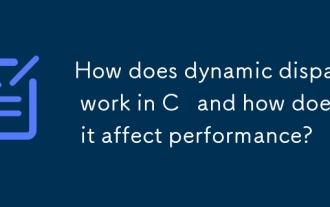
The article discusses dynamic dispatch in C , its performance costs, and optimization strategies. It highlights scenarios where dynamic dispatch impacts performance and compares it with static dispatch, emphasizing trade-offs between performance and
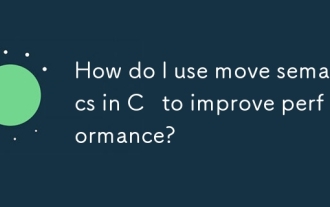
The article discusses using move semantics in C to enhance performance by avoiding unnecessary copying. It covers implementing move constructors and assignment operators, using std::move, and identifies key scenarios and pitfalls for effective appl
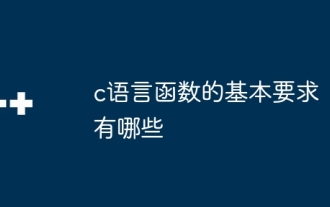
C language functions are the basis for code modularization and program building. They consist of declarations (function headers) and definitions (function bodies). C language uses values to pass parameters by default, but external variables can also be modified using address pass. Functions can have or have no return value, and the return value type must be consistent with the declaration. Function naming should be clear and easy to understand, using camel or underscore nomenclature. Follow the single responsibility principle and keep the function simplicity to improve maintainability and readability.

The article discusses auto type deduction in programming, detailing its benefits like reduced code verbosity and improved maintainability, and its limitations such as potential confusion and debugging challenges.
