


Why does `HttpClient.GetAsync(...)` sometimes deadlock when using `await`/`async` in ASP.NET?
In ASP.NET HttpClient.GetAsync(...)
occasional deadlock occurs when using await
/async
When using the new async
/await
language features and the Tasks API in .NET 4.5, the "awaiting" results of httpClient.GetAsync(...)
may sometimes get stuck. This is due to misuse of the API.
Explanation
In ASP.NET, only one thread can handle a request at a time. Parallel processing can be performed if desired, but additional threads will need to be borrowed from the thread pool, and these threads will have no request context. This is managed by the ASP.NET SynchronizationContext.
By default, when waiting for a Task, methods resume on the captured SynchronizationContext or captured TaskScheduler (if there is no SynchronizationContext). Normally, this is ideal: the async controller action will wait for something, and when it resumes, it resumes using the request context.
Test5Controller.Get
This particular controller method performs AsyncAwait_GetSomeDataAsync (in the context of an ASP.NET request). AsyncAwait_GetSomeDataAsync performs HttpClient.GetAsync (in the context of an ASP.NET request). The HTTP request is sent and HttpClient.GetAsync returns an unfinished Task. Then, AsyncAwait_GetSomeDataAsync waits for the unfinished Task, so it returns an unfinished Task. Now, Test5Controller.Get blocks the current thread until the Task completes.
The HTTP response arrives and the Task returned by HttpClient.GetAsync is completed. AsyncAwait_GetSomeDataAsync then attempts to resume in the ASP.NET request context. However, there is already a thread in this context: the thread blocked in Test5Controller.Get. This can lead to deadlock.
Best Practices
To correct this problem, it is recommended:
- Use
ConfigureAwait(false)
in your "library" async methods whenever possible. In this case AsyncAwait_GetSomeDataAsync will be changed toresult = await httpClient.GetAsync("http://stackoverflow.com", HttpCompletionOption.ResponseHeadersRead).ConfigureAwait(false);
- Avoid blocking Tasks; use
await
instead of "GetResult" (Task.Result
,Task.Wait
should also be replaced withawait
).
By following these best practices, the continuation (the rest of the AsyncAwait_GetSomeDataAsync method) will run on a normal thread pool thread without the need to enter the ASP.NET request context, and the controller itself is asynchronous (will not block the request thread) .
The above is the detailed content of Why does `HttpClient.GetAsync(...)` sometimes deadlock when using `await`/`async` in ASP.NET?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










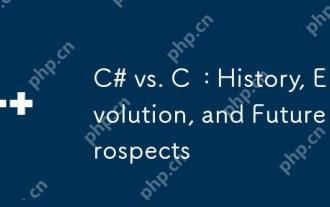
The history and evolution of C# and C are unique, and the future prospects are also different. 1.C was invented by BjarneStroustrup in 1983 to introduce object-oriented programming into the C language. Its evolution process includes multiple standardizations, such as C 11 introducing auto keywords and lambda expressions, C 20 introducing concepts and coroutines, and will focus on performance and system-level programming in the future. 2.C# was released by Microsoft in 2000. Combining the advantages of C and Java, its evolution focuses on simplicity and productivity. For example, C#2.0 introduced generics and C#5.0 introduced asynchronous programming, which will focus on developers' productivity and cloud computing in the future.
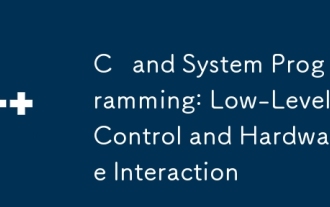
C is suitable for system programming and hardware interaction because it provides control capabilities close to hardware and powerful features of object-oriented programming. 1)C Through low-level features such as pointer, memory management and bit operation, efficient system-level operation can be achieved. 2) Hardware interaction is implemented through device drivers, and C can write these drivers to handle communication with hardware devices.
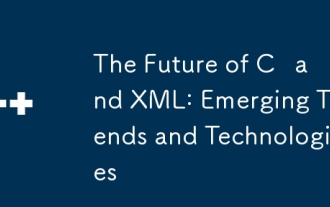
The future development trends of C and XML are: 1) C will introduce new features such as modules, concepts and coroutines through the C 20 and C 23 standards to improve programming efficiency and security; 2) XML will continue to occupy an important position in data exchange and configuration files, but will face the challenges of JSON and YAML, and will develop in a more concise and easy-to-parse direction, such as the improvements of XMLSchema1.1 and XPath3.1.
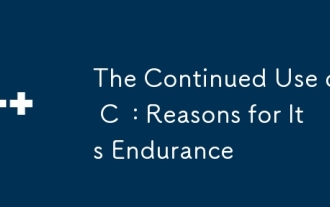
C Reasons for continuous use include its high performance, wide application and evolving characteristics. 1) High-efficiency performance: C performs excellently in system programming and high-performance computing by directly manipulating memory and hardware. 2) Widely used: shine in the fields of game development, embedded systems, etc. 3) Continuous evolution: Since its release in 1983, C has continued to add new features to maintain its competitiveness.
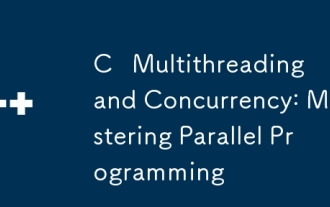
C The core concepts of multithreading and concurrent programming include thread creation and management, synchronization and mutual exclusion, conditional variables, thread pooling, asynchronous programming, common errors and debugging techniques, and performance optimization and best practices. 1) Create threads using the std::thread class. The example shows how to create and wait for the thread to complete. 2) Synchronize and mutual exclusion to use std::mutex and std::lock_guard to protect shared resources and avoid data competition. 3) Condition variables realize communication and synchronization between threads through std::condition_variable. 4) The thread pool example shows how to use the ThreadPool class to process tasks in parallel to improve efficiency. 5) Asynchronous programming uses std::as
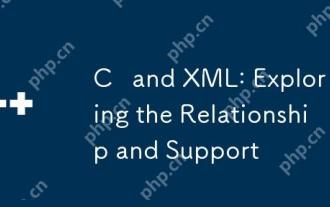
C interacts with XML through third-party libraries (such as TinyXML, Pugixml, Xerces-C). 1) Use the library to parse XML files and convert them into C-processable data structures. 2) When generating XML, convert the C data structure to XML format. 3) In practical applications, XML is often used for configuration files and data exchange to improve development efficiency.
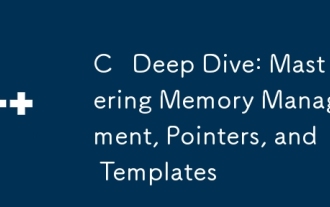
C's memory management, pointers and templates are core features. 1. Memory management manually allocates and releases memory through new and deletes, and pay attention to the difference between heap and stack. 2. Pointers allow direct operation of memory addresses, and use them with caution. Smart pointers can simplify management. 3. Template implements generic programming, improves code reusability and flexibility, and needs to understand type derivation and specialization.
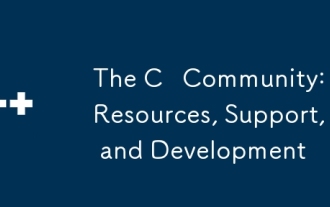
C Learners and developers can get resources and support from StackOverflow, Reddit's r/cpp community, Coursera and edX courses, open source projects on GitHub, professional consulting services, and CppCon. 1. StackOverflow provides answers to technical questions; 2. Reddit's r/cpp community shares the latest news; 3. Coursera and edX provide formal C courses; 4. Open source projects on GitHub such as LLVM and Boost improve skills; 5. Professional consulting services such as JetBrains and Perforce provide technical support; 6. CppCon and other conferences help careers
