How Can Parameterized SQL Statements Prevent SQL Injection Attacks?
Parameterized SQL: A Crucial Defense Against SQL Injection
Database security is paramount, especially when dealing with external inputs from web or desktop applications. Parameterized SQL statements are a cornerstone of robust database interaction, effectively preventing SQL injection attacks.
Consider a vulnerable SQL query:
SELECT empSalary FROM employee WHERE salary = txtSalary.Text
A malicious user could input 0 OR 1=1
, retrieving all salaries. Even more dangerous, input like 0; DROP TABLE employee
could lead to data loss.
Parameterized queries offer a solution. They use placeholders for user-supplied data, isolating the input from the SQL command itself.
Here's how it works in C#:
string sql = "SELECT empSalary FROM employee WHERE salary = @salary"; using (SqlConnection connection = new SqlConnection(/* connection info */)) using (SqlCommand command = new SqlCommand(sql, connection)) { SqlParameter salaryParam = new SqlParameter("salary", SqlDbType.Money); salaryParam.Value = txtMoney.Text; command.Parameters.Add(salaryParam); SqlDataReader results = command.ExecuteReader(); }
And in Visual Basic .NET:
Dim sql As String = "SELECT empSalary FROM employee WHERE salary = @salary" Using connection As New SqlConnection("connectionString") Using command As New SqlCommand(sql, connection) Dim salaryParam = New SqlParameter("salary", SqlDbType.Money) salaryParam.Value = txtMoney.Text command.Parameters.Add(salaryParam) Dim results = command.ExecuteReader() End Using End Using
The key is that the database treats @salary
as a data value, not as executable code. This prevents malicious code from being interpreted as SQL commands. Using parameterized queries significantly strengthens database security, mitigating the risk of data breaches and system compromise.
The above is the detailed content of How Can Parameterized SQL Statements Prevent SQL Injection Attacks?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


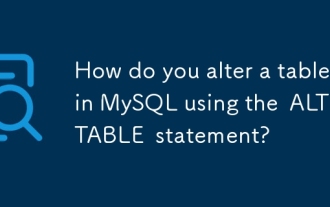
The article discusses using MySQL's ALTER TABLE statement to modify tables, including adding/dropping columns, renaming tables/columns, and changing column data types.
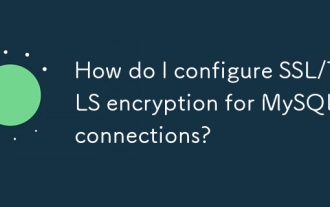
Article discusses configuring SSL/TLS encryption for MySQL, including certificate generation and verification. Main issue is using self-signed certificates' security implications.[Character count: 159]
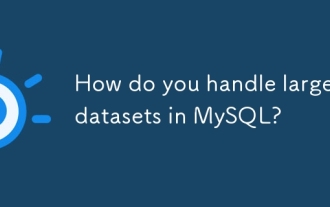
Article discusses strategies for handling large datasets in MySQL, including partitioning, sharding, indexing, and query optimization.
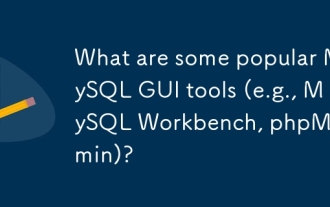
Article discusses popular MySQL GUI tools like MySQL Workbench and phpMyAdmin, comparing their features and suitability for beginners and advanced users.[159 characters]
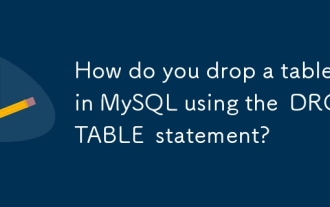
The article discusses dropping tables in MySQL using the DROP TABLE statement, emphasizing precautions and risks. It highlights that the action is irreversible without backups, detailing recovery methods and potential production environment hazards.
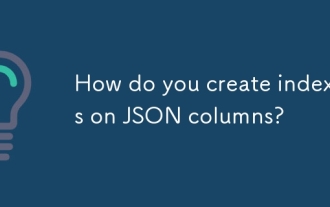
The article discusses creating indexes on JSON columns in various databases like PostgreSQL, MySQL, and MongoDB to enhance query performance. It explains the syntax and benefits of indexing specific JSON paths, and lists supported database systems.
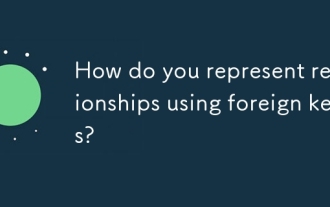
Article discusses using foreign keys to represent relationships in databases, focusing on best practices, data integrity, and common pitfalls to avoid.
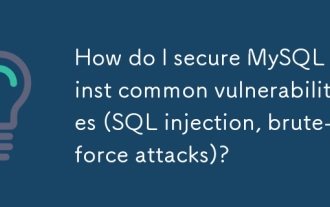
Article discusses securing MySQL against SQL injection and brute-force attacks using prepared statements, input validation, and strong password policies.(159 characters)
