How to Create Hierarchical Recursive Queries in MySQL?
Creating hierarchical recursive queries in MySQL
Understanding Hierarchy
To create hierarchical queries in MySQL, you need to understand how hierarchies are built. Consider the following example table:
id | 名称 | 父ID |
---|---|---|
19 | 类别1 | 0 |
20 | 类别2 | 19 |
21 | 类别3 | 20 |
22 | 类别4 | 21 |
In this table, the parent_id
column represents the ID of the parent category of a given category. For example, Category 2 has a parent_id
of 19, indicating that it is a subcategory of Category 1.
MySQL 8 solution: recursion WITH
For MySQL 8 and above, you can use the recursive WITH syntax:
WITH RECURSIVE cte (id, name, parent_id) AS ( SELECT id, name, parent_id FROM products WHERE parent_id = 19 UNION ALL SELECT p.id, p.name, p.parent_id FROM products p INNER JOIN cte ON p.parent_id = cte.id ) SELECT * FROM cte;
This query will recursively retrieve all child categories for a given parent ID (19 in this example). The output will include all categories that directly or indirectly belong to category 1 (id=19).
MySQL 5.x solution: inline variables or self-join
For older MySQL versions (5.x), there are two alternatives:
Inline variables:
SELECT id, name, parent_id FROM (SELECT * FROM products ORDER BY parent_id, id) products_sorted, (SELECT @pv := '19') initialisation WHERE FIND_IN_SET(parent_id, @pv) AND LENGTH(@pv := CONCAT(@pv, ',', id));
This query takes advantage of MySQL's specific capabilities to allocate and modify variables during its execution. By initializing the @pv
variable with the parent ID (19 in this case) and using the FIND_IN_SET
function to check if parent_id
appears in the descendant list, we can build the list of descendant IDs incrementally.
Self-connection:
SELECT DISTINCT child.id, child.name, child.parent_id FROM products AS child JOIN products AS parent ON child.parent_id = parent.id WHERE parent.id = 19;
This query traverses the hierarchy starting at the specified parent ID (19) using a self-join. By joining the child table with the parent table based on the parent_id
column, we can recursively extract all descendants of a given parent.
The above is the detailed content of How to Create Hierarchical Recursive Queries in MySQL?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


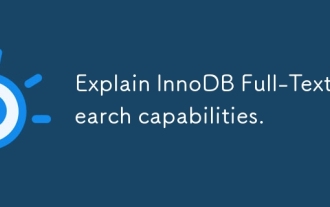
InnoDB's full-text search capabilities are very powerful, which can significantly improve database query efficiency and ability to process large amounts of text data. 1) InnoDB implements full-text search through inverted indexing, supporting basic and advanced search queries. 2) Use MATCH and AGAINST keywords to search, support Boolean mode and phrase search. 3) Optimization methods include using word segmentation technology, periodic rebuilding of indexes and adjusting cache size to improve performance and accuracy.
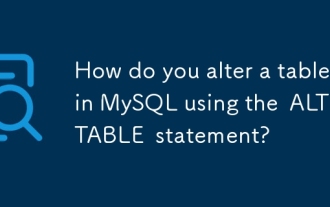
The article discusses using MySQL's ALTER TABLE statement to modify tables, including adding/dropping columns, renaming tables/columns, and changing column data types.
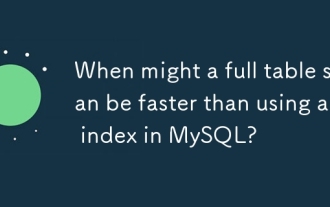
Full table scanning may be faster in MySQL than using indexes. Specific cases include: 1) the data volume is small; 2) when the query returns a large amount of data; 3) when the index column is not highly selective; 4) when the complex query. By analyzing query plans, optimizing indexes, avoiding over-index and regularly maintaining tables, you can make the best choices in practical applications.
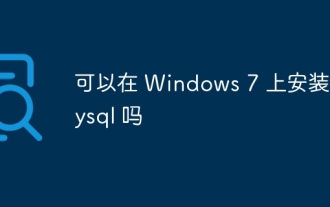
Yes, MySQL can be installed on Windows 7, and although Microsoft has stopped supporting Windows 7, MySQL is still compatible with it. However, the following points should be noted during the installation process: Download the MySQL installer for Windows. Select the appropriate version of MySQL (community or enterprise). Select the appropriate installation directory and character set during the installation process. Set the root user password and keep it properly. Connect to the database for testing. Note the compatibility and security issues on Windows 7, and it is recommended to upgrade to a supported operating system.
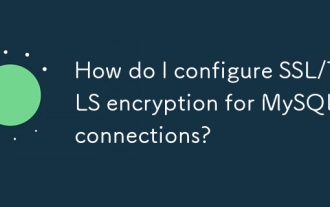
Article discusses configuring SSL/TLS encryption for MySQL, including certificate generation and verification. Main issue is using self-signed certificates' security implications.[Character count: 159]
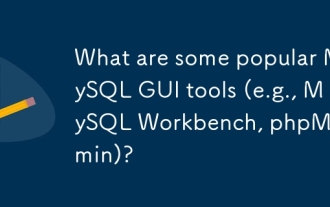
Article discusses popular MySQL GUI tools like MySQL Workbench and phpMyAdmin, comparing their features and suitability for beginners and advanced users.[159 characters]
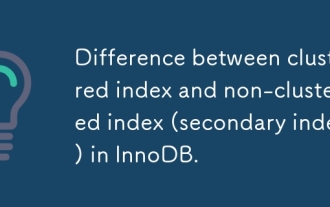
The difference between clustered index and non-clustered index is: 1. Clustered index stores data rows in the index structure, which is suitable for querying by primary key and range. 2. The non-clustered index stores index key values and pointers to data rows, and is suitable for non-primary key column queries.
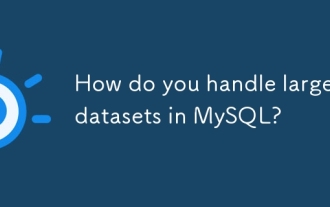
Article discusses strategies for handling large datasets in MySQL, including partitioning, sharding, indexing, and query optimization.
