Task-7
Assignment 7: Inheritance, super
Keyword, and Method Overriding
This assignment demonstrates inheritance, the super
keyword, and method overriding in Java. Let's break down the code and its output.
Grandma Class:
This class represents the base class (grandparent). It has a name
field and a work()
method.
package B15; public class Grandma { String name = "Stella"; // Note: Capitalized for consistency int age = 80; public void work() { System.out.println("Grandma is working."); // More descriptive output } }
Mother Class:
This class inherits from Grandma
. It overrides the work()
method and adds its own name
field. The super
keyword is used to access the Grandma
's name
and work()
method.
package B15; public class Mother extends Grandma { String name = "Arasi"; // Note: Capitalized for consistency int age = 50; public void work() { System.out.println("Mother is working."); // More descriptive output System.out.println("Mother's name = " + name); System.out.println("Grandma's name = " + super.name); System.out.println("Grandma's age = " + super.age); super.work(); } }
Kid Class:
This class inherits from Mother
. It overrides the work()
method and adds a study()
method. The main
method creates a Kid
object and calls its methods.
package B15; public class Kid extends Mother { String name = "Suman"; // Note: Capitalized for consistency int age = 30; public static void main(String[] args) { Kid kid = new Kid(); kid.work(); kid.study(); } public void work() { System.out.println("Kid is working."); // More descriptive output System.out.println("Kid's name = " + name); System.out.println("Mother's name = " + super.name); } public void study() { super.work(); System.out.println("Mother's age = " + super.age); System.out.println("Kid's name = " + name); System.out.println("Kid's age = " + age); System.out.println("Kid is studying."); // More descriptive output } }
Output Explanation:
The output shows the method calls cascading down the inheritance hierarchy. When kid.work()
is called, the Kid
's work()
method is executed first, printing "Kid is working." Then, kid.study()
calls super.work()
, executing the Mother
's work()
method, which in turn calls super.work()
again, executing the Grandma
's work()
method. The super
keyword ensures that the correct method from the parent class is invoked. The output reflects the values of the name
and age
variables at each level of the inheritance hierarchy.
Corrected and Improved Output:
The improved code provides more descriptive output, making it easier to understand the flow of execution. The output will now be:
<code>Kid is working. Kid's name = Suman Mother's name = Arasi Mother is working. Mother's name = Arasi Grandma's name = Stella Grandma's age = 80 Grandma is working. Mother's age = 50 Kid's name = Suman Kid's age = 30 Kid is studying.</code>
The above is the detailed content of Task-7. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


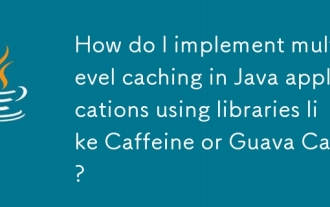
The article discusses implementing multi-level caching in Java using Caffeine and Guava Cache to enhance application performance. It covers setup, integration, and performance benefits, along with configuration and eviction policy management best pra
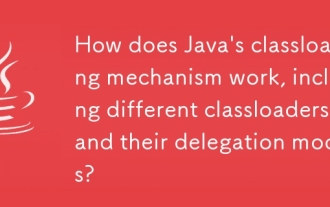
Java's classloading involves loading, linking, and initializing classes using a hierarchical system with Bootstrap, Extension, and Application classloaders. The parent delegation model ensures core classes are loaded first, affecting custom class loa
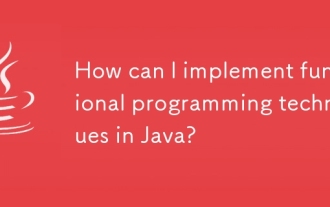
This article explores integrating functional programming into Java using lambda expressions, Streams API, method references, and Optional. It highlights benefits like improved code readability and maintainability through conciseness and immutability
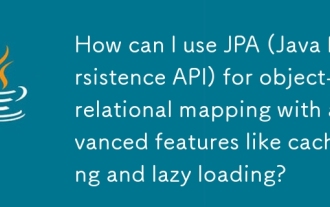
The article discusses using JPA for object-relational mapping with advanced features like caching and lazy loading. It covers setup, entity mapping, and best practices for optimizing performance while highlighting potential pitfalls.[159 characters]
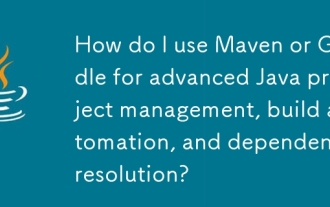
The article discusses using Maven and Gradle for Java project management, build automation, and dependency resolution, comparing their approaches and optimization strategies.
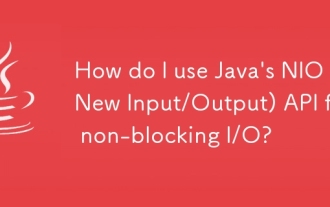
This article explains Java's NIO API for non-blocking I/O, using Selectors and Channels to handle multiple connections efficiently with a single thread. It details the process, benefits (scalability, performance), and potential pitfalls (complexity,
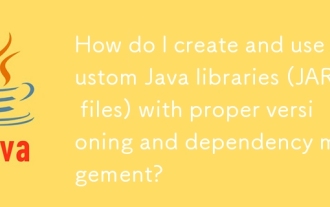
The article discusses creating and using custom Java libraries (JAR files) with proper versioning and dependency management, using tools like Maven and Gradle.
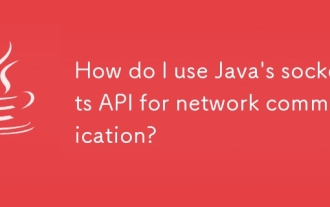
This article details Java's socket API for network communication, covering client-server setup, data handling, and crucial considerations like resource management, error handling, and security. It also explores performance optimization techniques, i
