


Monolithic Code vs. Modularized Code: Choosing the Right Fit for Your AI Project
Happy 2025!
Choosing the Right Code Structure for Your AI Project: Monolithic vs. Modular
The architecture of your codebase significantly impacts the maintainability and efficiency of your AI project, whether frontend or backend. This article compares monolithic and modular code structures, highlighting their advantages and disadvantages, particularly within the context of AI projects using APIs like Azure or Gemini.
Monolithic Code: All in One
A monolithic codebase integrates all components into a single unit. This simplifies smaller projects, offering a quick setup. However, scalability presents challenges.
Advantages of Monolithic Code:
- Simplicity: Easy to set up for smaller projects and teams.
- Reduced Initial Complexity: All components are in one place.
- Simplified Dependency Management: No complex module imports needed.
Disadvantages of Monolithic Code:
- Maintenance Difficulties: Scaling becomes challenging as the project grows.
- Interdependencies: Changes in one area can unintentionally impact others.
- Complex Testing: Debugging becomes harder with increased complexity.
Example (Python):
A simple web server interacting with an AI API might look like this (all logic in one file):
from flask import Flask, jsonify, request import requests app = Flask(__name__) @app.route('/predict', methods=['POST']) def predict(): data = request.json response = requests.post('https://your-ai-api.com/predict', json=data) return jsonify(response.json()) if __name__ == '__main__': app.run(debug=True)
This works for small projects but quickly becomes unwieldy.
Modularized Code: Divide and Conquer
Modularized code breaks down a project into independent modules. This improves organization and maintainability, particularly for larger projects or those involving multiple teams.
Advantages of Modularized Code:
- Clear Organization: Easier to understand and maintain.
- Independent Development: Teams can work on separate modules concurrently.
- Simplified Testing: Modules can be tested individually.
Disadvantages of Modularized Code:
- Complex Setup: Initial configuration is more involved.
- Dependency Management: Managing module interactions requires careful planning.
Example (Python):
The same web server, modularized:
app.py
from flask import Flask from routes.predict_routes import predict_routes app = Flask(__name__) app.register_blueprint(predict_routes) if __name__ == '__main__': app.run(debug=True)
routes/predict_routes.py
from flask import Blueprint, jsonify, request import requests predict_routes = Blueprint('predict_routes', __name__) @predict_routes.route('/predict', methods=['POST']) def predict(): data = request.json response = requests.post('https://your-ai-api.com/predict', json=data) return jsonify(response.json())
This structure is more manageable as the project scales.
Frontend Considerations (JavaScript):
Similar principles apply to JavaScript frontend development. Monolithic JavaScript might use a single file, while modularization uses ES6 modules or frameworks like React.
Choosing the Right Approach for AI Projects:
The best approach depends on:
- Project Scale: Monolithic suits small projects; modular is better for larger ones.
- Team Size: Modularization facilitates parallel work for larger teams.
- Technology Stack: Python and JavaScript readily support modularization.
Conclusion:
Both approaches have their place. Monolithic is suitable for small, simple projects, while modularization excels in larger, more complex AI projects integrating APIs like Azure or Gemini. Choosing the right architecture is crucial for long-term project success.
The above is the detailed content of Monolithic Code vs. Modularized Code: Choosing the Right Fit for Your AI Project. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
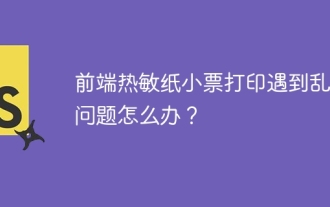
Frequently Asked Questions and Solutions for Front-end Thermal Paper Ticket Printing In Front-end Development, Ticket Printing is a common requirement. However, many developers are implementing...
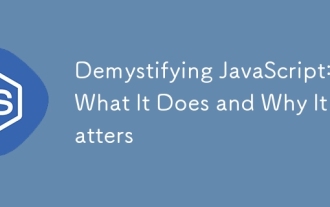
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
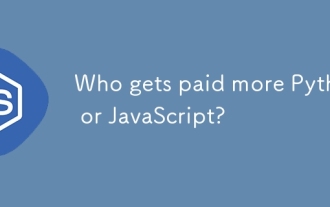
There is no absolute salary for Python and JavaScript developers, depending on skills and industry needs. 1. Python may be paid more in data science and machine learning. 2. JavaScript has great demand in front-end and full-stack development, and its salary is also considerable. 3. Influencing factors include experience, geographical location, company size and specific skills.
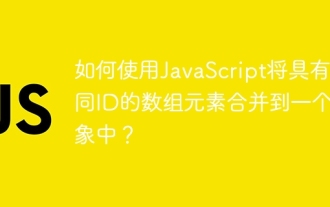
How to merge array elements with the same ID into one object in JavaScript? When processing data, we often encounter the need to have the same ID...
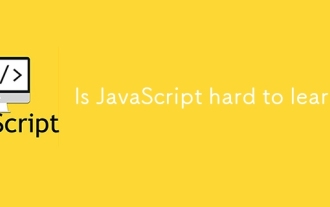
Learning JavaScript is not difficult, but it is challenging. 1) Understand basic concepts such as variables, data types, functions, etc. 2) Master asynchronous programming and implement it through event loops. 3) Use DOM operations and Promise to handle asynchronous requests. 4) Avoid common mistakes and use debugging techniques. 5) Optimize performance and follow best practices.
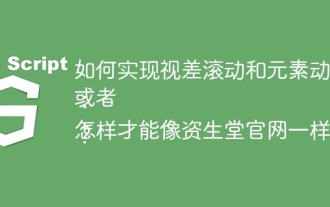
Discussion on the realization of parallax scrolling and element animation effects in this article will explore how to achieve similar to Shiseido official website (https://www.shiseido.co.jp/sb/wonderland/)...
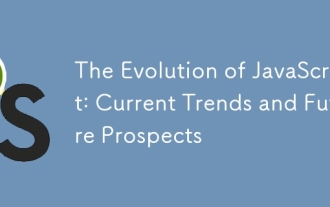
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.
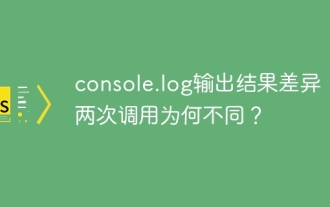
In-depth discussion of the root causes of the difference in console.log output. This article will analyze the differences in the output results of console.log function in a piece of code and explain the reasons behind it. �...
