


How to Fix 'The ObjectContext Instance has been Disposed' Error in Entity Framework?
Jan 26, 2025 am 01:22 AMTroubleshooting Entity Framework's "ObjectContext Instance Disposed" Error
The Problem:
When working with Entity Framework, you might encounter this frustrating error: "The ObjectContext instance has been disposed and can no longer be used for operations that require a connection."
This typically happens when lazy loading is used with a DbContext that's already been closed.
Understanding Lazy vs. Eager Loading:
Entity Framework offers two approaches to loading related data:
- Lazy Loading: Related entities are only fetched from the database when you directly access them. This is the default behavior, but requires the DbContext to remain open.
- Eager Loading: Related entities are loaded along with the initial query. This avoids the "disposed" error.
Why the Error Occurs:
The error arises because the DbContext
is disposed of (usually within a using
statement) before the lazy-loaded navigation properties are accessed. Once disposed, the connection is closed, preventing further database operations.
The Solution: Employ Eager Loading
The most effective solution is to switch to eager loading. Instead of letting Entity Framework load related data on demand, you explicitly include them in your initial query using the Include
method:
IQueryable<memberloan> query = db.MemberLoans.Include(m => m.Membership);
This line ensures that the Membership
property is loaded before the DbContext
is disposed, preventing the exception. Remember to replace memberloan
and Membership
with your actual entity and property names. For multiple related entities, chain multiple Include
calls.
This proactive approach guarantees that all necessary data is retrieved within the scope of the DbContext
, eliminating the "disposed" error and ensuring smooth operation.
The above is the detailed content of How to Fix 'The ObjectContext Instance has been Disposed' Error in Entity Framework?. For more information, please follow other related articles on the PHP Chinese website!

Hot Article

Hot tools Tags

Hot Article

Hot Article Tags

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
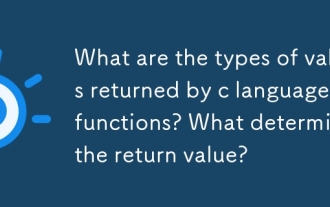
What are the types of values returned by c language functions? What determines the return value?
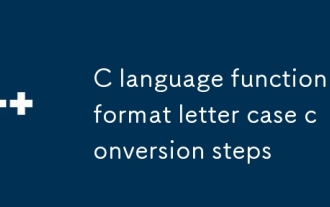
C language function format letter case conversion steps

What are the definitions and calling rules of c language functions and what are the
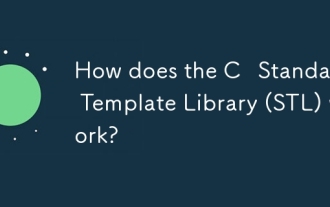
How does the C Standard Template Library (STL) work?

Where is the return value of the c language function stored in memory?
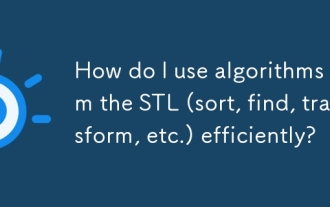
How do I use algorithms from the STL (sort, find, transform, etc.) efficiently?
