How to Build a CRUD App with Golang, Gin, and PostgreSQL
This tutorial shows you how to build a simple CRUD (Create, Read, Update, Delete) application using Golang, the Gin framework, and PostgreSQL. You'll learn to manage data stored in a PostgreSQL database.
Table of Contents
- Introduction
- Prerequisites
- Project Structure
- Project Setup
- Database and Table Creation
- Implementing CRUD Handlers
- API Testing
- Conclusion
1. Introduction
This guide utilizes Gin, a lightweight Golang web framework, to create API endpoints. The application interacts with a PostgreSQL database via the pgx driver. Basic familiarity with Golang and REST APIs is assumed.
2. Prerequisites
Before starting, ensure you have:
- Golang (version 1.20 or later)
- PostgreSQL (any version)
- Postman (or a similar API testing tool)
- A code editor (e.g., VS Code)
3. Project Structure
Organize your project as follows:
<code>crud-app/ ├── main.go ├── config/ │ └── database.go ├── controllers/ │ └── item.go ├── models/ │ └── item.go ├── routes/ │ └── routes.go ├── go.mod └── go.sum </code>
4. Project Setup
-
Create the project directory and initialize a Go module:
mkdir crud-app cd crud-app go mod init github.com/yourusername/crud-app // Replace with your GitHub username
Copy after login -
Install necessary packages:
go get github.com/gin-gonic/gin go get github.com/jackc/pgx/v5
Copy after login
5. Database and Table Creation
-
Create a PostgreSQL database (e.g.,
crud_app
). -
Connect to the database and create the
items
table:CREATE DATABASE crud_app; \c crud_app CREATE TABLE items ( id SERIAL PRIMARY KEY, name TEXT NOT NULL, description TEXT, price NUMERIC(10, 2) );
Copy after login
6. Implementing CRUD Handlers
6.1 Database Connection (config/database.go):
package config import ( "database/sql" "fmt" "log" _ "github.com/jackc/pgx/v5/stdlib" ) var DB *sql.DB func ConnectDatabase() { // ... (Connection string with your credentials) ... }
6.2 Model Definition (models/item.go):
package models type Item struct { ID int `json:"id"` Name string `json:"name"` Description string `json:"description"` Price float64 `json:"price"` }
6.3 CRUD Handlers (controllers/item.go): (Example: Create)
package controllers import ( "crud-app/config" "crud-app/models" "github.com/gin-gonic/gin" "net/http" ) func CreateItem(c *gin.Context) { // ... (Implementation for creating a new item) ... }
Implement similar functions for reading, updating, and deleting items.
6.4 Route Definitions (routes/routes.go):
package routes import ( "crud-app/controllers" "github.com/gin-gonic/gin" ) func SetupRoutes(router *gin.Engine) { router.POST("/items", controllers.CreateItem) // ... (Add routes for other CRUD operations) ... }
6.5 Main Application (main.go):
package main import ( "crud-app/config" "crud-app/routes" "github.com/gin-gonic/gin" ) func main() { config.ConnectDatabase() r := gin.Default() routes.SetupRoutes(r) r.Run(":8080") }
7. API Testing
Run the application (go run main.go
) and test the endpoints using Postman or a similar tool.
8. Conclusion
You've successfully created a basic CRUD application. Remember to fill in the missing CRUD handler implementations and adapt the database connection string to your environment. This foundation can be expanded upon with more advanced features.
The above is the detailed content of How to Build a CRUD App with Golang, Gin, and PostgreSQL. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
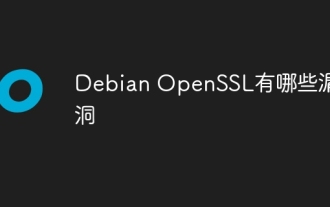
OpenSSL, as an open source library widely used in secure communications, provides encryption algorithms, keys and certificate management functions. However, there are some known security vulnerabilities in its historical version, some of which are extremely harmful. This article will focus on common vulnerabilities and response measures for OpenSSL in Debian systems. DebianOpenSSL known vulnerabilities: OpenSSL has experienced several serious vulnerabilities, such as: Heart Bleeding Vulnerability (CVE-2014-0160): This vulnerability affects OpenSSL 1.0.1 to 1.0.1f and 1.0.2 to 1.0.2 beta versions. An attacker can use this vulnerability to unauthorized read sensitive information on the server, including encryption keys, etc.
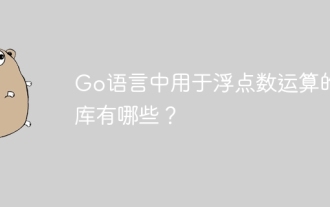
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
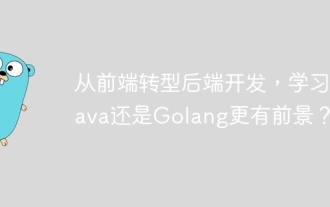
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
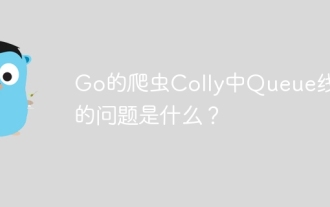
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
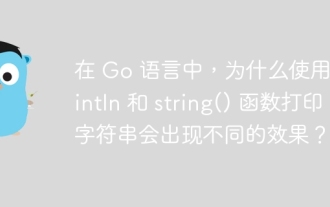
The difference between string printing in Go language: The difference in the effect of using Println and string() functions is in Go...
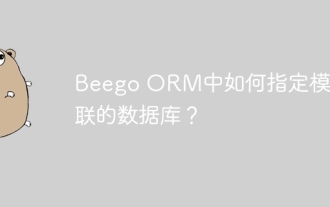
Under the BeegoORM framework, how to specify the database associated with the model? Many Beego projects require multiple databases to be operated simultaneously. When using Beego...
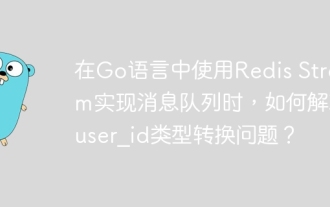
The problem of using RedisStream to implement message queues in Go language is using Go language and Redis...
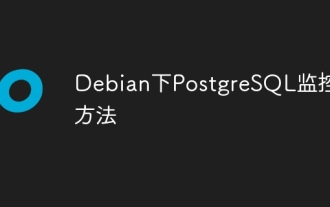
This article introduces a variety of methods and tools to monitor PostgreSQL databases under the Debian system, helping you to fully grasp database performance monitoring. 1. Use PostgreSQL to build-in monitoring view PostgreSQL itself provides multiple views for monitoring database activities: pg_stat_activity: displays database activities in real time, including connections, queries, transactions and other information. pg_stat_replication: Monitors replication status, especially suitable for stream replication clusters. pg_stat_database: Provides database statistics, such as database size, transaction commit/rollback times and other key indicators. 2. Use log analysis tool pgBadg
