Introduction to Linked Lists in PHP: A Beginners Guide
The structure of the linked list node
Each node in the linked list consists of two parts:
Data: The value stored in the node.
- Next: Reference (pointer) to the next node.
- The following is an example of implementing basic nodes in PHP:
Implement a simple linked list
class Node { public $data; public $next; public function __construct($data) { $this->data = $data; $this->next = null; } }
In order to manage the node, we create a LinkedList class that provides a method for the maintenance list and provides the method of operating it.
<本> Basic operation
<.> 1. Add the node to the end
We add the node to the end of the list by reaching the iterative node until the last node reaches the last node.<.> 2. Show the list
We can traverse the list to print all elements.
class LinkedList { private $head; public function __construct() { $this->head = null; } public function append($data) { $newNode = new Node($data); if ($this->head === null) { $this->head = $newNode; } else { $current = $this->head; while ($current->next !== null) { $current = $current->next; } $current->next = $newNode; } } }
<.> 3. Delete nodes
Delete nodes include finding nodes and updating the pointer of the previous node.
public function display() { $current = $this->head; while ($current !== null) { echo $current->data . " -> "; $current = $current->next; } echo "NULL\n"; }
<示> Example usage
The following is a method of implementing the linked list:
public function delete($data) { if ($this->head === null) { return; } if ($this->head->data === $data) { $this->head = $this->head->next; return; } $current = $this->head; while ($current->next !== null && $current->next->data !== $data) { $current = $current->next; } if ($current->next !== null) { $current->next = $current->next->next; } }
<论> Conclusion
$linkedList = new LinkedList(); $linkedList->append(10); $linkedList->append(20); $linkedList->append(30); echo "初始列表:\n"; $linkedList->display(); $linkedList->delete(20); echo "删除 20 后:\n"; $linkedList->display();
The above is the detailed content of Introduction to Linked Lists in PHP: A Beginners Guide. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
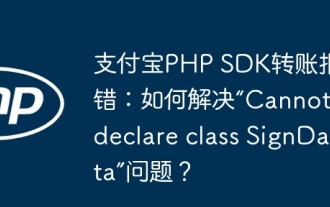
Alipay PHP...
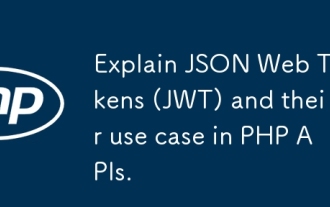
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
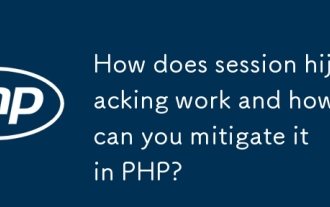
Session hijacking can be achieved through the following steps: 1. Obtain the session ID, 2. Use the session ID, 3. Keep the session active. The methods to prevent session hijacking in PHP include: 1. Use the session_regenerate_id() function to regenerate the session ID, 2. Store session data through the database, 3. Ensure that all session data is transmitted through HTTPS.
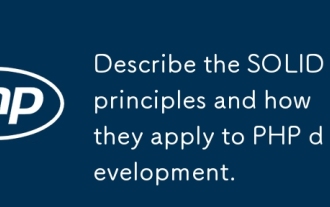
The application of SOLID principle in PHP development includes: 1. Single responsibility principle (SRP): Each class is responsible for only one function. 2. Open and close principle (OCP): Changes are achieved through extension rather than modification. 3. Lisch's Substitution Principle (LSP): Subclasses can replace base classes without affecting program accuracy. 4. Interface isolation principle (ISP): Use fine-grained interfaces to avoid dependencies and unused methods. 5. Dependency inversion principle (DIP): High and low-level modules rely on abstraction and are implemented through dependency injection.
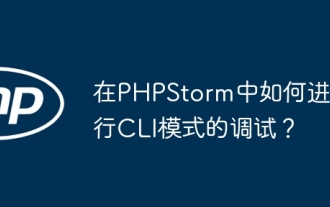
How to debug CLI mode in PHPStorm? When developing with PHPStorm, sometimes we need to debug PHP in command line interface (CLI) mode...
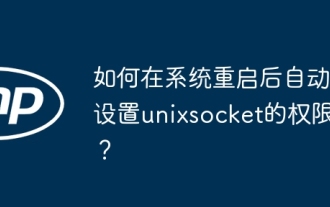
How to automatically set the permissions of unixsocket after the system restarts. Every time the system restarts, we need to execute the following command to modify the permissions of unixsocket: sudo...
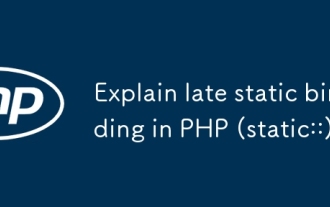
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.
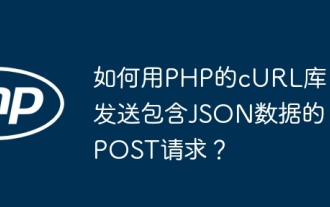
Sending JSON data using PHP's cURL library In PHP development, it is often necessary to interact with external APIs. One of the common ways is to use cURL library to send POST�...
