Rust and Go: The Future of High-Performance Computing
Rust ?: A Deep Dive into Performance and Security
Performance Comparison:
Memory Allocation:
C's manual memory management (illustrated below) is vulnerable to errors. Rust's automatic memory management and bounds checking (also shown below) guarantee memory safety. Rust achieves near-C performance while enhancing safety.
C (Manual Memory Management):
// C: Manual Memory Management (Vulnerable) char* create_string(int size) { char* buffer = malloc(size); // No size checking if (!buffer) return NULL; return buffer; // Caller responsible for free() }
Rust (Safe Memory Allocation):
// Rust: Safe Memory Allocation fn create_string(size: usize) -> Option<Vec<u8>> { // Automatic memory management // Bounds checking // Guaranteed memory safety Some(vec![0; size]) }
Performance Benchmark: Rust leverages zero-cost abstractions and compile-time guarantees to achieve performance comparable to C, but with significantly improved safety.
Memory Management:
C is prone to vulnerabilities like buffer overflows (example below). Rust's compile-time safety prevents such issues (example below).
C (Buffer Overflow Vulnerability):
// Classic Buffer Overflow void vulnerable_copy(char* dest, char* src) { strcpy(dest, src); // No length validation // Potential security exploit }
Rust (Compile-Time Safety):
// Rust prevents buffer overflows fn safe_copy(dest: &mut [u8], src: &[u8]) { // Compile-time bounds checking dest.copy_from_slice(&src[..dest.len()]); }
Security Features:
C's manual memory management increases the risk of buffer overflows, use-after-free vulnerabilities, and memory leaks. Rust's ownership and borrowing system eliminates these issues through compile-time checks, preventing dangling pointers and data races.
Development Effort:
Rust's simplified memory handling (example below) reduces code complexity compared to C's complex pointer management (example below). This translates to fewer lines of code, compile-time error prevention, and less debugging time.
C (Complex Pointer Management):
// C: Complex Pointer Management int* complex_pointer_logic(int* data, int size) { int* result = malloc(size * sizeof(int)); if (!result) return NULL; for (int i = 0; i < size; ++i) { result[i] = data[i] * 2; } return result; }
Rust (Simplified Memory Handling):
// Rust: Simplified Memory Handling fn simplified_logic(data: &[i32]) -> Vec<i32> { // Automatic memory management // No malloc/free required data.iter().map(|&x| x * 2).collect() }
Development Time Metrics: Rust significantly reduces development time due to its concise syntax and compile-time safety checks.
Compilation and Optimization:
Rust's compile-time verification ensures memory and thread safety, resulting in predictable performance and eliminating runtime overhead. Rust generates highly optimized machine code comparable to C.
Go ?: Powering Backend and Cloud Computing
Performance Metrics:
Computation Speed: Go's compiled nature delivers significantly faster execution speeds than interpreted languages like Python (examples below). Benchmarks show Go to be 10-40 times faster for computational tasks.
Python (Slow Computation):
# Python: Slow Computation def fibonacci(n): if n <= 1: return n return fibonacci(n-1) + fibonacci(n-2)
Go (Highly Optimized):
// Go: Highly Optimized func fibonacci(n int) int { if n <= 1 { return n } return fibonacci(n-1) + fibonacci(n-2) }
Benchmark Comparisons: Go's performance advantage stems from its compiled nature and efficient runtime.
Energy Consumption:
Go demonstrates significantly lower energy consumption compared to Python due to its efficient resource management (examples below). Estimates suggest a 60-70% reduction in energy usage.
Python (High Resource Usage):
// C: Manual Memory Management (Vulnerable) char* create_string(int size) { char* buffer = malloc(size); // No size checking if (!buffer) return NULL; return buffer; // Caller responsible for free() }
Go (Efficient Resource Management):
// Rust: Safe Memory Allocation fn create_string(size: usize) -> Option<Vec<u8>> { // Automatic memory management // Bounds checking // Guaranteed memory safety Some(vec![0; size]) }
Energy Metrics: Go's superior computational efficiency translates to considerable energy savings.
Concurrency Model:
Go's native concurrency model contrasts sharply with Python's Global Interpreter Lock (GIL), which limits true parallelism. Go's goroutines and channels enable efficient concurrent programming.
Learning Curve:
Go's statically-typed nature and compiled approach differ from Python's dynamic and interpreted characteristics (examples below). While Go has a steeper initial learning curve, its strong typing and compile-time checks ultimately improve code reliability.
Python (Dynamic, Interpreted):
// Classic Buffer Overflow void vulnerable_copy(char* dest, char* src) { strcpy(dest, src); // No length validation // Potential security exploit }
Go (Static, Compiled):
// Rust prevents buffer overflows fn safe_copy(dest: &mut [u8], src: &[u8]) { // Compile-time bounds checking dest.copy_from_slice(&src[..dest.len()]); }
Community and Ecosystem: Go boasts a growing enterprise adoption rate, a robust cloud-native ecosystem, and increasing job market demand.
Additional Advantages:
Go's single binary deployment, fast compilation times, cross-platform compatibility, comprehensive standard library, and built-in concurrency primitives contribute to its appeal.
Conclusion:
Rust and Go represent a paradigm shift in software development. Rust excels in systems programming by eliminating memory vulnerabilities and delivering C-level performance with enhanced safety. Go transforms backend and cloud computing with its native concurrency, simplified deployment, and speed advantages. Both languages offer superior performance, security, and modern design, making them ideal for future-proof development. They are not just alternatives but replacements for legacy languages, offering lower overhead, reduced complexity, and scalable architectures.
The above is the detailed content of Rust and Go: The Future of High-Performance Computing. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










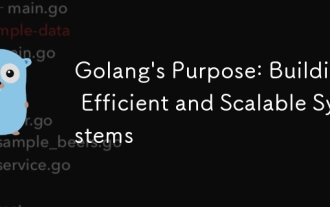
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
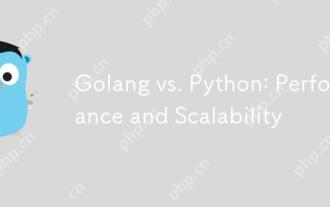
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
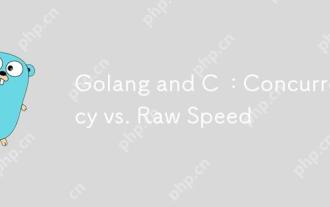
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
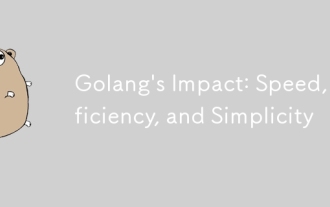
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
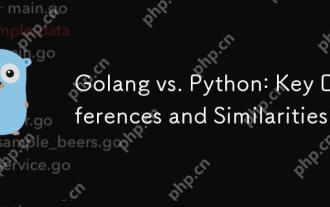
Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
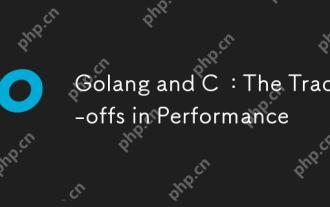
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
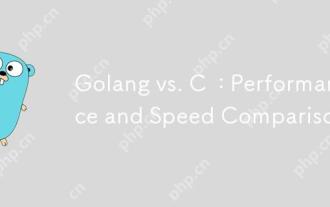
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
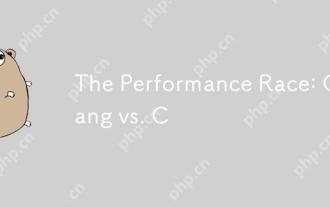
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
