


How Can I Execute Raw SQL Queries with Custom Result Mappings in Entity Framework Core?
Executing Raw SQL Queries and Custom Result Mapping in Entity Framework Core
Overview
Entity Framework Core's evolution has altered how raw SQL queries with custom result mappings are handled. This article addresses the challenges of retrieving data, particularly when combining table data with results from full-text search queries, focusing on solutions for various EF Core versions.
EF Core 8 and Later
EF Core 8 and subsequent versions simplify this process. The SqlQuery
method now directly supports returning arbitrary types. This allows the use of keyless entity types and custom classes to map query results.
EF Core 3.0 and Keyless Entity Types
For EF Core 3.0, keyless entity types provide a clean solution. Define a class without a primary key using the [Keyless]
attribute or by calling HasNoKey()
.
[Keyless] public class SomeModel { ... } protected override void OnModelCreating(ModelBuilder modelBuilder) { modelBuilder.Entity<SomeModel>().HasNoKey(); }
Execute your query using FromSqlRaw
or FromSql
:
var result = context.SomeModels.FromSqlRaw("YOUR SQL SCRIPT").ToList();
EF Core 2.1 and Query Types
EF Core 2.1 introduced query types, offering a structured approach to mapping custom classes. Add a DbQuery<T>
property to your DbContext
and use FromSql
:
public DbQuery<SomeModel> SomeModels { get; set; } var result = context.SomeModels.FromSql("YOUR SQL SCRIPT").ToList();
Summary
These methods effectively address the need for custom result mapping when executing raw SQL queries in Entity Framework Core, enabling flexible data retrieval, including scenarios involving full-text search results and combined data sets. Choose the approach that best suits your EF Core version. Remember to replace "YOUR SQL SCRIPT"
with your actual SQL query.
The above is the detailed content of How Can I Execute Raw SQL Queries with Custom Result Mappings in Entity Framework Core?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


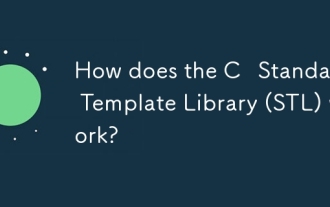
This article explains the C Standard Template Library (STL), focusing on its core components: containers, iterators, algorithms, and functors. It details how these interact to enable generic programming, improving code efficiency and readability t
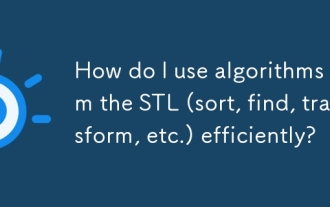
This article details efficient STL algorithm usage in C . It emphasizes data structure choice (vectors vs. lists), algorithm complexity analysis (e.g., std::sort vs. std::partial_sort), iterator usage, and parallel execution. Common pitfalls like
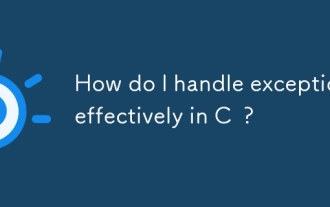
This article details effective exception handling in C , covering try, catch, and throw mechanics. It emphasizes best practices like RAII, avoiding unnecessary catch blocks, and logging exceptions for robust code. The article also addresses perf
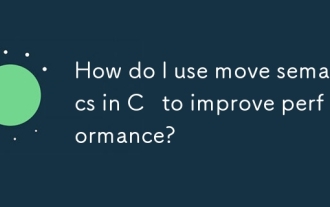
The article discusses using move semantics in C to enhance performance by avoiding unnecessary copying. It covers implementing move constructors and assignment operators, using std::move, and identifies key scenarios and pitfalls for effective appl
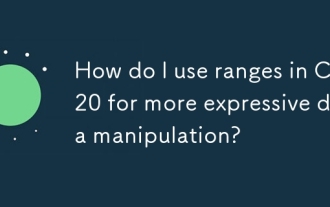
C 20 ranges enhance data manipulation with expressiveness, composability, and efficiency. They simplify complex transformations and integrate into existing codebases for better performance and maintainability.
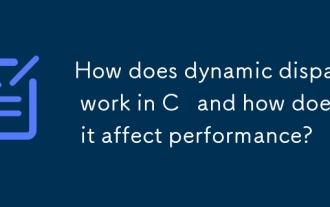
The article discusses dynamic dispatch in C , its performance costs, and optimization strategies. It highlights scenarios where dynamic dispatch impacts performance and compares it with static dispatch, emphasizing trade-offs between performance and
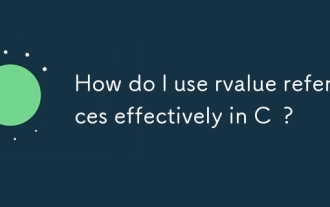
Article discusses effective use of rvalue references in C for move semantics, perfect forwarding, and resource management, highlighting best practices and performance improvements.(159 characters)
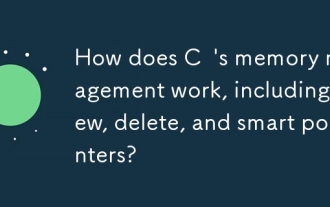
C memory management uses new, delete, and smart pointers. The article discusses manual vs. automated management and how smart pointers prevent memory leaks.
