


From Zero to Wow: Building a Beginner-Friendly Angular GPT-Powered App
Section 1: Introduction ?️
What This Article is About
Welcome to the exciting journey of building your very first Angular application! This article is designed specifically for beginners who want to dive into the world of web development. Together, we’ll build a simple yet powerful chat application powered by OpenAI’s GPT API. Along the way, you’ll discover how Angular, a popular front-end framework, enables developers to create scalable, modern web applications.
Whether you’re entirely new to coding or just starting with Angular, this article will guide you every step of the way, ensuring no prior experience is required.
Why Angular?
Angular is one of the most versatile frameworks for building interactive and dynamic web applications. Here’s why we’ve chosen it for this project:
- Beginner-Friendly: Angular provides a structured and consistent development experience, making it an excellent choice for those just starting out.
- TypeScript Integration: It leverages TypeScript, a strongly typed superset of JavaScript, to enhance productivity and catch errors during development.
- Powerful Ecosystem: With features like built-in dependency injection, reusable components, and an active community, Angular equips you to build professional-grade applications.
What You’ll Achieve
By the end of this article, you will have:
- A Fully Functional GPT-Powered App: A simple, interactive chat application where users can ask questions and receive responses from OpenAI’s GPT model.
- Angular Knowledge: A foundational understanding of how Angular components, services, and configurations come together to create a web application.
- API Integration Experience: Learn how to connect Angular applications to external APIs, like OpenAI’s GPT, through HTTP requests.
What Makes This Article Special
Unlike many tutorials, this guide not only walks you through creating an app but also provides clear explanations for every step, helping you grasp the underlying concepts of Angular. By the end, you’ll not only have a working app but also the confidence to build your own projects.
? Ready to start coding? In the next section, we’ll explore the basic tools and setup required to build this app.
? Full Code Repository: You can find the complete source code for this tutorial here.
Section 2: Getting Ready to Learn Angular: Becoming an Angular developer
Before you start building your Angular GPT-powered app, it’s important to prepare your environment and familiarize yourself with the tools and concepts you’ll be using. This section guides you step-by-step to ensure a smooth setup and learning experience.
What You Need to Learn First
To build an Angular app, there are a few foundational skills you need to acquire:
1. HTML and CSS
-
What They Are:
- HTML (HyperText Markup Language) defines the structure of your webpage.
- CSS (Cascading Style Sheets) is used to style the content.
- Why They’re Important: Angular components rely on HTML templates and CSS styles to define their structure and appearance.
-
Resources:
- HTML and CSS for Beginners: Article
- HTML Crash course: Video
2. TypeScript
- What It Is: TypeScript is a strongly typed superset of JavaScript, used by Angular to enhance code quality and maintainability.
- Why It’s Important: Angular uses TypeScript for features like type checking, interfaces, and decorators.
-
Resource:
- TypeScript Official Docs
- Typescript Crash Course: Video
3. Node.js
What It Is:
Node.js is a JavaScript runtime that Angular uses to manage dependencies and run development tools.How to Install:
- Download the installer for your OS from Node.js Official Website.
- Follow the instructions to complete the installation.
- Verify the installation:
node -v npm -v
4. Git Basics
- What It Is: Git is a version control system that allows you to track changes and collaborate effectively.
-
Key Steps:
- Install Git: Git Installation Guide.
- Initialize a repository:
git init
-
Resource:
- Git Basics Guide
Setting Up Angular CLI
The Angular CLI (Command Line Interface) is a powerful tool that helps you scaffold and manage Angular projects effortlessly.
- Install the Angular CLI globally using npm:
npm install -g @angular/cli
- Verify the installation:
ng version
-
Resource:
- Angular CLI Documentation
Getting Your OpenAI API Key
You’ll need an API key to connect your Angular app to OpenAI’s GPT API.
-
Create an OpenAI Account:
- Sign up at OpenAI.
-
Generate Your API Key:
- Navigate to the API Keys section.
- Click Create New Key and copy the generated key.
⚠️ Important: Keep your API key private to avoid unauthorized access.
Familiarizing Yourself with Angular
Take time to understand Angular’s structure and ecosystem. Here are some helpful resources:
-
Official Tutorials:
- The Angular team provides beginner-friendly guides to help you get started.
- Angular Tutorials
-
Angular Documentation:
- Use the official documentation as a reference throughout your journey.
- Angular Documentation
-
Communities to Join:
- Angular Reddit
- Stack Overflow - Angular Questions
- Angular Discord Community: Engage with developers to ask questions and share knowledge.
? You’re Ready!
With the environment set up and foundational knowledge in place, you’re prepared to start planning your GPT-powered app.
Next up: Section 3: Planning the GPT-Powered Chat App.
Section 3: Planning the GPT-Powered Chat App
Before diving into coding, it’s essential to have a clear plan. In this section, you’ll understand the app’s purpose, the technologies involved, and how the app will function.
Overview of the App
The goal is to build a GPT-powered chat interface that allows users to send a prompt and receive responses from OpenAI’s GPT API. The app will focus on simplicity and usability while introducing key Angular concepts.
Key Features:
- A user-friendly chat interface.
- Integration with OpenAI’s GPT API to handle prompts and generate responses.
- A responsive design for better usability.
Technologies You’ll Use
To bring this app to life, you’ll rely on the following technologies:
-
Angular:
- Angular serves as the frontend framework for building a dynamic and scalable user interface.
- You’ll leverage Angular features such as components, services, and dependency injection.
-
OpenAI’s GPT API:
- The GPT API will power the chatbot’s responses.
- You’ll use the Chat Completions API to send and receive user prompts.
How the App Works
Here’s a step-by-step breakdown of how your app will function:
-
User Input:
- The user types a prompt into the chat input field.
-
API Request:
- The input is sent to OpenAI’s GPT API using Angular’s HTTP client service.
-
Response Handling:
- The GPT API generates a response based on the prompt and sends it back.
-
Display Response:
- The response is displayed in the chat interface for the user to see.
Preparing for Development
API Key Reminder:
Before you proceed, ensure you have:
- Registered for an OpenAI account.
- Generated and securely saved your API key. You’ll need it to configure the GPT service in your app.
? Next Steps: Now that you have a plan, it’s time to set up your Angular project in Section 4: Setting Up the Angular Project.
Fantastic! Ready to move forward with Section 4: Setting Up the Angular Project. Let’s get it done! ??️
Section 4: Setting Up the Angular Project
With your environment ready and a clear plan in place, it’s time to create the foundation of your GPT-powered Angular app. In this section, you’ll set up the Angular project, explore its structure, and ensure everything runs correctly.
Install Angular CLI
If you haven’t already installed the Angular CLI, here’s a quick recap:
- Open your terminal and run the following command to install the CLI globally:
node -v npm -v
- Verify the installation:
git init
Create and Explore Your Project
-
Generate a New Project:
- Use the Angular CLI to scaffold a new project:
npm install -g @angular/cli
-
Navigate to Your Project Directory:
- Once the project is created, move into the project folder:
ng version
Project Structure Overview
Angular generates a default project structure. Here are the key folders and files:
-
src/app:
- The main folder where your app’s code lives.
- You’ll create components, services, and modules here.
-
angular.json:
- The configuration file for your Angular app.
-
package.json:
- Lists all dependencies and scripts for your project.
-
node_modules:
- Contains all installed dependencies for the app.
Understanding this structure will help you navigate your project as you develop.
First Run: Test Your Setup
- Start the Angular development server:
node -v npm -v
- Open your browser and navigate to:
git init
- You should see the default Angular app running. If the page loads successfully, your setup is complete!
? Next Steps: With the foundation in place, it’s time to start building the core features of your GPT-powered chat app in Section 5: Building the Chat App.
Section 5: Building the GPT Powered Angular Chat App
Now that your Angular project is set up, it’s time to build the core feature: the GPT-powered chat app. In this section, you’ll create a chat component, implement the GPT service, and connect them together for a fully functional experience.
Create the Chat Component
Angular components are the building blocks of your application. Follow these steps to create a standalone chat component:
-
Generate the Component:
- Use the Angular CLI to generate a standalone chat component:
npm install -g @angular/cli
-
What Happens:
- This command creates the following files in src/app/components/chat/:
- chat.component.ts (logic and structure)
- chat.component.html (HTML template)
- chat.component.css (styles)
- This command creates the following files in src/app/components/chat/:
Update the Chat Component Template
To create the chat interface, update chat.component.html:
ng version
Explanation:
- Dependency Injection: The GptService is injected into the component through the constructor.
- Two-Way Binding: The userInput variable is bound to the
- API Integration: The sendPrompt() function calls the GPT service, handles responses, and updates the response variable.
Create the GPT Service
Services in Angular manage data and logic that can be reused across components. Follow these steps to create and configure the GPT service:
-
Generate the Service:
- Run the following command:
npm install -g @angular/cli
-
Update the GPT Service Logic:
- Open src/app/services/gpt.service.ts and update it as follows:
ng version
Explanation:
- HttpClient: Used to send HTTP requests to OpenAI’s GPT API.
- generateResponse(): Sends the user prompt to the API and returns an observable with the response.
- API Key: Replace 'your-api-key-here' with your actual OpenAI API key.
Style the Chat Component
Add basic styling to chat.component.css:
node -v npm -v
Explanation:
- The chat-container centers the chat interface.
- The textarea and button styles ensure usability and aesthetics.
Securing Your API Key with Environment Variables
Hardcoding sensitive data like API keys in your service files is risky. A better approach is to use environment variables to keep your keys secure. This section will guide you on how to set up and use environment files in your Angular project.
Step 1: Create Environment Files
- Navigate to the src/ directory in your project.
- Create a new folder named environments if it doesn’t already exist.
- Inside the environments/ folder, create two files:
- environment.ts: For development settings.
- environment.prod.ts: For production settings.
Step 2: Add Your API Key
- Open the environment.ts file and define your API key:
git init
- Open the environment.prod.ts file and add the same key for production:
npm install -g @angular/cli
Step 3: Update the GPT Service
Modify the gpt.service.ts file to use the environment variable instead of a hardcoded API key:
ng version
Step 4: Prevent Sensitive Data from Being Committed
- Open the .gitignore file in your project’s root directory.
- Add the following line to ignore your environment files:
npm install -g @angular/cli
This ensures your API keys and sensitive data won’t be included in version control.
Step 5: Share Environment Setup Instructions
When working on a team or sharing the project, provide clear instructions (like this section) on how to create and configure environment files. Avoid sharing actual API keys.
? Why Use Environment Variables?
This approach keeps your sensitive information secure while allowing different configurations for development and production environments. It’s a best practice for modern web development.
? Next Steps: Now that the chat component and GPT service are ready, you’ll integrate the component into your app in Section 6: Testing Your Application.
Section 6: Testing Your Application
Now that your GPT-powered chat app is built, it’s time to test it and ensure everything works as expected. This section will guide you through running your app locally, testing its features, and troubleshooting common issues.
Run the App Locally
- Start the development server:
node -v npm -v
- Open your browser and navigate to:
git init
- What You Should See:
- The default Angular app should load with your custom GPT-powered chat component at the center.
- If you can interact with the chat interface and get responses from the API, congratulations! Your app is working.
Interact with the Chat Interface
- Enter a message or question into the chat input field.
- Click the Send button.
- Wait for the GPT API to respond. The reply should display in the response area below the input.
Troubleshooting Tips
If something isn’t working as expected, use the following checklist to debug your app:
Common Issues and Solutions
-
The Page Doesn't Load:
- Check the terminal output for errors when running ng serve.
- Ensure all dependencies are installed:
npm install -g @angular/cli
-
No Response from GPT API:
- Verify your API key in the environment.ts file.
- Check for typos in your GPT service URL (https://api.openai.com/v1/chat/completions).
- Ensure you’ve included the necessary headers (Authorization and Content-Type).
-
CORS Issues:
- Ensure your browser isn’t blocking API requests due to Cross-Origin Resource Sharing (CORS).
- If needed, use a browser extension or configure your backend to allow CORS.
-
Error in the Console:
- Look for error messages in the browser developer console.
- If the error relates to the API, double-check your service configuration.
-
Styling Issues:
- Ensure the styles in chat.component.css are applied correctly.
- Use browser developer tools to inspect the DOM and debug CSS.
? Pro Tip: Regularly check the terminal and browser console for any warnings or errors. These often provide useful hints for troubleshooting.
? Next Steps: Once your app is tested and functional, you can enhance it further in Section 7: Taking the Next Steps.
Section 7: Taking the Next Steps
Congratulations! You’ve successfully built and tested a GPT-powered chat app using Angular. Now, let’s explore Angular-specific enhancements and techniques to elevate your app and expand your skillset.
Enhancing Your App
-
Loading Indicators:
- Improve user experience by adding a loading spinner or message while waiting for a response.
- Use Angular’s *ngIf directive to display a spinner conditionally during HTTP calls.
-
Styling with Angular Material:
- Enhance your app’s appearance with Angular Material components.
- Install Angular Material:
node -v npm -v
- Add prebuilt components like buttons, input fields, and dialog boxes for a polished look.
- ?️ Resource: Angular Material Documentation.
-
Form Validation:
- Implement validation for user inputs to ensure prompts meet criteria (e.g., non-empty, character limits).
- Use Angular’s FormBuilder and reactive forms to manage form state and validation logic.
git init
-
Reusability with Shared Components:
- Extract reusable UI elements, such as buttons or input fields, into shared components.
- Use these components across multiple parts of your app to maintain consistency.
-
Routing:
- Add multiple pages to your app, such as a settings page for customization or a help page for user guidance.
- Use Angular Router to set up and navigate between these pages.
- ?️ Resource: Angular Routing Guide.
-
State Management:
- Manage app-wide state, such as chat history or user preferences, using Angular services or state management libraries like NgRx.
- ?️ Resource: Introduction to NgRx.
Connecting to Other Endpoints
Learning how to work with APIs is a crucial skill in Angular development. Practice by integrating additional endpoints into your app:
-
REST API Integration:
- Experiment with connecting to public APIs, such as weather or news APIs.
- Use Angular’s HttpClient to fetch data and display it dynamically in your app.
- ?️ Resource: Angular HTTPClient Guide.
-
CRUD Operations:
- Build a simple feature to Create, Read, Update, and Delete data using a RESTful API.
- Practice building forms for input, lists for data display, and edit/delete functionality.
-
Error Handling:
- Learn to handle API errors gracefully using Angular’s catchError operator in rxjs.
- Display user-friendly error messages for better usability.
Share Your Work
-
Deploy Your App:
- Host your app using platforms like Firebase, Vercel, or Netlify.
- ?️ Resource: Angular Deployment Guide.
-
Collaborate with Others:
- Share your code on GitHub and invite feedback or contributions from the Angular community.
- Include a README file with setup instructions and a project overview.
? Next Steps:
Keep refining your app, experimenting with Angular’s features, and building more projects to deepen your understanding. Angular offers powerful tools to build dynamic and scalable web applications—mastering them is your next milestone!
Section 8: Conclusion
You’ve come a long way! From setting up your development environment to building and testing your GPT-powered chat app, you’ve successfully taken your first steps into Angular development. Along the way, you’ve learned critical skills like creating components, managing services, and integrating APIs.
What You’ve Accomplished
- Built a Functional Angular App: You created a GPT-powered chat interface using Angular's powerful framework and tools.
- Learned Angular Fundamentals: From components to dependency injection, you’ve explored Angular’s core features.
- Connected to External APIs: You integrated a third-party API and handled HTTP requests and responses effectively.
- Followed Best Practices: By using environment variables and secure development techniques, you’ve adopted professional coding standards.
Call to Action
Your journey into Angular development has only just begun. Here’s how you can keep progressing:
-
Practice Regularly:
- Build more projects to solidify your understanding of Angular concepts.
- Experiment with features like routing, state management, and animations.
-
Explore Advanced Angular Topics:
- Learn about lazy loading, optimizing performance, and testing Angular applications.
- ?️ Resource:
- Angular Advanced Topics.
- Angular & AI
-
Join the Community:
- Engage with other Angular developers for inspiration and support.
- Contribute to open-source Angular projects to sharpen your skills.
-
Stay Updated:
- Angular is a constantly evolving framework. Follow the official Angular blog and community channels to stay informed about new features and best practices.
Encouragement
Starting something new can be daunting, but remember: every expert was once a beginner. The effort you’ve put into learning Angular will pay off as you continue to build and grow. With the resources and knowledge you now have, you’re well-equipped to tackle more ambitious projects.
? Keep Building: The world of web development is vast and full of opportunities. Angular is just the beginning—dive deeper, experiment, and make your ideas come to life. Please feel free to ask questions if any or make contributions, you are highly welcomed!
? Explore the Code: The full source code for this project is available on GitHub. Feel free to clone, modify, or contribute to the project!
The above is the detailed content of From Zero to Wow: Building a Beginner-Friendly Angular GPT-Powered App. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
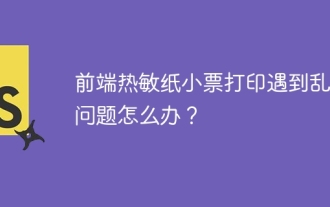
Frequently Asked Questions and Solutions for Front-end Thermal Paper Ticket Printing In Front-end Development, Ticket Printing is a common requirement. However, many developers are implementing...
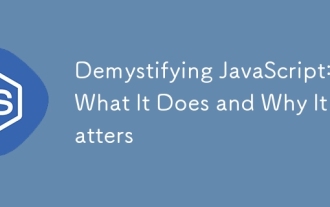
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
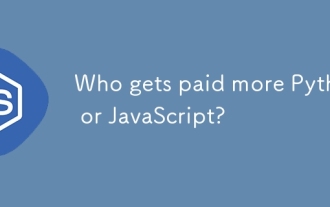
There is no absolute salary for Python and JavaScript developers, depending on skills and industry needs. 1. Python may be paid more in data science and machine learning. 2. JavaScript has great demand in front-end and full-stack development, and its salary is also considerable. 3. Influencing factors include experience, geographical location, company size and specific skills.
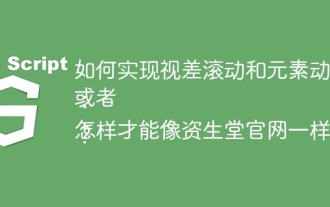
Discussion on the realization of parallax scrolling and element animation effects in this article will explore how to achieve similar to Shiseido official website (https://www.shiseido.co.jp/sb/wonderland/)...
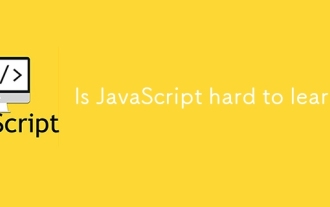
Learning JavaScript is not difficult, but it is challenging. 1) Understand basic concepts such as variables, data types, functions, etc. 2) Master asynchronous programming and implement it through event loops. 3) Use DOM operations and Promise to handle asynchronous requests. 4) Avoid common mistakes and use debugging techniques. 5) Optimize performance and follow best practices.
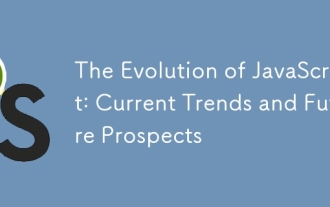
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.
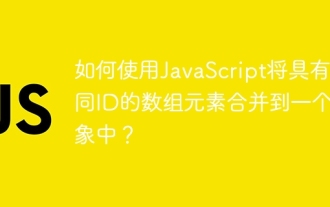
How to merge array elements with the same ID into one object in JavaScript? When processing data, we often encounter the need to have the same ID...
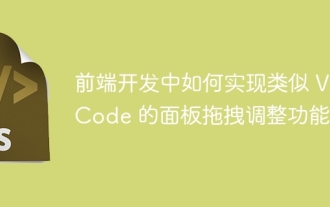
Explore the implementation of panel drag and drop adjustment function similar to VSCode in the front-end. In front-end development, how to implement VSCode similar to VSCode...
