Unraveling the '===' of javascript
This guide delves into the intricacies of JavaScript's strict equality operator (===
), as defined within the ECMAScript specification's "Strict Equality Comparison" section. Let's explore its functionality step-by-step:
The ===
Algorithm: A Detailed Look
The ===
operator employs the following algorithm to determine equality:
-
Type Check: The operator first compares the types of the two values. If the types differ, it immediately returns
false
. Type matching proceeds to the next step only if types are identical. -
Type-Specific Comparison:
-
Numbers:
-
NaN === NaN
evaluates tofalse
(a crucial point of divergence). - Numerically identical values return
true
. -
0
and-0
are considered equal (true
).
-
-
Strings: Character-by-character comparison determines equality. Identical sequences yield
true
; otherwise,false
. -
Booleans:
true === true
andfalse === false
both returntrue
. Otherwise,false
. -
Objects (Arrays and Functions Included):
===
checks for reference equality. Only if both values point to the same memory location (the same object) will it returntrue
. -
null
andundefined
:null === null
andundefined === undefined
returntrue
. However,null === undefined
isfalse
due to type differences.
-
Why NaN === NaN
is false
This is a frequent source of confusion. The specification defines NaN
(Not-a-Number) as unequal to itself. This is because NaN
represents an invalid or undefined numerical result; comparing two undefined results as equal lacks logical coherence.
Example:
NaN === NaN; // false
To reliably check for NaN
, utilize Number.isNaN()
or Object.is()
:
Number.isNaN(NaN); // true Object.is(NaN, NaN); // true
Why 0 === -0
is true
The specification treats 0
and -0
as equivalent because, in most mathematical operations, their behavior is indistinguishable. However, subtle differences exist in specific scenarios (e.g., 1 / 0
yields Infinity
, while 1 / -0
results in -Infinity
). For situations requiring differentiation, use Object.is()
:
NaN === NaN; // false
Objects and Reference Equality (Revisited)
When comparing objects, ===
assesses reference equality. Two objects with identical contents are not considered equal unless they are the same object in memory:
Number.isNaN(NaN); // true Object.is(NaN, NaN); // true
But:
Object.is(+0, -0); // false
Further Exploration of JavaScript Fundamentals
The above is the detailed content of Unraveling the '===' of javascript. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










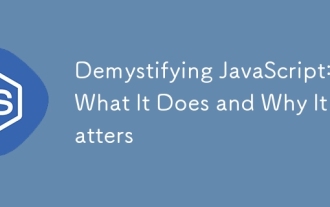
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
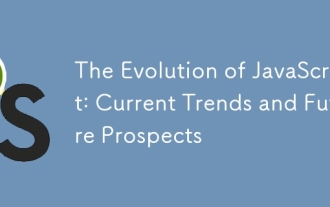
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.
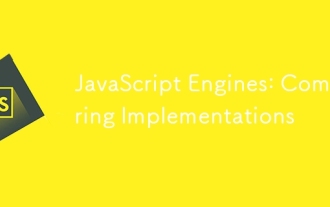
Different JavaScript engines have different effects when parsing and executing JavaScript code, because the implementation principles and optimization strategies of each engine differ. 1. Lexical analysis: convert source code into lexical unit. 2. Grammar analysis: Generate an abstract syntax tree. 3. Optimization and compilation: Generate machine code through the JIT compiler. 4. Execute: Run the machine code. V8 engine optimizes through instant compilation and hidden class, SpiderMonkey uses a type inference system, resulting in different performance performance on the same code.
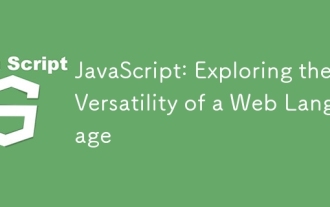
JavaScript is the core language of modern web development and is widely used for its diversity and flexibility. 1) Front-end development: build dynamic web pages and single-page applications through DOM operations and modern frameworks (such as React, Vue.js, Angular). 2) Server-side development: Node.js uses a non-blocking I/O model to handle high concurrency and real-time applications. 3) Mobile and desktop application development: cross-platform development is realized through ReactNative and Electron to improve development efficiency.
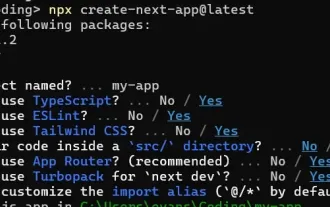
This article demonstrates frontend integration with a backend secured by Permit, building a functional EdTech SaaS application using Next.js. The frontend fetches user permissions to control UI visibility and ensures API requests adhere to role-base
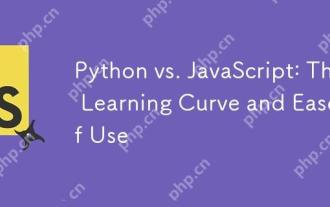
Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
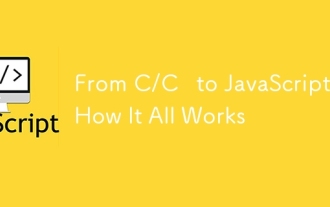
The shift from C/C to JavaScript requires adapting to dynamic typing, garbage collection and asynchronous programming. 1) C/C is a statically typed language that requires manual memory management, while JavaScript is dynamically typed and garbage collection is automatically processed. 2) C/C needs to be compiled into machine code, while JavaScript is an interpreted language. 3) JavaScript introduces concepts such as closures, prototype chains and Promise, which enhances flexibility and asynchronous programming capabilities.
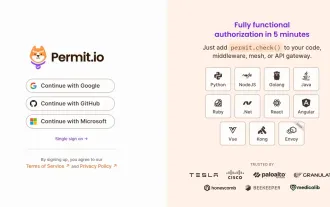
I built a functional multi-tenant SaaS application (an EdTech app) with your everyday tech tool and you can do the same. First, what’s a multi-tenant SaaS application? Multi-tenant SaaS applications let you serve multiple customers from a sing
